


Explore memory optimization technology and garbage collector management in Go language
Exploring memory optimization technology and garbage collector management in Go language
Introduction:
Go language has a powerful memory management and garbage collection mechanism, providing Many tools and techniques to optimize an application's memory usage. In this article, we will explore some memory optimization techniques in the Go language and show how to use the garbage collector for memory management. We will introduce in detail the memory allocation, memory pool, pointer and garbage collector technologies in the Go language, and give corresponding code examples.
- Memory allocation
In the Go language, use the "new" keyword to create a new object and return its pointer. This object allocates a space in memory and is initialized to zero. The following is a simple example:
type Person struct { Name string Age int } func main() { p := new(Person) p.Name = "Alice" p.Age = 25 }
Go language also provides the "make" function for creating reference type data structures such as slices, maps, and channels. The "make" function allocates a contiguous space in memory and returns a reference. The following is an example of creating a slice:
slice := make([]int, 0, 10)
- Memory Pool
In the Go language, we can use the memory pool to reuse allocated memory blocks and avoid frequent memory allocation and release operations. . The memory pool can be implemented through the sync.Pool type. The following is a simple example:
import "sync" type ByteSlice struct { buf []byte } var pool = sync.Pool{ New: func() interface{} { return &ByteSlice{make([]byte, 0, 1024)} }, } func GetByteSlice() *ByteSlice { return pool.Get().(*ByteSlice) } func PutByteSlice(bs *ByteSlice) { bs.buf = bs.buf[:0] pool.Put(bs) }
In the above example, a memory pool is created through sync.Pool. Every time a ByteSlice object is obtained from the memory pool, the New function is called to create it. a new object. After using the ByteSlice object, you can use the Put function to put the object back into the memory pool and reuse it.
- Pointer
In Go language, we can use pointers to operate memory. Pointers can avoid copying large data into functions, improving performance. The following is a simple pointer example:
type Person struct { Name string Age int } func updateAge(p *Person) { p.Age = 30 } func main() { p := &Person{ Name: "Bob", Age: 25, } updateAge(p) }
In the above example, the Age property of the object pointed to by the p pointer can be directly modified by passing the pointer as a parameter to the updateAge function.
- Garbage Collector
The garbage collector of Go language is an important feature of automatic memory management. The garbage collector periodically checks for blocks of memory in your program that are no longer in use and frees them. The garbage collector uses a three-color marking algorithm for memory recycling, ensuring memory security and efficiency.
In the Go language, you can manually trigger the execution of the garbage collector through the functions in the runtime package. The following is an example:
import ( "fmt" "runtime" ) func main() { fmt.Println("Before GC:", runtime.NumGoroutine()) runtime.GC() fmt.Println("After GC:", runtime.NumGoroutine()) }
In the above example, the runtime.GC function is used to manually trigger the execution of the garbage collector, and the current number of Goroutines is obtained through the runtime.NumGoroutine function.
Conclusion:
This article introduces some memory optimization techniques and garbage collector management related knowledge in the Go language. We learned about techniques such as memory allocation, memory pools, pointers, and garbage collectors, and gave corresponding code examples. By rationally using these technologies, we can optimize the memory usage of the application and improve performance and stability.
References:
- Go language official documentation (https://golang.org/doc/)
- Go language bible (https://books. studygolang.com/gopl-zh/)
- Go language standard library (http://cngolib.com/)
- Go language advanced programming (https://book.eddycjy.com/ golang/)
The above is an article about memory optimization technology and garbage collector management in Go language. I hope it will be helpful to you.
The above is the detailed content of Explore memory optimization technology and garbage collector management in Go language. For more information, please follow other related articles on the PHP Chinese website!
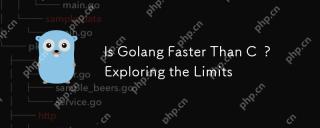
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
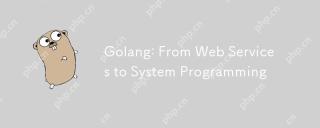
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
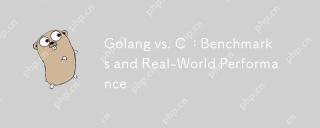
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
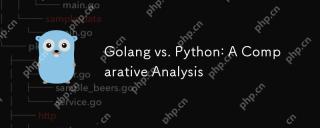
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.
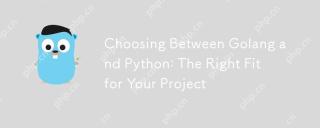
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
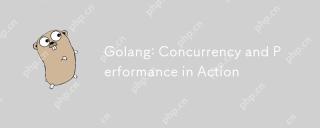
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
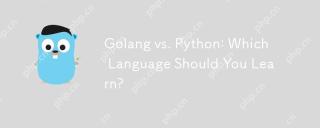
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
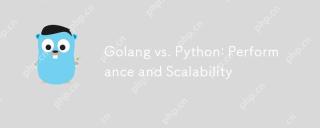
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
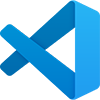
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft