


How to use PHP to write an employee attendance data cleaning tool?
How to use PHP to write an employee attendance data cleaning tool?
In modern enterprises, the accuracy and completeness of attendance data are crucial for management and salary payment. However, attendance data may contain erroneous, missing or inconsistent information for a variety of reasons. Therefore, developing an employee attendance data cleaning tool has become one of the necessary tasks. This article will describe how to write such a tool using PHP and provide some specific code examples.
First of all, let us clarify the functional requirements that the employee attendance data cleaning tool needs to meet:
- Clean data errors: delete invalid or wrong data, such as invalid attendance time , invalid employee number, etc.
- Fill in missing data: If the attendance data of an employee on a certain day is missing, it needs to be filled in based on other relevant data.
- Correction of data consistency: Compare different attendance data fields, and make corrections if the data is inconsistent.
Next, we will introduce step by step how to implement these functions step by step.
Step 1: Read the original data
We need an original attendance data file, which can be in CSV, Excel, database and other formats. First, use PHP to read this file and store the data as an array or object.
$data = []; $file = 'attendance_data.csv'; // 假设考勤数据保存在CSV文件中 $handle = fopen($file, 'r'); if ($handle) { while (($line = fgets($handle)) !== false) { // 解析一行数据 $row = str_getcsv($line); // 将数据存储到数组中 $data[] = $row; } fclose($handle); } else { echo "无法打开文件:$file"; }
Step 2: Clean data errors
For each row in the attendance data, we need to perform some basic data cleaning, such as deleting invalid attendance time or invalid employee number.
foreach ($data as $index => $row) { // 清理无效的考勤时间 if (!isValidDate($row['attendance_date'])) { unset($data[$index]); continue; } // 清理无效的员工编号 if (!isValidEmployeeId($row['employee_id'])) { unset($data[$index]); } } function isValidDate($date) { // 自定义日期验证逻辑,例如检查日期格式是否正确 // 返回TRUE或FALSE } function isValidEmployeeId($id) { // 自定义员工编号验证逻辑,例如判断是否为整数 // 返回TRUE或FALSE }
Step 3: Fill in missing data
If the attendance data of an employee on a certain day is missing, we need to fill it in based on other related data. For example, you can find the employee's attendance data on the previous day and the next day for inference.
foreach ($data as $index => $row) { // 查找缺失数据 if (empty($row['attendance'])) { $previousDayData = findPreviousDayData($row['employee_id'], $row['attendance_date']); $nextDayData = findNextDayData($row['employee_id'], $row['attendance_date']); // 根据相关数据推断考勤情况 $attendance = inferAttendance($previousDayData, $nextDayData); // 填充缺失数据 $data[$index]['attendance'] = $attendance; } } function findPreviousDayData($employeeId, $date) { // 根据员工编号和日期获取前一天的考勤数据 // 返回相关数据 } function findNextDayData($employeeId, $date) { // 根据员工编号和日期获取后一天的考勤数据 // 返回相关数据 } function inferAttendance($previousData, $nextData) { // 根据前一天和后一天的考勤数据进行推断 // 返回推断的考勤情况 }
Step 4: Correct data consistency
In order to ensure the consistency of attendance data, we need to compare different attendance data fields and make corrections as needed. For example, employees' tardiness and early departure can be judged based on the start and end times.
foreach ($data as $index => $row) { // 比较上班时间和下班时间 if ($row['start_time'] > $row['end_time']) { // 进行校正,例如交换上班时间和下班时间 $temp = $data[$index]['start_time']; $data[$index]['start_time'] = $data[$index]['end_time']; $data[$index]['end_time'] = $temp; } }
Finally, we can write the processed data back to the file or upload it to the database for further analysis and use.
To sum up, this article introduces how to use PHP to write an employee attendance data cleaning tool. This tool ensures the accuracy and completeness of attendance data by cleaning data errors, filling in missing data, and correcting data consistency. Through specific code examples, readers can better understand how to implement these functions. I hope this article will be helpful to readers who need to develop employee attendance data cleaning tools.
The above is the detailed content of How to use PHP to write an employee attendance data cleaning tool?. For more information, please follow other related articles on the PHP Chinese website!
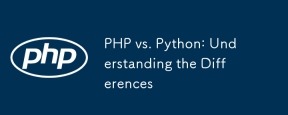
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
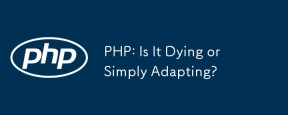
PHP is not dying, but constantly adapting and evolving. 1) PHP has undergone multiple version iterations since 1994 to adapt to new technology trends. 2) It is currently widely used in e-commerce, content management systems and other fields. 3) PHP8 introduces JIT compiler and other functions to improve performance and modernization. 4) Use OPcache and follow PSR-12 standards to optimize performance and code quality.
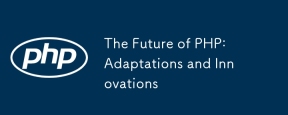
The future of PHP will be achieved by adapting to new technology trends and introducing innovative features: 1) Adapting to cloud computing, containerization and microservice architectures, supporting Docker and Kubernetes; 2) introducing JIT compilers and enumeration types to improve performance and data processing efficiency; 3) Continuously optimize performance and promote best practices.
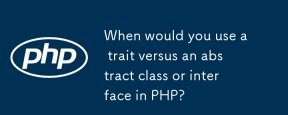
In PHP, trait is suitable for situations where method reuse is required but not suitable for inheritance. 1) Trait allows multiplexing methods in classes to avoid multiple inheritance complexity. 2) When using trait, you need to pay attention to method conflicts, which can be resolved through the alternative and as keywords. 3) Overuse of trait should be avoided and its single responsibility should be maintained to optimize performance and improve code maintainability.
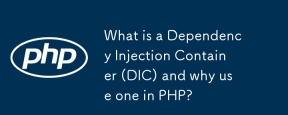
Dependency Injection Container (DIC) is a tool that manages and provides object dependencies for use in PHP projects. The main benefits of DIC include: 1. Decoupling, making components independent, and the code is easy to maintain and test; 2. Flexibility, easy to replace or modify dependencies; 3. Testability, convenient for injecting mock objects for unit testing.
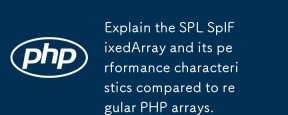
SplFixedArray is a fixed-size array in PHP, suitable for scenarios where high performance and low memory usage are required. 1) It needs to specify the size when creating to avoid the overhead caused by dynamic adjustment. 2) Based on C language array, directly operates memory and fast access speed. 3) Suitable for large-scale data processing and memory-sensitive environments, but it needs to be used with caution because its size is fixed.
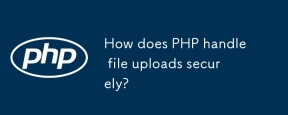
PHP handles file uploads through the $\_FILES variable. The methods to ensure security include: 1. Check upload errors, 2. Verify file type and size, 3. Prevent file overwriting, 4. Move files to a permanent storage location.
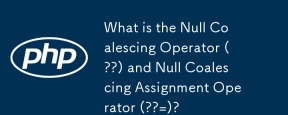
In JavaScript, you can use NullCoalescingOperator(??) and NullCoalescingAssignmentOperator(??=). 1.??Returns the first non-null or non-undefined operand. 2.??= Assign the variable to the value of the right operand, but only if the variable is null or undefined. These operators simplify code logic, improve readability and performance.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Notepad++7.3.1
Easy-to-use and free code editor
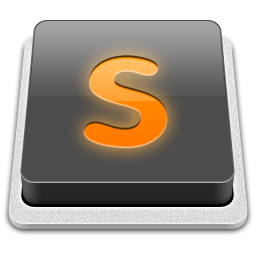
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.