


The cold chain logistics management and temperature monitoring functions of the Java warehouse management system require specific code examples
With the continuous development of the logistics industry, cold chain logistics has become increasingly important in food, It plays an important role in pharmaceutical and other industries. In order to ensure the safety and quality of goods during the logistics process, the warehouse management system needs to have the functions of cold chain logistics management and temperature monitoring.
Cold chain logistics management mainly includes cargo tracking and positioning, temperature and humidity monitoring and alarms, transportation route planning, etc. Temperature monitoring is one of the most important links in cold chain logistics. By monitoring and recording the temperature changes of goods in real time, abnormalities can be discovered in time and corresponding measures can be taken to ensure the quality of the goods.
Below, we will use a simple sample code to demonstrate the implementation of the cold chain logistics management and temperature monitoring functions of the Java warehouse management system.
First, we need to create a temperature sensor class named TemperatureSensor
to simulate temperature collection. The sample code is as follows:
public class TemperatureSensor { public double getTemperature() { // 模拟温度采集 Random random = new Random(); double temperature = random.nextDouble() * 10 + 20; // 生成20~30之间的随机温度 return temperature; } }
Next, we create a warehouse class named Warehouse
for managing goods and monitoring temperature. The sample code is as follows:
public class Warehouse { private List<Goods> goodsList; private TemperatureSensor temperatureSensor; public Warehouse() { goodsList = new ArrayList<>(); temperatureSensor = new TemperatureSensor(); } public void addGoods(Goods goods) { goodsList.add(goods); } public void removeGoods(Goods goods) { goodsList.remove(goods); } public void checkTemperature() { double temperature = temperatureSensor.getTemperature(); for (Goods goods : goodsList) { if (goods.getTemperatureRange().contains(temperature)) { System.out.println("货物:" + goods.getName() + " 温度正常"); } else { System.out.println("货物:" + goods.getName() + " 温度异常,当前温度为:" + temperature); } } } }
Next, we create a goods class named Goods
to manage the information and temperature range of the goods. The sample code is as follows:
public class Goods { private String name; private Range<Double> temperatureRange; public Goods(String name, Range<Double> temperatureRange) { this.name = name; this.temperatureRange = temperatureRange; } public String getName() { return name; } public Range<Double> getTemperatureRange() { return temperatureRange; } }
Finally, we create a main class named Main
to test the functionality of the warehouse management system. The sample code is as follows:
public class Main { public static void main(String[] args) { Warehouse warehouse = new Warehouse(); Goods goods1 = new Goods("苹果", Range.closed(0.0, 10.0)); // 苹果的温度范围为0~10度 Goods goods2 = new Goods("牛奶", Range.closed(2.0, 8.0)); // 牛奶的温度范围为2~8度 warehouse.addGoods(goods1); warehouse.addGoods(goods2); for (int i = 0; i < 10; i++) { warehouse.checkTemperature(); // 每隔一段时间检查温度 try { Thread.sleep(1000); } catch (InterruptedException e) { e.printStackTrace(); } } } }
Through the above sample code, we created a simple warehouse management system and implemented the functions of cold chain logistics management and temperature monitoring. Among them, TemperatureSensor
simulates the temperature collection process of the temperature sensor, the Warehouse
class manages the goods and temperature sensors, and monitors the temperature of the goods through the checkTemperature()
method , Goods
class manages the information and temperature range of the goods, and Main
class tests the functions of the warehouse management system.
Of course, the above is just a simplified example, and actual warehouse management systems involve more functions and complexities. But through this example, you can clearly understand the basic implementation of cold chain logistics management and temperature monitoring of the Java warehouse management system.
The above is the detailed content of Cold chain logistics management and temperature monitoring functions of Java warehouse management system. For more information, please follow other related articles on the PHP Chinese website!
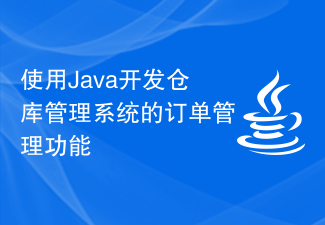
Java仓库管理系统的订单管理功能订单管理是仓库管理系统的重要功能之一。通过订单管理,可以实现对仓库中的产品进行购买、查看、修改和删除等操作。在本文中,将介绍如何使用Java开发仓库管理系统的订单管理功能,并提供具体的代码示例。系统需求分析在开发订单管理功能之前,首先需要进行系统需求分析。根据实际需求,订单管理功能应包括以下基本功能:添加订单:将产品添加到订
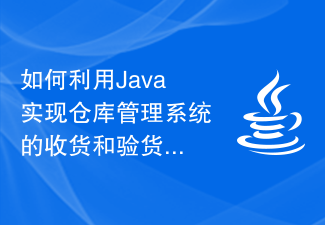
如何利用Java实现仓库管理系统的收货和验货功能随着电商的发展,仓库管理系统的重要性不可忽视。在仓库管理系统中,收货和验货是至关重要的环节。本文将介绍如何利用Java实现仓库管理系统中的收货和验货功能,并提供具体的代码示例。1.收货功能的实现收货功能是指将货物从供应商处接收并入库的过程。在仓库管理系统中,我们可以通过以下步骤实现收货功能。1.1创建货物类首
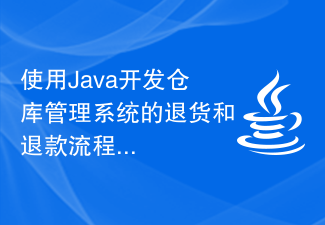
使用Java开发仓库管理系统的退货和退款流程标题:Java仓库管理系统的退货和退款流程及代码示例一、引言随着电子商务的快速发展,仓库管理系统成为了现代物流行业中不可或缺的一部分。而其中,退货和退款流程则是仓库管理系统中一项重要的功能。本文将介绍如何使用Java语言开发一个完善的仓库管理系统中的退货和退款流程,并提供相关的代码示例。二、退货流程设计用户发起退货
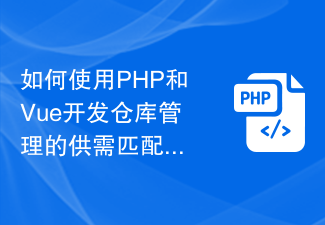
如何使用PHP和Vue开发仓库管理的供需匹配功能在物流和供应链管理中,仓库管理是一项重要的任务。一个有效的仓库管理系统可以提高物流效率,减少成本,并确保库存的准确性。为了更好地管理仓库,供需匹配功能是一项必不可少的功能。本文将介绍如何使用PHP和Vue来开发仓库管理的供需匹配功能,并提供具体的代码示例。一、需求分析在开发仓库管理的供需匹配功能之前,我们首先需
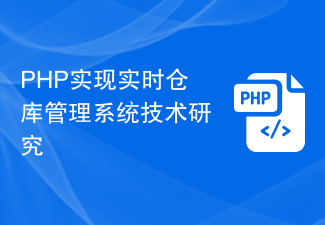
随着电子商务行业的不断发展,仓库管理成为企业供应链管理的重要环节之一。传统的仓库管理方式已经无法满足企业的需求,如何实现高效、精准的仓库管理成为企业亟需解决的问题。本文将针对PHP实现实时仓库管理系统的技术研究进行探讨和分析。一、仓库管理系统的概述仓库管理系统是企业为管理和控制库存而建立的系统化管理平台,它主要包括库房管理、货品入库、货品出库、库存盘点和供应
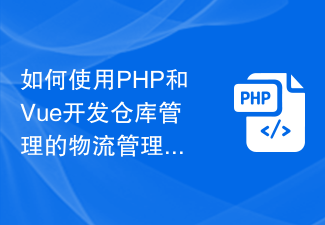
如何使用PHP和Vue开发仓库管理的物流管理功能随着电子商务的快速发展,仓库管理的物流管理功能变得越来越重要。在这篇文章中,我将介绍如何使用PHP和Vue来开发一个简单而实用的仓库管理系统,并提供具体的代码示例。环境准备在开始开发之前,我们需要准备一些开发环境。首先,确保你的电脑上已经安装了PHP和Vue的开发环境。你可以通过下载和安装XAMPP、WAMP或
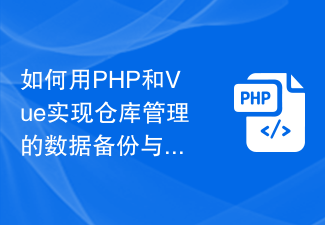
如何用PHP和Vue实现仓库管理的数据备份与恢复功能,需要具体代码示例在现代仓库管理系统中,数据备份与恢复是不可或缺的功能之一。仓库管理涉及大量的数据,包括库存信息、入库记录、出库记录等。因此,保证数据的安全性和可靠性是至关重要的。在本文中,将介绍如何使用PHP和Vue来实现仓库管理的数据备份与恢复功能,同时给出具体的代码示例。一、数据备份功能创建数据库和表
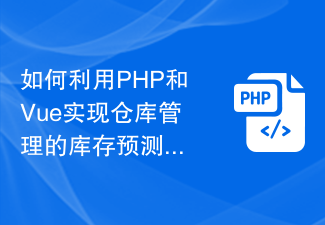
如何利用PHP和Vue实现仓库管理的库存预测功能,需要具体代码示例【引言】在仓库管理系统中,库存的预测是至关重要的一环,它能够帮助仓库管理员提前做好备货和销售规划,从而提高仓库的运营效率和利润。本文将介绍如何利用PHP和Vue这两个流行的开发工具,来实现仓库管理中的库存预测功能,给出具体的代码示例。【背景】仓库管理系统通常涉及到商品的进货、销售、库存等流程,


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
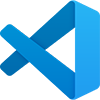
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
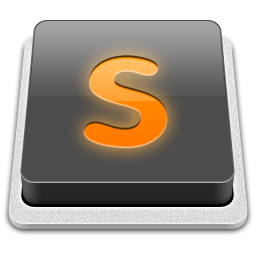
SublimeText3 Mac version
God-level code editing software (SublimeText3)
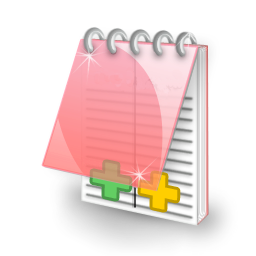
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
