


Golang and RabbitMQ implement an event-driven large-scale data processing system
Abstract:
In today's big data era, processing large-scale data has become a meet the needs of many enterprises. To handle this data efficiently, event-driven architectural patterns are becoming increasingly popular. Golang, as an efficient and reliable programming language, and RabbitMQ, as a reliable message queue system, can be used to build an efficient event-driven large-scale data processing system. This article will introduce how to use Golang and RabbitMQ to build such a system, and provide specific code examples.
- Introduction
With the rapid development of the Internet, massive amounts of data continue to emerge, and many companies are facing the challenge of processing this data. The traditional batch processing method can no longer meet the requirements for real-time and responsiveness, so the event-driven architecture model is gradually becoming popular. Event-driven architecture can better handle the challenges of large-scale data processing by splitting the system into discrete, autonomous components and communicating through message passing.
- Introduction to Golang and RabbitMQ
Golang is a high-level programming language developed by Google. It has the characteristics of high concurrency and high performance. Through Goroutine and Channel, Golang can easily implement concurrent and synchronous operations, which is very suitable for building efficient event-driven systems.
RabbitMQ is a reliable message queuing system based on the AMQP (Advanced Message Queuing Protocol) protocol, which provides a highly reliable and scalable message delivery mechanism. RabbitMQ can send messages from producers to multiple consumers, enabling decoupling and horizontal scalability.
- Building an event-driven data processing system
To demonstrate how to use Golang and RabbitMQ to build an event-driven data processing system, we assume that there is a requirement: from a folder Read files in and perform different processing according to different file types.
First, we need to create a producer to read files from the folder and send the file information to the RabbitMQ queue. The following is an example Golang code:
package main import ( "io/ioutil" "log" "os" "path/filepath" "github.com/streadway/amqp" ) func main() { conn, _ := amqp.Dial("amqp://guest:guest@localhost:5672/") defer conn.Close() ch, _ := conn.Channel() defer ch.Close() files, _ := ioutil.ReadDir("./folder") for _, file := range files { filePath := filepath.Join("./folder", file.Name()) data, _ := ioutil.ReadFile(filePath) msg := amqp.Publishing{ ContentType: "text/plain", Body: data, } ch.Publish( "", // exchange "file_queue", // routing key false, // mandatory false, // immediate msg, ) log.Printf("Sent file: %q", filePath) } }
In the above code, we use RabbitMQ’s Go client package github.com/streadway/amqp
to create a connection to the RabbitMQ server, And create a channel for communication with the server. We then use the ioutil.ReadDir
function to read the files in the folder and the ioutil.ReadFile
function to read the file contents. After that, we encapsulate the file content into the message body amqp.Publishing
, and use the ch.Publish
function to send the message to the RabbitMQ queue named file_queue
.
Then, we need to create a consumer to receive messages from the RabbitMQ queue and perform different processing according to the file type. The following is an example Golang code:
package main import ( "log" "github.com/streadway/amqp" ) func main() { conn, _ := amqp.Dial("amqp://guest:guest@localhost:5672/") defer conn.Close() ch, _ := conn.Channel() defer ch.Close() msgs, _ := ch.Consume( "file_queue", // queue "", // consumer true, // auto-ack true, // exclusive false, // no-local false, // no-wait nil, // args ) for msg := range msgs { // 根据文件类型处理消息 fileContentType := msg.ContentType switch fileContentType { case "text/plain": // 处理文本文件 log.Printf("Processing text file: %q", string(msg.Body)) case "image/jpeg": // 处理图片文件 log.Printf("Processing image file") // TODO: 处理图片文件的逻辑 default: // 处理其他文件类型 log.Printf("Processing unknown file type") // TODO: 处理未知文件类型的逻辑 } } }
In the above code, we also use RabbitMQ’s Go client packagegithub.com/streadway/amqp
to create a connection to the RabbitMQ server , and create a channel for communication with the server. Then, we use the ch.Consume
function to subscribe to consumer messages, and use for msg := range msgs
to receive messages in a loop. When processing messages, we determine the file type by checking the ContentType of the message, and perform corresponding processing logic based on different file types.
- Summary
This article introduces how to use Golang and RabbitMQ to build an event-driven large-scale data processing system. Through the high concurrency and high performance features of Golang and the reliable messaging mechanism of RabbitMQ, we can easily build an efficient and reliable data processing system. Not only that, Golang and RabbitMQ can also meet the requirements of real-time and responsiveness when processing large-scale data. This article provides specific code examples based on Golang and RabbitMQ to help readers understand how to apply this architectural pattern in actual projects.
Reference:
- Golang official website: https://golang.org/
- RabbitMQ official website: https://www.rabbitmq.com/
- RabbitMQ’s Go client package: https://github.com/streadway/amqp
The above is the detailed content of Golang and RabbitMQ implement event-driven large-scale data processing system. For more information, please follow other related articles on the PHP Chinese website!
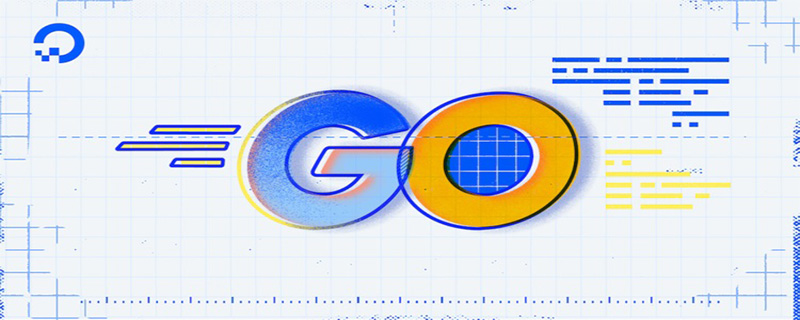
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
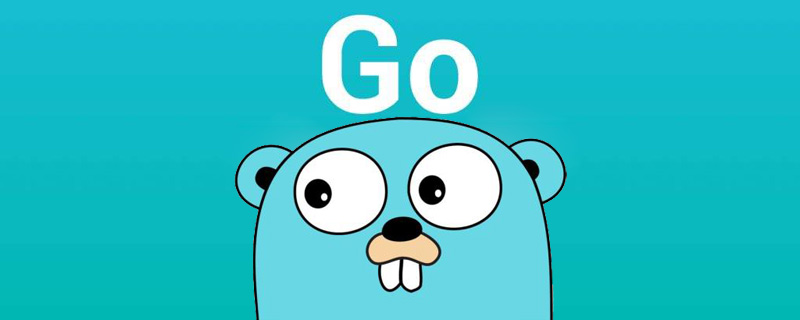
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
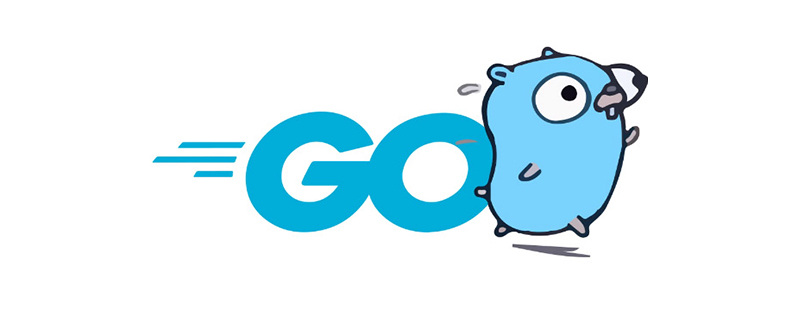
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
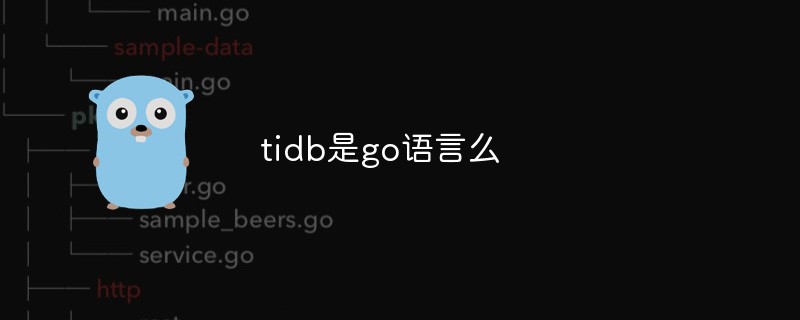
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
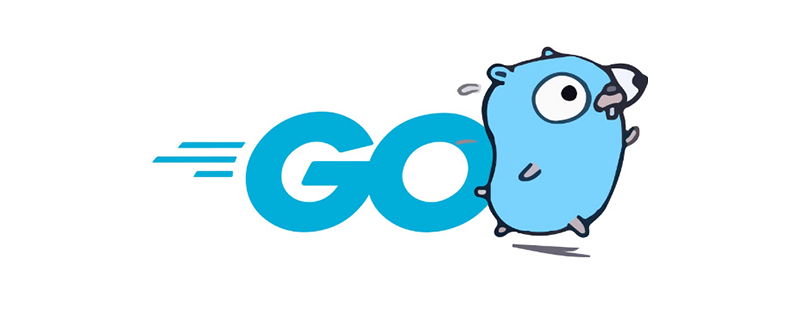
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
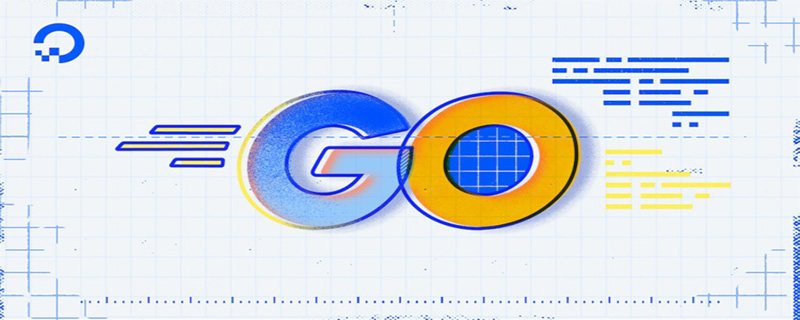
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
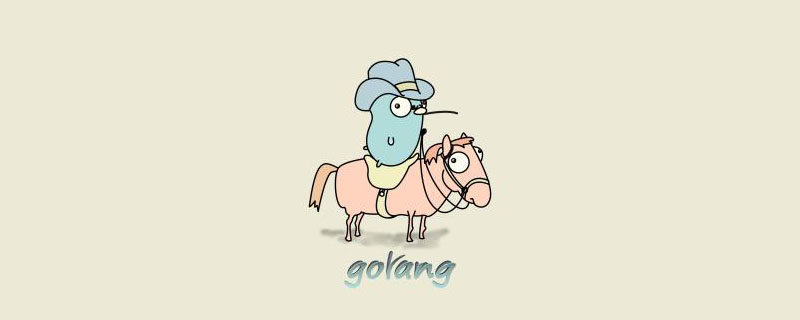
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
