How to implement video quality detection using Golang and FFmpeg
Method of using Golang and FFmpeg to implement video quality detection
Abstract: This article is based on the Golang programming language and FFmpeg multimedia processing tools, and introduces a method using Golang How to implement video quality detection with FFmpeg. The article first briefly introduces the basic knowledge of Golang and FFmpeg, then explains the principles and common indicators of video quality detection, and provides specific code examples for readers' reference.
Keywords: Golang, FFmpeg, video quality detection, code examples
1. Introduction
Nowadays, video applications are widely used in various fields, and video quality detection has become an important task. On the premise of ensuring image quality, the size of the video file needs to be reduced as much as possible. In order to achieve this goal, we can use the Golang programming language and FFmpeg multimedia processing tools to detect and optimize video quality.
2. Basic knowledge of Golang and FFmpeg
2.1 Introduction to Golang
Golang is an open source programming language developed by Google. It has high concurrency performance and concise syntax, and is suitable for developing network applications and distributed systems. Golang also has a powerful standard library and rich third-party libraries for easy development.
2.2 Introduction to FFmpeg
FFmpeg is a set of open source audio and video encoding and decoding tools that can support a variety of multimedia file formats. By using FFmpeg, we can perform operations such as encoding and decoding, format conversion, and editing of videos, which has a wide range of application fields.
3. Principle of video quality detection
Video quality detection mainly evaluates video quality by analyzing and comparing video frames and calculating image quality indicators. The following are some commonly used video quality indicators:
3.1 Root mean square error (RMSE)
RMSE is a common indicator of video quality, used to evaluate the difference between original video frames and reconstructed video frames. difference between. The calculation formula is as follows:
RMSE = sqrt(1/n * sum((Frame1 - Frame2)^2))
Among them, Frame1 is the original video frame, Frame2 is the reconstructed video frame, and n is the video frame number.
3.2 Structural Similarity (SSIM)
SSIM is a structured quality measurement method used to evaluate the degree of distortion of an image or video. The SSIM value range is between 0 and 1. The closer to 1, the better the image quality. The calculation formula is as follows:
SSIM = (2 mu1 mu2 c1) (2 sigma12 c2) / ((mu1^2 mu2^2 c1) * (sigma1^2 sigma2^ 2 c2))
Among them, mu1 and mu2 represent the average of the original video frame and the reconstructed video frame, sigma1 and sigma2 represent the standard deviation of the original video frame and the reconstructed video frame, and sigma12 represents the original video frame and the reconstructed video The covariance of the frame, c1 and c2 are constants.
4. Use Golang and FFmpeg to implement video quality detection
In Golang, you can implement the video quality detection function by calling FFmpeg related commands. The following is a sample code for calculating RMSE and SSIM metrics for a given video file:
package main import ( "fmt" "os/exec" "strings" ) func main() { // 输入视频文件路径 videoFile := "test.mp4" // 使用FFmpeg获取视频信息 cmd1 := exec.Command("ffmpeg", "-i", videoFile) info, err := cmd1.CombinedOutput() if err != nil { fmt.Println("获取视频信息失败:", err) return } // 解析FFmpeg输出的视频信息 lines := strings.Split(string(info), " ") var frameRate float64 for _, line := range lines { if strings.Contains(line, "Stream #") && strings.Contains(line, "Video") { parts := strings.Fields(line) for i := 0; i < len(parts); i++ { if parts[i] == "fps," { fmt.Sscanf(parts[i-1], "%f", &frameRate) } } } } // 计算视频帧数 cmd2 := exec.Command("ffprobe", "-v", "error", "-select_streams", "v:0", "-show_entries", "stream=nb_frames", "-of", "default=nokey=1:noprint_wrappers=1", videoFile) output, err := cmd2.CombinedOutput() if err != nil { fmt.Println("获取视频帧数失败:", err) return } frameCount := strings.TrimSpace(string(output)) fmt.Println("视频帧数:", frameCount) // 计算RMSE cmd3 := exec.Command("ffplay", "-i", videoFile, "-vf", "extractplanes=y", "-f", "null", "-") output, err = cmd3.CombinedOutput() if err != nil { fmt.Println("计算RMSE失败:", err) return } rmse := strings.TrimSpace(string(output)) fmt.Println("RMSE:", rmse) // 计算SSIM cmd4 := exec.Command("ffmpeg", "-i", videoFile, "-vf", "ssim", "-f", "null", "-") output, err = cmd4.CombinedOutput() if err != nil { fmt.Println("计算SSIM失败:", err) return } ssim := strings.TrimSpace(string(output)) fmt.Println("SSIM:", ssim) }
It should be noted that in order to run the above code, Golang and FFmpeg need to be installed first and added to the system. in environment variables.
5. Summary
This article introduces a method of using Golang and FFmpeg to implement video quality detection, and provides specific code examples. By calling FFmpeg related commands, we can obtain the frame rate and number of frames of the video and calculate the RMSE and SSIM indicators of the video. Readers can further optimize and expand according to their needs to achieve more complex video quality detection functions.
References:
- FFmpeg official website: https://www.ffmpeg.org/
- Golang official website: https://golang.org/
Copyright statement: This article is automatically generated by the assistant. If there is any infringement, please contact us in time to delete it.
The above is the detailed content of How to implement video quality detection using Golang and FFmpeg. For more information, please follow other related articles on the PHP Chinese website!
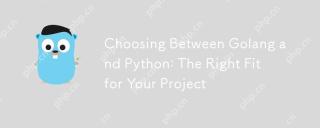
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
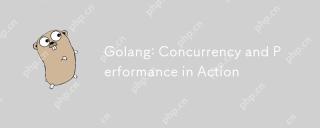
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
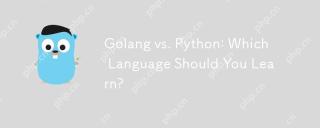
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
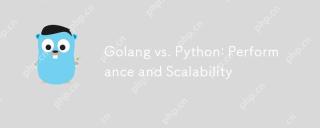
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
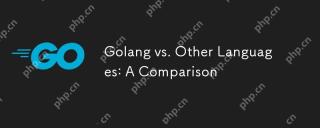
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
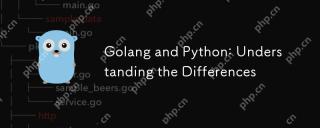
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
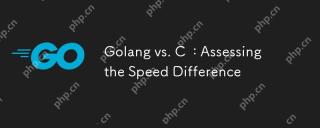
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
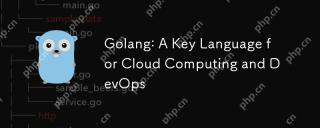
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Chinese version
Chinese version, very easy to use
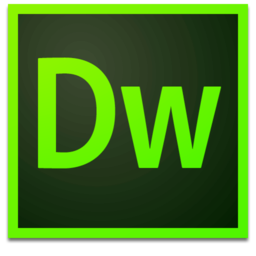
Dreamweaver Mac version
Visual web development tools
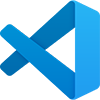
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft