Compute-intensive tasks: Optimize performance with Go WaitGroup
Computationally intensive tasks: Use Go WaitGroup to optimize performance
Overview:
In daily software development, we often encounter computationally intensive tasks, That is, tasks that require a lot of calculation and processing, which usually consume a lot of CPU resources and time. In order to improve performance, we can use WaitGroup in the Go language to implement concurrent calculations to optimize task execution efficiency.
What is WaitGroup?
WaitGroup is a mechanism in the Go language that can be used to wait for the end of a group of coroutines (goroutines). It provides three methods: Add(), Done() and Wait(). Add() is used to increase the number of waiting coroutines, Done() is used to mark the end of a coroutine, and Wait() is used to block and wait for the completion of all coroutines. Through WaitGroup, we can easily control the number of concurrent coroutines to make full use of CPU resources.
Example of using WaitGroup to optimize performance:
In order to better understand how to use WaitGroup to optimize the execution efficiency of computing-intensive tasks, let's take a look at a specific example.
package main import ( "fmt" "sync" ) func main() { var wg sync.WaitGroup // 增加等待的协程数量 wg.Add(3) go calculate(1, 10000, &wg) go calculate(10001, 20000, &wg) go calculate(20001, 30000, &wg) // 阻塞等待所有协程结束 wg.Wait() fmt.Println("All calculations have been completed.") } func calculate(start, end int, wg *sync.WaitGroup) { defer wg.Done() for i := start; i <= end; i++ { // 执行计算密集型任务 _ = i * 2 } }
In the above example, we used three coroutines to perform computationally intensive tasks. By segmenting the tasks, we can better utilize CPU resources. Each coroutine is responsible for processing calculations within a certain range. When the coroutine is completed, the Done() method is called to mark the task completion. Finally, the main coroutine blocks at the Wait() method and waits for all coroutines to end.
In this way, we can make full use of the advantages of multi-core CPUs and improve the execution efficiency of computing-intensive tasks. At the same time, using WaitGroup can also simplify concurrency control logic and make the code more concise and maintainable.
Summary:
Computing-intensive tasks consume a large amount of CPU resources. In order to improve performance, we can use WaitGroup in the Go language to implement concurrent computing. By rationally using WaitGroup, we can make full use of the capabilities of multi-core CPUs and improve task execution efficiency. In actual development, we can reasonably divide tasks according to specific business scenarios and needs, and use WaitGroup to control the number of concurrent coroutines, thereby optimizing the performance of computing-intensive tasks.
The above is the detailed content of Compute-intensive tasks: Optimize performance with Go WaitGroup. For more information, please follow other related articles on the PHP Chinese website!
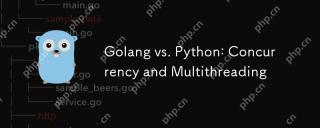
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
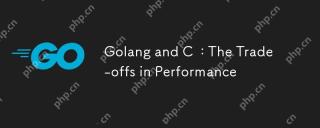
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
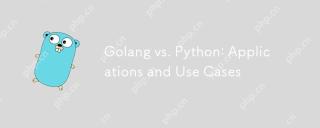
ChooseGolangforhighperformanceandconcurrency,idealforbackendservicesandnetworkprogramming;selectPythonforrapiddevelopment,datascience,andmachinelearningduetoitsversatilityandextensivelibraries.

Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
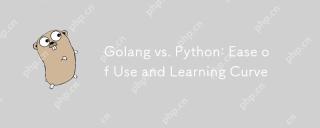
In what aspects are Golang and Python easier to use and have a smoother learning curve? Golang is more suitable for high concurrency and high performance needs, and the learning curve is relatively gentle for developers with C language background. Python is more suitable for data science and rapid prototyping, and the learning curve is very smooth for beginners.
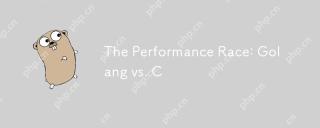
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
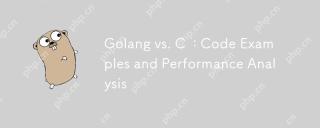
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
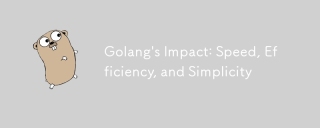
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Notepad++7.3.1
Easy-to-use and free code editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool