Using React Query and database to implement data access control
In modern web applications, data access control is an integral part. It ensures that only authorized users can access and manipulate specific data. Using React Query combined with the database to control data access permissions can provide an efficient and scalable solution.
React Query is a powerful and flexible data retrieval and management library that handles data retrieval, caching and updating in an easy and intuitive way. It integrates well with various backends and databases, and can be easily integrated with authentication and authorization systems.
In this article, we will introduce the basic principles of how to use React Query and the database to implement data access control, and give some specific code examples.
- Define permission models and roles
First, we need to define permission models and roles. The permission model defines what data and operations exist in the system and gives the permissions that different roles have on these data and operations. A role is a set of permissions, and each user can be assigned one or more roles. - Set data access restrictions for different roles
According to the permission model and role definition, we can set data access restrictions for different roles. For example, one role might be able to read only specific data, while another role can read and modify all data. We can use React Query's query hooks to achieve these restrictions. Here is an example:
import { useQuery } from 'react-query'; const getData = async () => { // 这里是获取数据的逻辑 } const useRestrictedData = (role) => { const { data, isLoading, isError } = useQuery( 'restrictedData', getData, { enabled: role === 'admin', // 只有管理员角色可以访问 } ); return { data, isLoading, isError }; } function RestrictedDataComponent() { const { data, isLoading, isError } = useRestrictedData('admin'); if (isLoading) { return 'Loading...'; } if (isError) { return 'Error loading data.'; } return ( <div> {data.map((item) => ( <div key={item.id}>{item.name}</div> ))} </div> ); }
In the above example, only the administrator role can get restricted data through the useRestrictedData('admin')
hook. For other roles, the enabled
property is set to false
, so the query will not be triggered.
- Combined with the database for permission verification
To achieve true data access permission control, we need to combine the database for permission verification. This usually involves storing the user's role information in the database and validating the user's role before querying the data. Here is a simple example:
import { useQuery } from 'react-query'; import { db } from '../myDatabase'; // 假设我们使用了一个名为 db 的数据库库 const getData = async () => { const userRole = getCurrentUserRole(); // 获取当前用户的角色信息 if (userRole === 'admin') { return db.query('SELECT * FROM restrictedData'); } else { throw new Error('Unauthorized access'); } } const useRestrictedData = () => { const { data, isLoading, isError } = useQuery( 'restrictedData', getData ); return { data, isLoading, isError }; } // 省略其他代码...
In the above example, we used a hypothetical db
module to perform database query operations. In the getData
function, we obtain the current user's role information through the getCurrentUserRole()
function. If the user role is administrator, we perform database query operations, otherwise an unauthorized access error is thrown.
It should be noted that the database query logic in the above example is a simple example and not a real database access code. In practical applications, we need to write corresponding query code based on the specific backend and database.
Conclusion
Using React Query combined with the database, we can easily implement data access control. In this article, we introduced how to define permission models and roles, and gave example code for how to perform permission verification with React Query and a database. Of course, the specific implementation methods will vary depending on actual needs and technology stacks. I hope this article can help readers understand how to use React Query and database to achieve data access control, and provide some reference for the development of actual projects.
The above is the detailed content of Use React Query and database to control data access permissions. For more information, please follow other related articles on the PHP Chinese website!

本篇文章给大家带来了关于mysql的相关知识,其中主要介绍了关于索引优化器工作原理的相关内容,其中包括了MySQL Server的组成,MySQL优化器选择索引额原理以及SQL成本分析,最后通过 select 查询总结整个查询过程,下面一起来看一下,希望对大家有帮助。
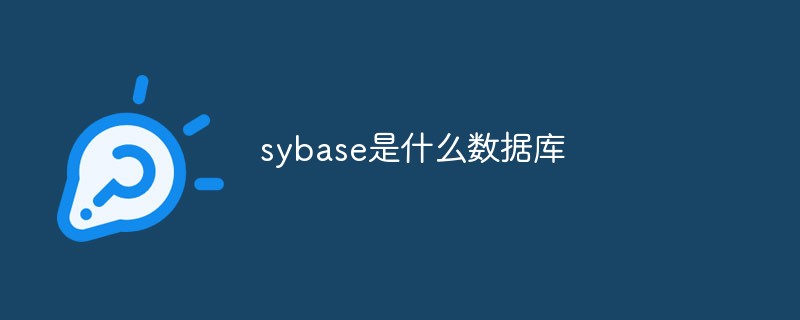
sybase是基于客户/服务器体系结构的数据库,是一个开放的、高性能的、可编程的数据库,可使用事件驱动的触发器、多线索化等来提高性能。
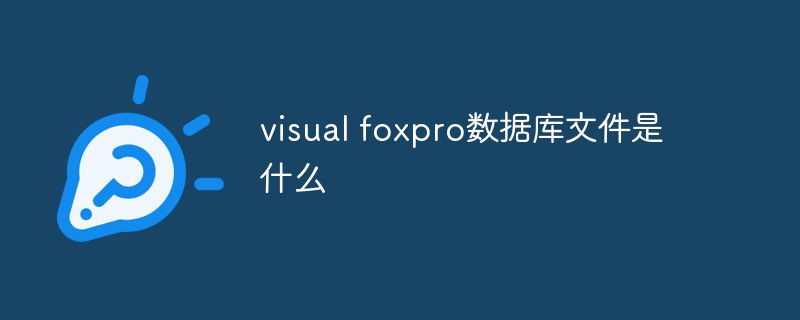
visual foxpro数据库文件是管理数据库对象的系统文件。在VFP中,用户数据是存放在“.DBF”表文件中;VFP的数据库文件(“.DBC”)中不存放用户数据,它只起将属于某一数据库的 数据库表与视图、连接、存储过程等关联起来的作用。
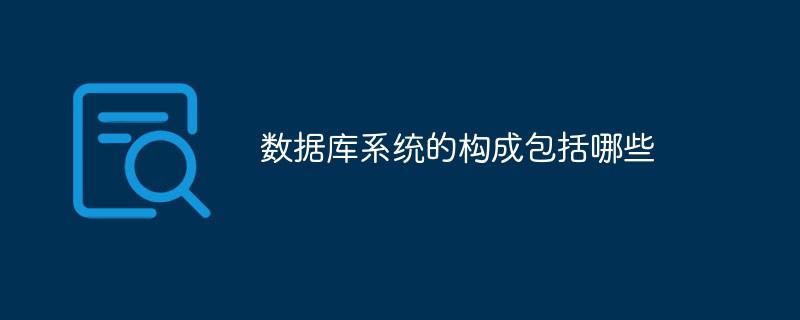
数据库系统由4个部分构成:1、数据库,是指长期存储在计算机内的,有组织,可共享的数据的集合;2、硬件,是指构成计算机系统的各种物理设备,包括存储所需的外部设备;3、软件,包括操作系统、数据库管理系统及应用程序;4、人员,包括系统分析员和数据库设计人员、应用程序员(负责编写使用数据库的应用程序)、最终用户(利用接口或查询语言访问数据库)、数据库管理员(负责数据库的总体信息控制)。
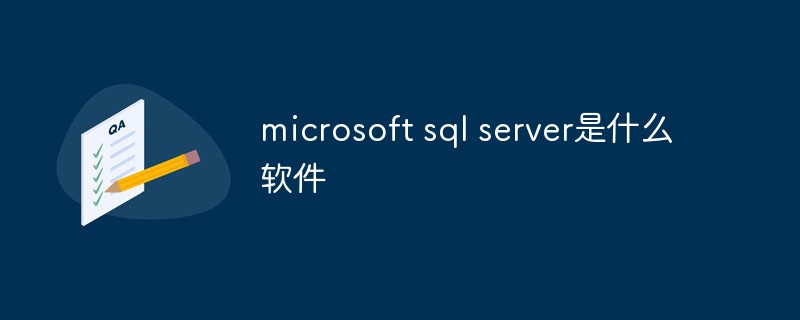
microsoft sql server是Microsoft公司推出的关系型数据库管理系统,是一个全面的数据库平台,使用集成的商业智能(BI)工具提供了企业级的数据管理,具有使用方便可伸缩性好与相关软件集成程度高等优点。SQL Server数据库引擎为关系型数据和结构化数据提供了更安全可靠的存储功能,使用户可以构建和管理用于业务的高可用和高性能的数据应用程序。
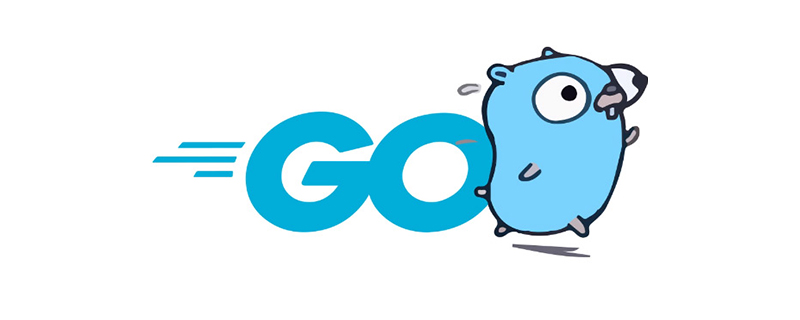
go语言可以写数据库。Go语言和其他语言不同的地方是,Go官方没有提供数据库驱动,而是编写了开发数据库驱动的标准接口,开发者可以根据定义的接口来开发相应的数据库驱动;这样做的好处在于,只要是按照标准接口开发的代码,以后迁移数据库时,不需要做任何修改,极大方便了后期的架构调整。
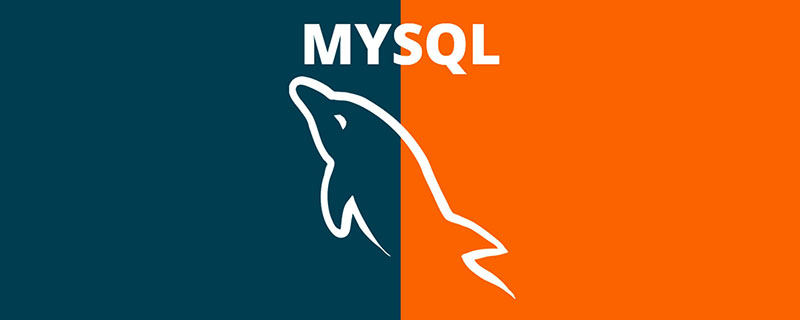
mysql查询为什么会慢,关于这个问题,在实际开发经常会遇到,而面试中,也是个高频题。遇到这种问题,我们一般也会想到是因为索引。那除开索引之外,还有哪些因素会导致数据库查询变慢呢?
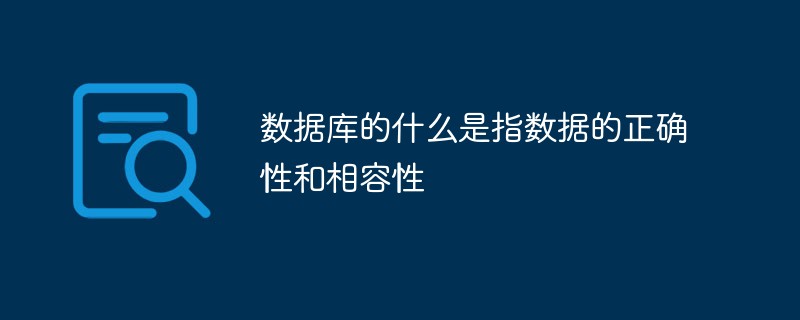
数据库的“完整性”是指数据的正确性和相容性。完整性是指数据库中数据在逻辑上的一致性、正确性、有效性和相容性。完整性对于数据库系统的重要性:1、数据库完整性约束能够防止合法用户使用数据库时向数据库中添加不合语义的数据;2、合理的数据库完整性设计,能够同时兼顾数据库的完整性和系统的效能;3、完善的数据库完整性有助于尽早发现应用软件的错误。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
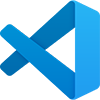
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
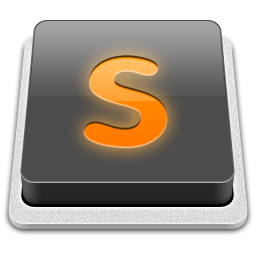
SublimeText3 Mac version
God-level code editing software (SublimeText3)
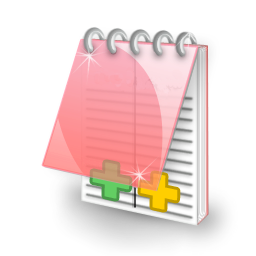
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
