Implement performance testing of database queries in React Query
To implement performance testing of database queries in React Query, specific code examples are required
As the complexity of front-end applications increases, for data processing and Management requirements are also becoming increasingly important. In front-end applications, data is usually stored in a database and read and written through the back-end interface. In order to ensure the efficient performance and user experience of the front-end page, we need to test and optimize the performance of the front-end data query.
React Query is a powerful data query and state management library, which provides us with the function of processing front-end data queries. When using React Query for database queries, we can take advantage of its data caching, query and automated request features to improve page performance and user experience.
In order to test the performance of React Query in database queries, we can write specific code examples and conduct some performance tests. The following is a sample code for a database query performance test based on React Query:
First, we need to install React Query.
npm install react-query
Then, we create a server-side interface for database query and use JSONPlaceholder to simulate database access.
// server.js const express = require('express'); const app = express(); const port = 3001; app.get('/users', (req, res) => { // Simulate the database query const users = [ { id: 1, name: 'John' }, { id: 2, name: 'Jane' }, { id: 3, name: 'Bob' }, // ... ]; res.json(users); }); app.listen(port, () => { console.log(`Server is running on port ${port}`); });
Next, we create a React component and use React Query to perform database queries. In this component, we use the useQuery hook to perform a database query and display the query results when the component renders.
// App.js import React from 'react'; import { useQuery, QueryClient, QueryClientProvider } from 'react-query'; // Create a new QueryClient const queryClient = new QueryClient(); const App = () => { // Define a query key const queryKey = 'users'; // Define a query function const fetchUsers = async () => { const response = await fetch('http://localhost:3001/users'); const data = response.json(); return data; }; // Execute the query and get the result const { status, data, error } = useQuery(queryKey, fetchUsers); // Render the result return ( <div> {status === 'loading' && <div>Loading...</div>} {status === 'error' && <div>Error: {error}</div>} {status === 'success' && ( <ul> {data.map((user) => ( <li key={user.id}>{user.name}</li> ))} </ul> )} </div> ); }; const WrappedApp = () => ( <QueryClientProvider client={queryClient}> <App /> </QueryClientProvider> ); export default WrappedApp;
Finally, we render the component in the application's entry file.
// index.js import React from 'react'; import ReactDOM from 'react-dom'; import App from './App'; ReactDOM.render( <React.StrictMode> <App /> </React.StrictMode>, document.getElementById('root') );
The above is a code example for implementing performance testing of database queries in React Query. By using functions such as data caching and automated requests provided by React Query, we can optimize the performance of front-end database queries and improve page response speed and user experience. At the same time, we can perform performance testing based on this sample code to evaluate and improve our front-end applications.
The above is the detailed content of Implement performance testing of database queries in React Query. For more information, please follow other related articles on the PHP Chinese website!
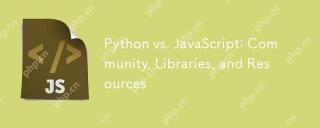
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
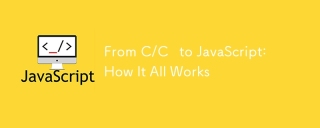
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
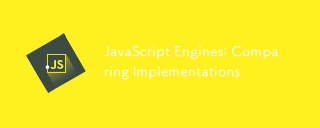
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
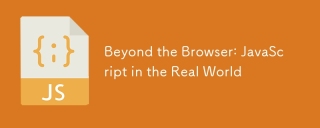
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
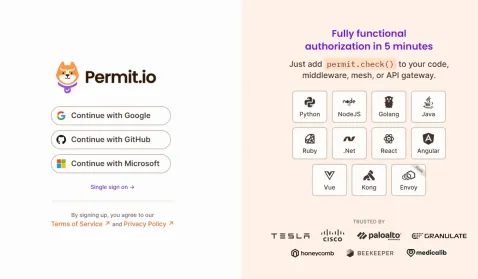
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
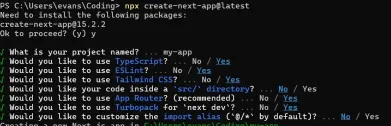
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
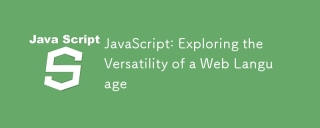
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
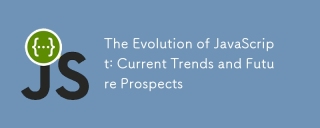
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
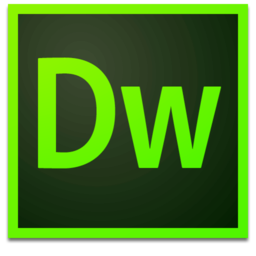
Dreamweaver Mac version
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.