


The powerful ORM of the Django framework and the lightweight database access of Flask
Introduction:
In web development, the database is an indispensable part. Database access and operations are critical to the performance and reliability of a web application. Django and Flask are two popular Python web frameworks that provide different ways of accessing databases. This article will introduce the powerful ORM (Object Relational Mapping) in the Django framework and the lightweight database access method in the Flask framework, and provide specific code examples.
- Powerful ORM of Django framework
Django is a full-featured web application framework, of which ORM is an important part of it. ORM is a programming technology that allows developers to operate the database in an object-oriented manner by mapping tables in the database to Python objects. Django's ORM provides rich functions and convenient syntax, which can perform complex query, insert, update and delete operations on the database.
The following is a simple Django ORM example, showing how to create a model class, insert data and query data through ORM:
from django.db import models class User(models.Model): name = models.CharField(max_length=50) age = models.IntegerField() # 插入数据 user1 = User(name='Alice', age=25) user1.save() user2 = User(name='Bob', age=30) user2.save() # 查询数据 users = User.objects.all() for user in users: print(user.name, user.age)
In the above code, we first define a User model class, which maps to the "user" table in the database. Then, we created two User objects and saved them to the database. Finally, through the User.objects.all() method, we obtain all User objects in the database and print their names and ages.
Django's ORM also provides a wealth of query methods, such as filter(), exclude() and annotate(), etc., which can filter data according to conditions, exclude specified data, and perform aggregation operations. In addition, ORM also supports advanced functions such as transaction management, data migration and model association, which is very suitable for complex database operation requirements.
- Flask's lightweight database access
Compared with Django, Flask is a more lightweight web framework that does not have a built-in ORM. However, Flask provides the extension package "Flask-SQLAlchemy", which is a Flask integrated version of SQLAlchemy and provides ORM support for Flask applications.
SQLAlchemy is a Python SQL toolkit that allows developers to use SQL language for database operations in Python by providing an efficient and flexible database access interface. Flask-SQLAlchemy integrates SQLAlchemy and provides convenient database access.
The following is a simple Flask-SQLAlchemy example that shows how to use ORM for database operations:
from flask import Flask from flask_sqlalchemy import SQLAlchemy app = Flask(__name__) app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///users.db' db = SQLAlchemy(app) class User(db.Model): id = db.Column(db.Integer, primary_key=True) name = db.Column(db.String(50)) age = db.Column(db.Integer) # 插入数据 user1 = User(name='Alice', age=25) db.session.add(user1) db.session.commit() user2 = User(name='Bob', age=30) db.session.add(user2) db.session.commit() # 查询数据 users = User.query.all() for user in users: print(user.name, user.age)
In the above code, first we create a Flask application and configure the database connection . Then a User model class is defined, and the table and field types are specified by extending the syntax of Flask-SQLAlchemy. Next, we created two User objects and added them to the database through the db.session.add()
and db.session.commit()
methods, and finally User.query.all()
Obtains all User objects in the database and prints their names and ages.
In addition to basic database operations, Flask-SQLAlchemy also supports complex queries, transaction management, data migration, model association and other functions, which can meet most common database needs.
Summary:
The Django framework provides rich database access functions through its powerful ORM, which is suitable for complex database operations and large-scale projects. The Flask framework provides a lightweight database access method through Flask-SQLAlchemy, which is suitable for small projects or scenarios with low database access requirements. Based on actual needs, we can choose the appropriate framework and database access method, and use the functions and syntax it provides to develop efficient and reliable Web applications.
Reference:
- Django documentation. [Online]. Available: https://docs.djangoproject.com/
- Flask documentation. [Online]. Available: https://flask.palletsprojects.com/
- SQLAlchemy documentation. [Online]. Available: https://www.sqlalchemy.org/
- Flask-SQLAlchemy documentation. [Online] ]. Available: https://flask-sqlalchemy.palletsprojects.com/
The above is the detailed content of Powerful ORM of Django framework and lightweight database access of Flask. For more information, please follow other related articles on the PHP Chinese website!
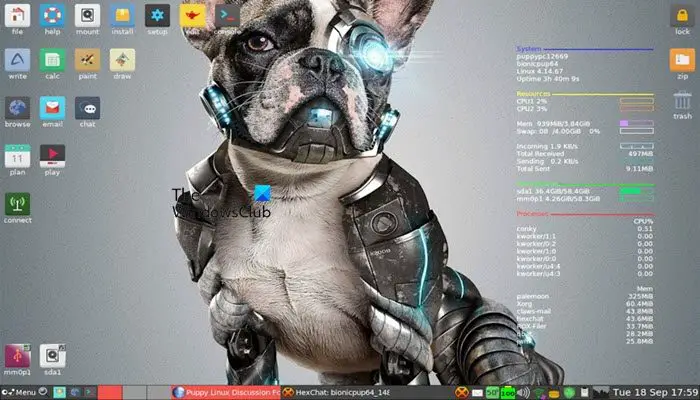
正在寻找完美的Linux发行版,为旧的或低端计算机注入新的活力吗?如果是的话,那么你来对地方了。在本文中,我们将探索一些轻量级Linux发行版的首选,这些发行版是专门为较旧或功能较弱的硬件量身定做的。无论这样做背后的动机是重振老旧的设备,还是只是在预算内最大化性能,这些轻量级选项肯定能满足需求。为什么要选择轻量级的Linux发行版?选择轻量级Linux发行版有几个优点,第一个优点是在最少的系统资源上获得最佳性能,这使得它们非常适合处理能力、RAM和存储空间有限的旧硬件。除此之外,与较重的资源密集
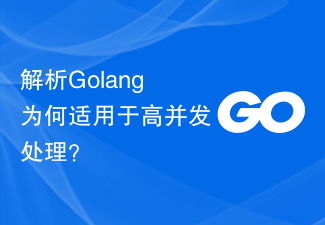
Golang(Go语言)是一种由Google开发的编程语言,旨在提供高效、简洁、并发和轻量级的编程体验。它内置了并发特性,为开发者提供了强大的工具,使其在处理高并发情况下表现优异。本文将深入探讨Golang为何适用于高并发处理的原因,并提供具体的代码示例加以说明。Golang并发模型Golang采用了基于goroutine和channel的并发模型。goro
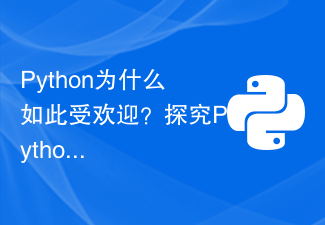
Python为什么如此受欢迎?探究Python在编程领域的优势,需要具体代码示例Python作为一种高级编程语言,自问世之日起便备受程序员们的喜爱与推崇。究其原因,不仅仅是因为它的简洁、易读和强大的功能,更因为它在各个领域都展现出了无可比拟的优势。本文将探究Python在编程领域的优势,并通过具体的代码示例来解释为什么Python如此受欢迎。首先,Pytho
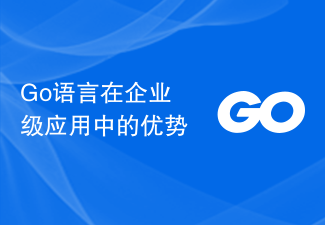
思考使用Go语言进行企业级应用开发是越来越受到关注的原因之一是其出色的性能和并发特性。本文将探讨Go语言在企业级应用开发中的优势,并给出具体的代码示例来说明这些优势。一、并发编程能力Go语言通过goroutine的机制实现高效的并发编程,这使得它在处理并发任务时具有明显的优势。利用goroutine,我们可以轻松地创建并发任务,并通过通道(channel)进
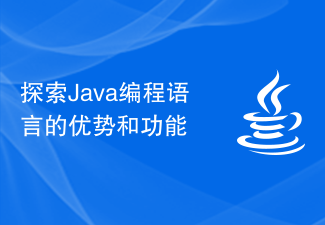
Java是一种广泛使用的计算机编程语言,由SunMicrosystems公司于1995年推出。它是一种高级的、面向对象的、可移植的编程语言,被设计用于开发跨平台的应用程序。Java编程语言具有许多优点,使其在软件开发领域中广泛受欢迎。首先,Java是一种面向对象的编程语言。面向对象编程是一种计算机编程的范式,它将程序中的数据和操作封装在对象中,通过对象之间
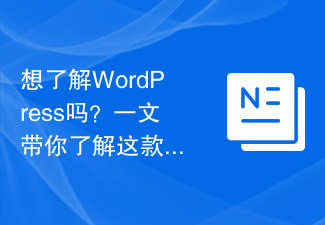
WordPress是当今世界上最受欢迎的网站构建和内容管理系统之一。它的灵活性和可定制性使其成为许多网站所有者和开发者的首选工具。无论是个人博客、企业网站,还是电子商务平台,WordPress都能提供强大的功能和可扩展性。本文将深入讨论WordPress的特点、优势以及如何使用它来建设自己的网站。首先,让我们来了解一下WordPress的起源和发展历程。Wo
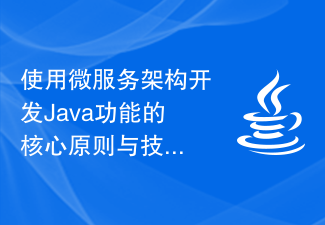
使用微服务架构开发Java功能的核心原则与技巧随着云计算和大数据的迅速发展,传统的单体应用已经不再适应复杂的业务需求。微服务架构(MicroservicesArchitecture)应运而生,成为了构建可扩展、灵活和可维护的应用程序的新兴范式。在这篇文章中,我们将探讨使用微服务架构开发Java功能的核心原则与技巧,并给出具体的代码示例。单一职责原则(Sin
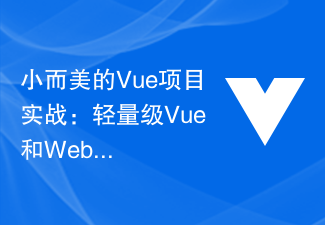
Vue框架的兴起改变了前端开发的方式,其简单易用、高效灵活的特点受到了广大开发者的认可。而Webpack作为一款强大的模块打包工具,也在前端工程化的发展中扮演了重要角色。本文将会介绍一个小而美的Vue项目实战,使用轻量级的Vue.js和Webpack进行开发,能够快速上手,为初学者提供学习参考。前置知识在学习本文前,我们需要具备以下知识储备:•基础的HTM


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
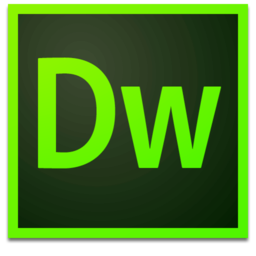
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
