


Using Java to implement the test score analysis function of the online examination system
Title: Using Java to implement the test score analysis function of the online examination system
[Introduction]
With the rapid development of online education, more and more Students take exams online. In order to better understand students' learning situation and provide personalized tutoring services, the test score analysis function of online examination systems has become very important. This article will introduce the specific method of implementing this function using the Java programming language and provide corresponding code examples.
[Implementation method]
In order to implement the test score analysis function of the online examination system, we need the following steps:
- Data storage: Obtain students from the database or other data sources test score data. Relational databases such as MySQL and Oracle can be used to store student performance data.
- Data processing: Properly process the obtained data for performance analysis. For example, you can calculate a student's average grade, highest grade, lowest grade, etc. At the same time, you can sort, group and other operations on the results.
- Score analysis: Analyze score data according to needs. For example, statistical indicators such as average scores and passing rates for each subject can be calculated, and information such as score distribution charts and rankings can be generated.
- Result display: Display the analysis results to users in an appropriate way. For example, you can generate reports, charts, or output in text form.
The specific code examples are as follows:
import java.util.ArrayList; import java.util.Collections; class Student { private String name; private int score; // 构造方法 public Student(String name, int score) { this.name = name; this.score = score; } // 获取姓名 public String getName() { return name; } // 获取成绩 public int getScore() { return score; } } public class ExamScoreAnalyzer { public static void main(String[] args) { // 模拟从数据源获取学生成绩数据 ArrayList<Student> students = new ArrayList<>(); students.add(new Student("小明", 80)); students.add(new Student("小红", 90)); students.add(new Student("小刚", 70)); students.add(new Student("小李", 85)); students.add(new Student("小张", 95)); // 计算平均成绩 int sum = 0; for (Student student : students) { sum += student.getScore(); } double averageScore = sum / students.size(); System.out.println("平均成绩:" + averageScore); // 计算最高成绩 int maxScore = Collections.max(students, (s1, s2) -> s1.getScore() - s2.getScore()).getScore(); System.out.println("最高成绩:" + maxScore); // 计算最低成绩 int minScore = Collections.min(students, (s1, s2) -> s1.getScore() - s2.getScore()).getScore(); System.out.println("最低成绩:" + minScore); // 成绩排序 Collections.sort(students, (s1, s2) -> s1.getScore() - s2.getScore()); System.out.println("成绩排序:"); for (Student student : students) { System.out.println(student.getName() + ":" + student.getScore()); } } }
[Summary]
Through the above example code, we can see how to use Java language to implement the test score analysis function of the online examination system. Through the four steps of data storage, data processing, score analysis and result display, we can quickly count and analyze students' test scores and obtain corresponding analysis results. This analysis function is very valuable for both online education platforms and individual students, and can improve learning effects and teaching quality to a certain extent.
The above is the detailed content of Using Java to implement the test score analysis function of the online examination system. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
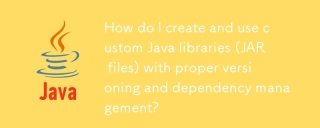
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
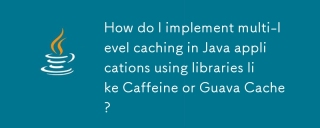
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
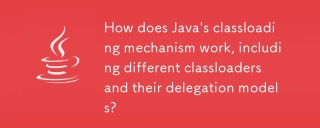
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
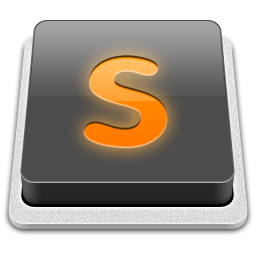
SublimeText3 Mac version
God-level code editing software (SublimeText3)
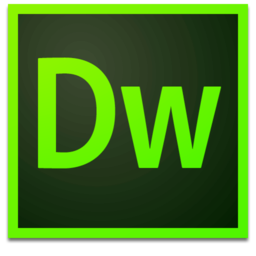
Dreamweaver Mac version
Visual web development tools