


Design and implementation of distributed system combining Golang and RabbitMQ
Distributed system design and implementation combining Golang and RabbitMQ
Abstract:
With the continuous development of the Internet and the continuous expansion of application scenarios, distributed systems The design and implementation are becoming increasingly important. This article will introduce how to use Golang and RabbitMQ to design a highly reliable distributed system, and provide specific code examples.
- Introduction
A distributed system refers to a system that works in parallel on multiple computers, communicating and coordinating through network connections to achieve a common goal. Compared with traditional single applications, distributed systems have higher scalability, reliability and recoverability. - Introduction to Golang
Golang is an efficient, concise and easy-to-use programming language. Its design goal is to provide high concurrency and high performance support. Golang's concurrency model is based on goroutines (lightweight threads) and channels (for communication between coroutines), which makes it ideal for distributed system development. - Introduction to RabbitMQ
RabbitMQ is an open source message middleware, which is based on AMQP (Advanced Message Queuing Protocol) and provides a reliable message delivery mechanism. RabbitMQ has the characteristics of high availability, scalability and flexibility, and can effectively support the communication and collaboration of distributed systems. - Design and Implementation of Distributed System
When designing a distributed system, you need to consider the following key factors:
4.1 Message Communication
Use RabbitMQ as the message middleman software that enables asynchronous communication between different components. By defining message queues and switches, reliable delivery and subscription functions of messages can be achieved.
4.2 Data Consistency
Data consistency in distributed systems is an important challenge. You can use the distributed lock or consistent hash algorithm provided by Golang to solve this problem.
4.3 Fault Tolerance
The fault tolerance of a distributed system refers to the system's ability to operate normally and automatically repair in the face of failures. Fault detection and automatic recovery can be achieved by monitoring the health status of components.
4.4 Logging and Monitoring
In a distributed system, logging and monitoring are very important. You can use Golang's log library and monitoring tools to achieve real-time log collection and system status monitoring.
- Code Example
The following is a simple distributed system example that demonstrates how to use Golang and RabbitMQ to design a distributed system based on message passing:
package main import ( "log" "github.com/streadway/amqp" ) func main() { conn, err := amqp.Dial("amqp://guest:guest@localhost:5672/") if err != nil { log.Fatalf("Failed to connect to RabbitMQ: %s", err) } defer conn.Close() ch, err := conn.Channel() if err != nil { log.Fatalf("Failed to open a channel: %s", err) } defer ch.Close() q, err := ch.QueueDeclare( "hello", false, false, false, false, nil, ) if err != nil { log.Fatalf("Failed to declare a queue: %s", err) } msgs, err := ch.Consume( q.Name, "", true, false, false, false, nil, ) if err != nil { log.Fatalf("Failed to register a consumer: %s", err) } forever := make(chan bool) go func() { for d := range msgs { log.Printf("Received a message: %s", d.Body) } }() log.Printf(" [*] Waiting for messages. To exit press CTRL+C") <-forever }
The above code connects to RabbitMQ and creates a consumer to receive messages from the queue "hello". Through coroutines to process messages concurrently, the basic communication functions of distributed systems are realized.
- Summary
This article introduces how to use Golang and RabbitMQ to design a highly reliable distributed system, and provides specific code examples. By using this combination, functions such as asynchronous communication, data consistency, fault tolerance, and monitoring of distributed systems can be achieved.
It is worth noting that in an actual production environment, the design and implementation of distributed systems need to consider more factors and require sufficient testing and optimization. Therefore, readers can expand and improve the above examples according to their own needs and actual conditions.
Reference:
- Golang official website: https://golang.org/
- RabbitMQ official website: https://www.rabbitmq.com/
- RabbitMQ Golang library: https://github.com/streadway/amqp
The above is the detailed content of Design and implementation of distributed system combining Golang and RabbitMQ. For more information, please follow other related articles on the PHP Chinese website!
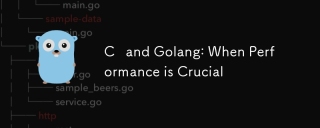
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
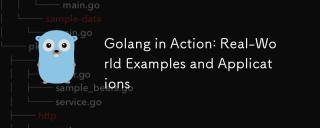
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
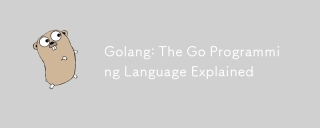
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
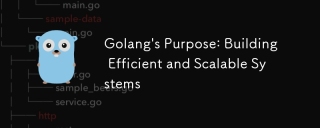
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
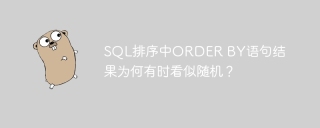
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
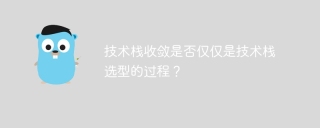
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
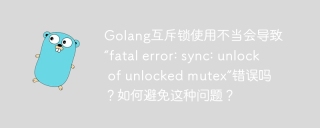
Golang ...
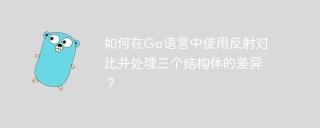
How to compare and handle three structures in Go language. In Go programming, it is sometimes necessary to compare the differences between two structures and apply these differences to the...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
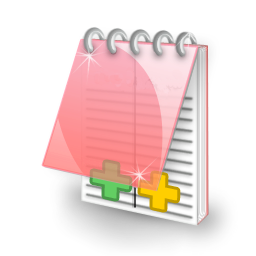
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use