How to use PHP to develop a simple shopping cart function
Introduction: With the rapid development of e-commerce, the shopping cart function is essential in online shopping malls part. This article will introduce how to use PHP language to develop a simple shopping cart function, help readers understand the basic principles of shopping carts, and provide specific code examples so that readers can better understand and practice.
1. Basic principles of shopping cart
Before starting to develop the shopping cart function, we need to understand the basic principles of shopping cart. The shopping cart is actually a container that stores information about the products purchased by the user. It can be used to record the products and their quantities that the user has purchased, and provide operations such as adding, deleting, and modifying products. Shopping carts usually use sessions to store product information purchased by users to ensure that users can access and modify their shopping carts throughout the shopping process.
2. Create a shopping cart page
First, we need to create a shopping cart page to display the list of products that the user has purchased and the corresponding action buttons. The shopping cart page is usually implemented with a form.
<table> <tr> <th>商品名称</th> <th>商品价格</th> <th>商品数量</th> <th>操作</th> </tr> <?php // 获取购物车中的商品信息,并进行遍历输出 foreach($_SESSION['cart'] as $product){ echo "<tr>"; echo "<td>".$product['name']."</td>"; echo "<td>".$product['price']."</td>"; echo "<td>".$product['quantity']."</td>"; echo "<td><a href='remove.php?id=".$product['id']."'>删除</a></td>"; echo "</tr>"; } ?> </table>
3. Add products to the shopping cart
When the user clicks the "Add to Shopping Cart" button, we need to add the purchased product information to the shopping cart. To achieve this functionality, we can add an "Add to Cart" button for each item in the product list and pass the product's unique ID using a URL parameter.
<!-- 商品列表页面 --> <?php // 获取商品列表信息,并进行遍历输出 foreach($products as $product){ echo "<p>".$product['name']." - ".$product['price']."</p>"; echo "<a href='add_to_cart.php?id=".$product['id']."'>添加到购物车</a>"; echo "<hr>"; } ?> <!-- add_to_cart.php --> <?php session_start(); $productId = $_GET['id']; // 根据商品ID查询商品信息 $product = getProductById($productId); // 判断购物车中是否已存在该商品 if(isset($_SESSION['cart'][$productId])){ $_SESSION['cart'][$productId]['quantity']++; }else{ $_SESSION['cart'][$productId] = [ 'id' => $product['id'], 'name' => $product['name'], 'price' => $product['price'], 'quantity' => 1 ]; } // 重定向到购物车页面 header("Location: cart.php"); ?>
4. Delete products from the shopping cart
When the user clicks the "Delete" link, we need to delete the corresponding product from the shopping cart. To achieve this functionality, we can add a "Delete" link for each item in the action column of the shopping cart page and pass the item's unique ID using a URL parameter.
<!-- cart.php --> <table> <tr> <th>商品名称</th> <th>商品价格</th> <th>商品数量</th> <th>操作</th> </tr> <?php // 获取购物车中的商品信息,并进行遍历输出 foreach($_SESSION['cart'] as $productId => $product){ echo "<tr>"; echo "<td>".$product['name']."</td>"; echo "<td>".$product['price']."</td>"; echo "<td>".$product['quantity']."</td>"; echo "<td><a href='remove.php?id=".$productId."'>删除</a></td>"; echo "</tr>"; } ?> </table> <!-- remove.php --> <?php session_start(); $productId = $_GET['id']; // 从购物车中删除指定ID的商品 unset($_SESSION['cart'][$productId]); // 重定向到购物车页面 header("Location: cart.php"); ?>
Summary: Through the above code examples, we have learned how to use PHP language to develop a simple shopping cart function. The shopping cart function is a typical application scenario that uses sessions to store user data, and it is also very common in actual development. I hope this article can help readers better understand and master the basic principles of shopping carts, and be able to flexibly apply them in actual projects.
The above is the detailed content of How to develop a simple shopping cart function using PHP. For more information, please follow other related articles on the PHP Chinese website!
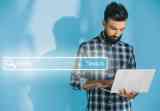
Long URLs, often cluttered with keywords and tracking parameters, can deter visitors. A URL shortening script offers a solution, creating concise links ideal for social media and other platforms. These scripts are valuable for individual websites a
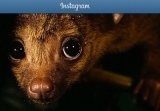
Following its high-profile acquisition by Facebook in 2012, Instagram adopted two sets of APIs for third-party use. These are the Instagram Graph API and the Instagram Basic Display API.As a developer building an app that requires information from a
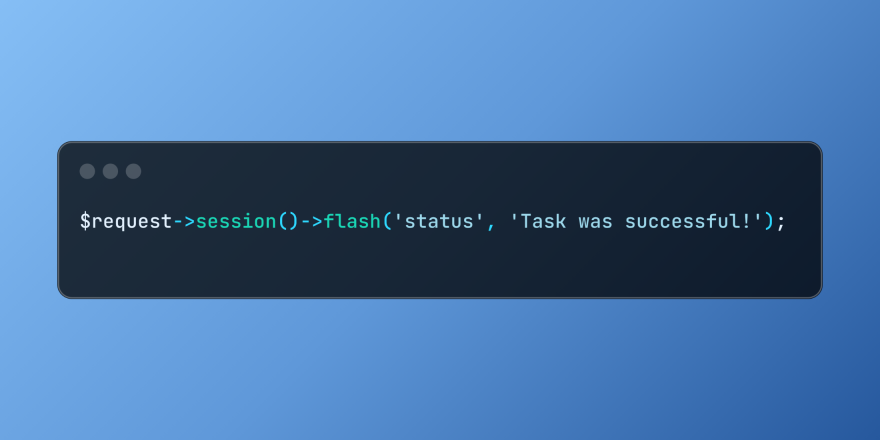
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
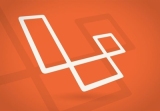
This is the second and final part of the series on building a React application with a Laravel back-end. In the first part of the series, we created a RESTful API using Laravel for a basic product-listing application. In this tutorial, we will be dev
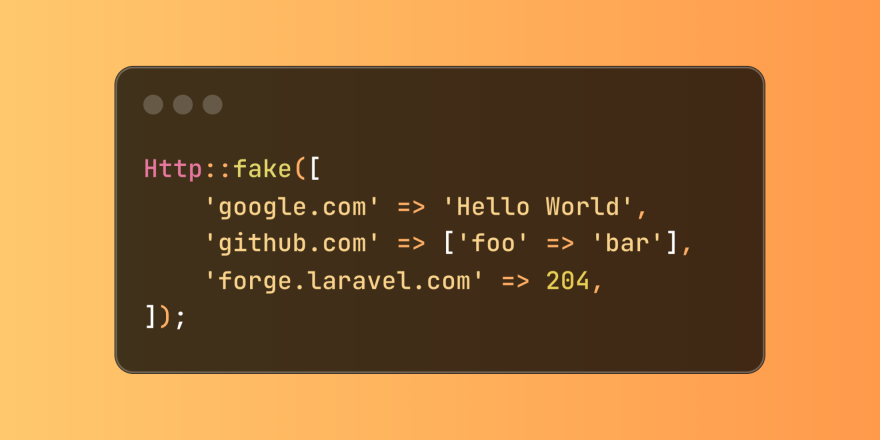
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
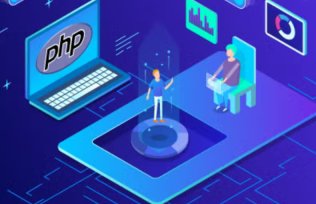
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
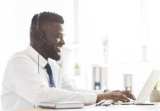
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
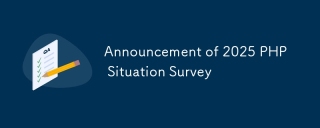
The 2025 PHP Landscape Survey investigates current PHP development trends. It explores framework usage, deployment methods, and challenges, aiming to provide insights for developers and businesses. The survey anticipates growth in modern PHP versio


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
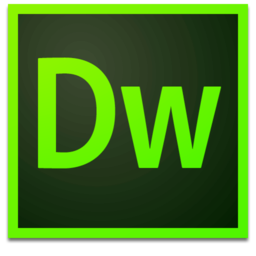
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Zend Studio 13.0.1
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!
