


How to add batch operation function to the accounting system - using PHP to develop batch operation methods requires specific code examples
With the development of the Internet, the accounting system is in plays an important role in our daily lives. However, as time passes and the amount of data increases, manual operations become less efficient. In order to improve users' work efficiency, we can add batch operation functions to the accounting system. This article will introduce how to use PHP to develop batch operations and provide specific code examples.
1. Requirements Analysis
Before adding the batch operation function, we first need to clarify the user's needs. In accounting systems, common batch operation functions include batch deletion, batch editing, batch import, and batch export.
2. PHP development batch operation method
- Batch deletion
Batch deletion is one of the most common batch operations in the accounting system. We can implement the batch deletion function through the following steps:
(1) Display the list of data to be deleted on the front-end page, and provide check boxes for users to select the data that needs to be deleted.
(2) After the user selects the data to be deleted, click the delete button.
(3) The front end sends the selected data ID to the background PHP file for processing through AJAX.
(4) After the background PHP file receives the data ID, it deletes the selected data from the database through SQL statements.
The specific code examples are as follows:
Front-end page (HTML):
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>批量删除</title> </head> <body> <form id="deleteForm" method="POST"> <table> <tr> <th>选择</th> <th>数据</th> </tr> <tr> <td><input type="checkbox" name="data[]" value="1"></td> <td>数据1</td> </tr> <tr> <td><input type="checkbox" name="data[]" value="2"></td> <td>数据2</td> </tr> <tr> <td><input type="checkbox" name="data[]" value="3"></td> <td>数据3</td> </tr> </table> <input type="submit" value="删除"> </form> </body> </html>
Backend PHP file:
<?php // 连接数据库 $conn = mysqli_connect("localhost", "root", "password", "database"); // 检查是否连接成功 if (mysqli_connect_errno()) { echo "Failed to connect to MySQL: " . mysqli_connect_error(); } if ($_SERVER["REQUEST_METHOD"] == "POST") { // 获取选中的数据ID $dataIds = $_POST["data"]; // 删除数据 foreach ($dataIds as $dataId) { $sql = "DELETE FROM table_name WHERE id = " . $dataId; mysqli_query($conn, $sql); } } // 关闭数据库连接 mysqli_close($conn); ?>
- Batch editing
The batch editing function allows users to modify the properties of multiple pieces of data at the same time. The method of implementing batch editing is similar to batch deletion. You only need to change the deletion operation to an update operation. The specific code example is similar to batch deletion and will not be repeated here. - Batch import
Batch import is a very practical function, which can import the user's existing data into the accounting system in batches. We can implement the batch import function through the following steps:
(1) The front-end page provides a file upload form to allow users to select the files that need to be imported.
(2) The front end sends the file to the background PHP file for processing through AJAX.
(3) After receiving the file, the background PHP file parses the file data and stores the parsed data in the database.
The specific code examples are as follows:
Front-end page (HTML):
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>批量导入</title> </head> <body> <form id="importForm" method="POST" enctype="multipart/form-data"> <input type="file" name="file" accept=".csv"> <input type="submit" value="导入"> </form> </body> </html>
Backend PHP file:
<?php // 连接数据库 $conn = mysqli_connect("localhost", "root", "password", "database"); // 检查是否连接成功 if (mysqli_connect_errno()) { echo "Failed to connect to MySQL: " . mysqli_connect_error(); } if ($_SERVER["REQUEST_METHOD"] == "POST") { // 获取上传的文件名和临时文件路径 $fileName = $_FILES["file"]["name"]; $tempName = $_FILES["file"]["tmp_name"]; // 读取文件数据 $file = fopen($tempName, "r"); while (($data = fgetcsv($file, 1000, ",")) !== FALSE) { // 解析文件数据并插入数据库 $sql = "INSERT INTO table_name (column1, column2, column3) VALUES ('" . $data[0] . "', '" . $data[1] . "', '" . $data[2] . "')"; mysqli_query($conn, $sql); } fclose($file); } // 关闭数据库连接 mysqli_close($conn); ?>
- Batch export
The batch export function can export data in the database to files in specified formats, such as CSV files or Excel files. The method of implementing batch export is similar to batch import. You only need to save the data in the file and read the data from the file. The specific code example is similar to batch import and will not be described again here.
Through the above code examples, we can add batch operation functions to the accounting system to improve user work efficiency. Of course, the code implementation may be different depending on specific project needs, and needs to be adapted and adjusted according to the actual situation. Hope this article is helpful to you.
The above is the detailed content of How to add batch operation functionality to the accounting system - How to develop batch operations using PHP. For more information, please follow other related articles on the PHP Chinese website!
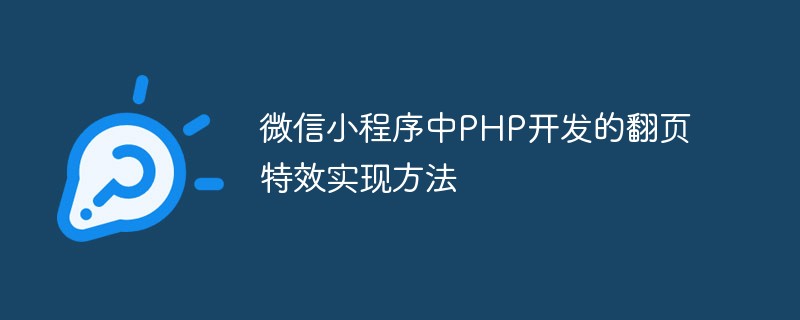
在微信小程序中,PHP开发的翻页特效是非常常见的功能。通过这种特效,用户可以轻松地在不同的页面之间进行切换,浏览更多的内容。在本文中,我们将介绍如何使用PHP来实现微信小程序中的翻页特效。我们将会讲解一些基本的PHP知识和技巧,以及一些实际的代码示例。理解基本的PHP语言知识在PHP中,我们经常会用到IF/ELSE语句、循环结构,以及函数等一些基本语言知识。
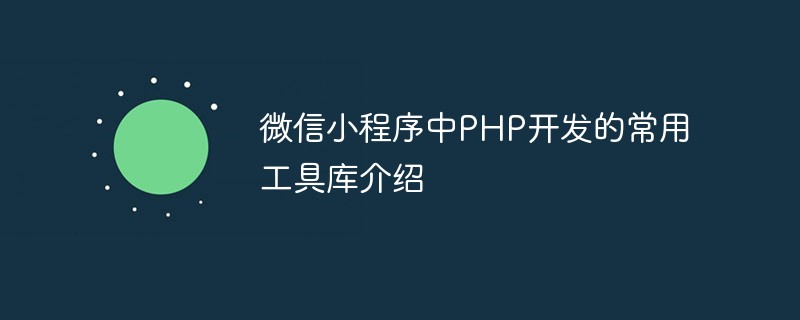
随着微信小程序的普及和发展,越来越多的开发者开始涉足其中。而PHP作为一种后端技术的代表,也在小程序中得到了广泛的运用。在小程序的开发中,PHP常用工具库也是很重要的一个部分。本文将介绍几款比较实用的PHP常用工具库,供大家参考。一、EasyWeChatEasyWeChat是一个开源的微信开发工具库,用于快速开发微信应用。它提供了一些常用的微信接口,如微信公
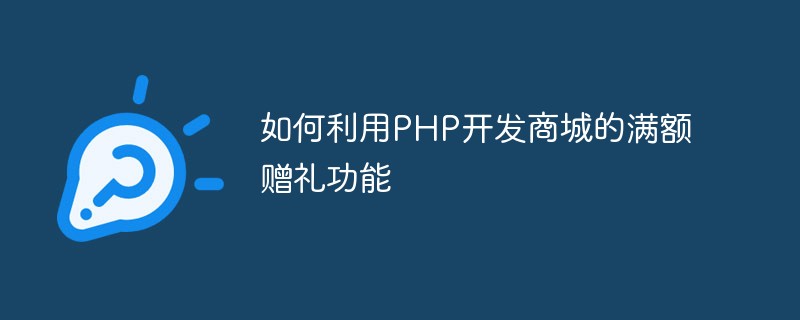
网上购物已经成为人们日常生活中不可或缺的一部分,因此,越来越多的企业开始关注电商领域。开发一款实用、易用的商城网站也成为了企业提高销售额、拓展市场的必要手段之一。在商城网站中,满额赠礼功能是提高用户购买欲望和促进销售增长的重要功能之一。本文将探讨如何利用PHP开发商城的满额赠礼功能。一、满额赠礼功能的实现思路在商城开发中,如何实现满额赠礼功能呢?简单来说就是
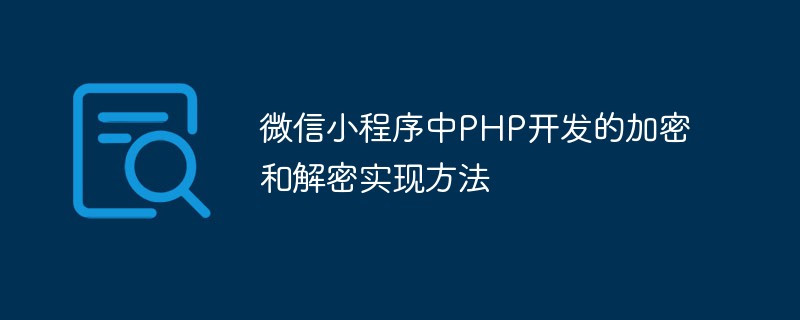
随着微信小程序在移动应用市场中越来越流行,它的开发也受到越来越多的关注。在小程序中,PHP作为一种常用的后端语言,经常用于处理敏感数据的加密和解密。本文将介绍在微信小程序中如何使用PHP实现加密和解密。一、什么是加密和解密?加密是将敏感数据转换为不可读的形式,以确保数据在传输过程中不被窃取或篡改。解密是将加密数据还原为原始数据。在小程序中,加密和解密通常包括
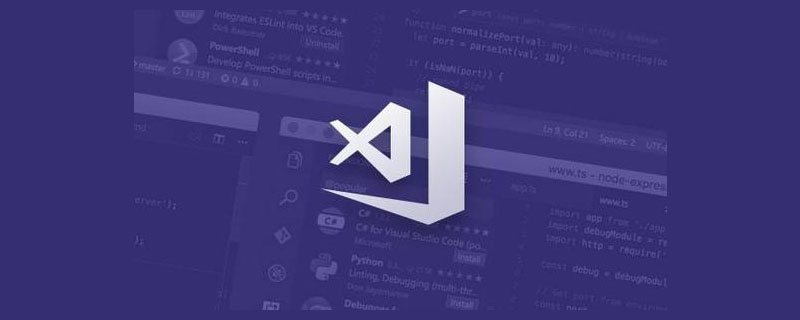
本篇文章给大家推荐一些VSCode+PHP开发中实用的插件。有一定的参考价值,有需要的朋友可以参考一下,希望对大家有所帮助。
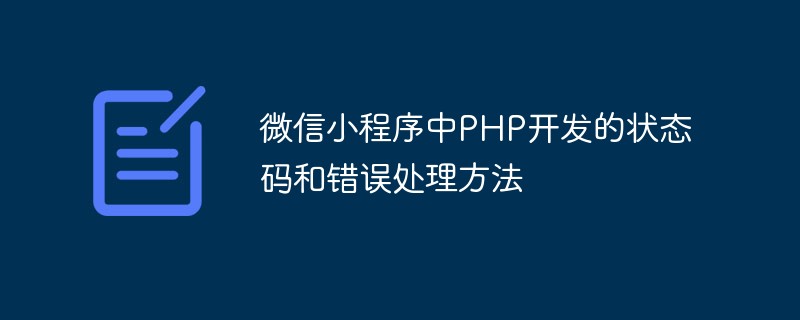
作为目前最流行的社交媒体平台之一,微信已经成为了企业和个人互动沟通的重要渠道之一。而微信小程序则更加方便用户使用和开发者创新。在小程序开发中,PHP是一种重要的后端技术,但是在开发过程中很容易出现状态码和错误处理方面的问题。本文将介绍微信小程序中PHP开发的各种状态码和错误处理方法。一、微信小程序中的状态码在微信小程序中,HTTP状态码(HTTPStatu
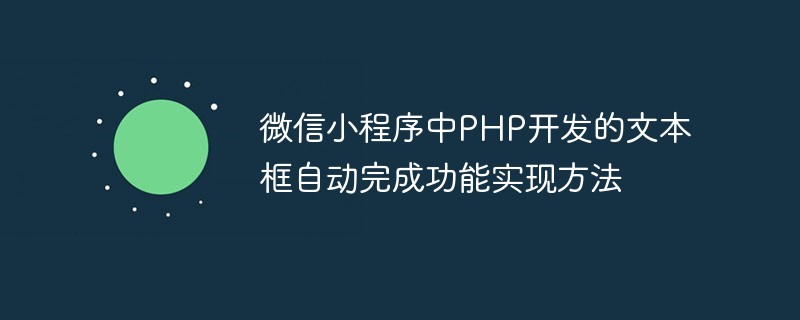
随着微信小程序的普及,各类开发需求也日渐增多。其中,文本框自动完成功能是小程序中常用的功能之一。虽然微信小程序提供了一些原生的组件,但是有一些特殊需求还是需要进行二次开发。本文将介绍如何使用PHP语言实现微信小程序中文本框自动完成功能。准备工作在开始开发之前,需要准备一些基本的环境和工具。首先,需要安装好PHP环境。其次,需要在微信小程序后台获取到自己的Ap
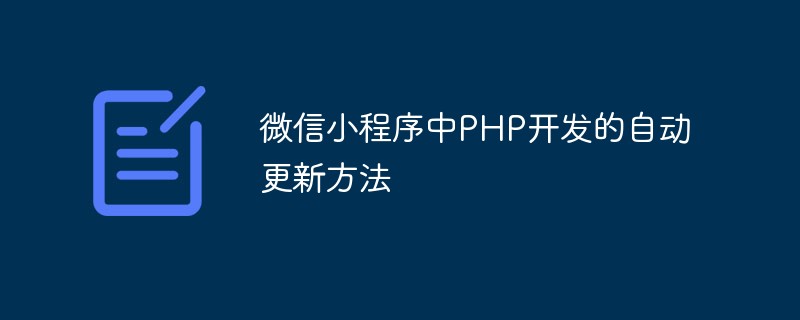
近年来,移动互联网的快速发展和移动终端的普及,让微信应用程序成为了人们生活中不可或缺的一部分。而在微信应用程序中,小程序更是以其轻量、快速、便捷的特点受到了广泛的欢迎。但是,对于小程序中的数据更新问题,却成为了一个比较头疼的问题。为了解决这一问题,我们可以使用PHP开发的自动更新方法来实现自动化数据更新。本篇文章就来探讨一下微信小程序中PHP开发的自动更新方


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
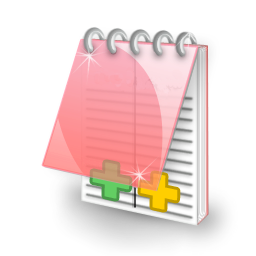
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
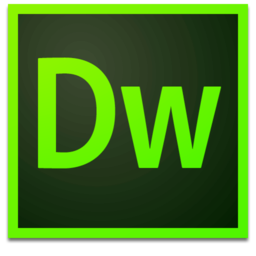
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor
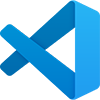
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
