


How to implement real-time data synchronization and backup functions in PHP
How to implement real-time synchronization and backup of data in PHP
In web application development, real-time synchronization and backup of data are very important, especially In scenarios involving key information such as user data and transaction records. As a popular server-side scripting language, PHP can achieve real-time synchronization and backup of data through some technical means. This article will introduce a method based on database triggers and scheduled tasks to implement this function, and provide corresponding code examples.
1. Database triggers to achieve data synchronization
A database trigger is a special object that can be defined in the database. It can automatically trigger some operations under specific conditions, such as in a certain Execute a predetermined code when an insert, update, or delete operation occurs on a table. Using database triggers, we can achieve real-time synchronization of data.
The following is a simple example, assuming we have two database tables: table1 and table2. When data in table1 is inserted, updated, or deleted, we need to automatically synchronize the corresponding operations to table2.
First, we create a trigger in the database:
CREATE TRIGGER sync_trigger AFTER INSERT ON table1 FOR EACH ROW BEGIN INSERT INTO table2 (col1, col2, col3) VALUES (NEW.col1, NEW.col2, NEW.col3); END;
The above code indicates that when an insert operation is performed in table1, the inserted data will be synchronized to table2.
Through the above method, we can define corresponding triggers for different operations (insert, update, delete) to achieve real-time synchronization of data.
2. Scheduled tasks to implement data backup
In addition to data synchronization, data backup is also very important to prevent data loss. In PHP, we can use scheduled tasks to implement the data backup function.
In Linux systems, we can use the crontab command to set up scheduled tasks. The following is an example, assuming that we need to automatically back up the data of database table table1 to a file at 3 am every day.
First, edit the scheduled task file:
crontab -e
Then, add the following content to the opened file:
0 3 * * * mysqldump -u username -p password database_name table1 > /path/to/backup/file.sql
The above code indicates that executing the mysqldump command at 3 a.m. every day will The data of table1 is backed up to the specified file.
By setting appropriate scheduled tasks, the function of regularly automatically backing up database tables can be achieved.
3. Complete code example
The following is a complete PHP code example, which combines the functions of database triggers and scheduled tasks to achieve real-time synchronization and backup of data:
<?php // 设置数据库连接信息 $host = 'localhost'; $username = 'root'; $password = 'password'; $dbname = 'database_name'; // 创建数据库连接 $conn = new mysqli($host, $username, $password, $dbname); // 设置触发器 $trigger_sql = " CREATE TRIGGER sync_trigger AFTER INSERT ON table1 FOR EACH ROW BEGIN INSERT INTO table2 (col1, col2, col3) VALUES (NEW.col1, NEW.col2, NEW.col3); END; "; $conn->query($trigger_sql); // 数据备份函数 function backupData() { $backup_file = '/path/to/backup/file.sql'; $cmd = "mysqldump -u $username -p $password $dbname table1 > $backup_file"; exec($cmd); } // 设置定时任务 $cron_job = "0 3 * * * php /path/to/backup-script.php"; shell_exec("crontab -l | { cat; echo "$cron_job"; } | crontab -"); // 关闭数据库连接 $conn->close(); ?>
In the above code, we first implement the real-time synchronization function of data by creating a trigger. Then, we defined a backup function backupData()
and set up a scheduled task to call this function to implement data backup.
It should be noted that the above code is a simple example and may need to be appropriately modified and optimized according to specific needs in actual scenarios.
Summary:
Real-time synchronization and backup of data in PHP can be achieved through database triggers and scheduled tasks. Database triggers can trigger corresponding operations under specific conditions to achieve data synchronization. Scheduled tasks can execute backup functions regularly to achieve data backup. Through these methods, we can protect the security and reliability of critical data and ensure that data can be quickly restored in unexpected situations.
The above is the detailed content of How to implement real-time data synchronization and backup functions in PHP. For more information, please follow other related articles on the PHP Chinese website!
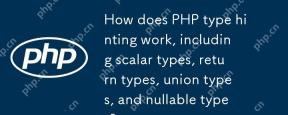
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
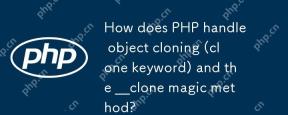
In PHP, use the clone keyword to create a copy of the object and customize the cloning behavior through the \_\_clone magic method. 1. Use the clone keyword to make a shallow copy, cloning the object's properties but not the object's properties. 2. The \_\_clone method can deeply copy nested objects to avoid shallow copying problems. 3. Pay attention to avoid circular references and performance problems in cloning, and optimize cloning operations to improve efficiency.
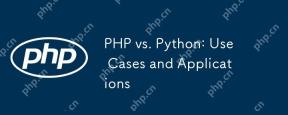
PHP is suitable for web development and content management systems, and Python is suitable for data science, machine learning and automation scripts. 1.PHP performs well in building fast and scalable websites and applications and is commonly used in CMS such as WordPress. 2. Python has performed outstandingly in the fields of data science and machine learning, with rich libraries such as NumPy and TensorFlow.
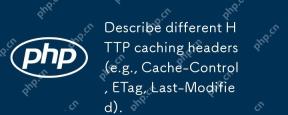
Key players in HTTP cache headers include Cache-Control, ETag, and Last-Modified. 1.Cache-Control is used to control caching policies. Example: Cache-Control:max-age=3600,public. 2. ETag verifies resource changes through unique identifiers, example: ETag: "686897696a7c876b7e". 3.Last-Modified indicates the resource's last modification time, example: Last-Modified:Wed,21Oct201507:28:00GMT.
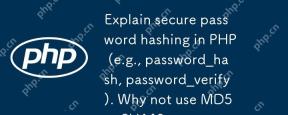
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
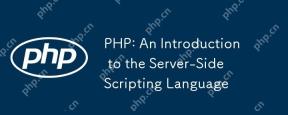
PHP is a server-side scripting language used for dynamic web development and server-side applications. 1.PHP is an interpreted language that does not require compilation and is suitable for rapid development. 2. PHP code is embedded in HTML, making it easy to develop web pages. 3. PHP processes server-side logic, generates HTML output, and supports user interaction and data processing. 4. PHP can interact with the database, process form submission, and execute server-side tasks.
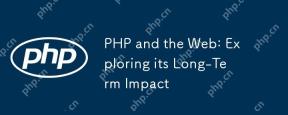
PHP has shaped the network over the past few decades and will continue to play an important role in web development. 1) PHP originated in 1994 and has become the first choice for developers due to its ease of use and seamless integration with MySQL. 2) Its core functions include generating dynamic content and integrating with the database, allowing the website to be updated in real time and displayed in personalized manner. 3) The wide application and ecosystem of PHP have driven its long-term impact, but it also faces version updates and security challenges. 4) Performance improvements in recent years, such as the release of PHP7, enable it to compete with modern languages. 5) In the future, PHP needs to deal with new challenges such as containerization and microservices, but its flexibility and active community make it adaptable.
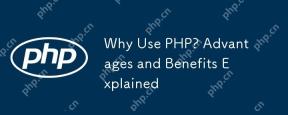
The core benefits of PHP include ease of learning, strong web development support, rich libraries and frameworks, high performance and scalability, cross-platform compatibility, and cost-effectiveness. 1) Easy to learn and use, suitable for beginners; 2) Good integration with web servers and supports multiple databases; 3) Have powerful frameworks such as Laravel; 4) High performance can be achieved through optimization; 5) Support multiple operating systems; 6) Open source to reduce development costs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
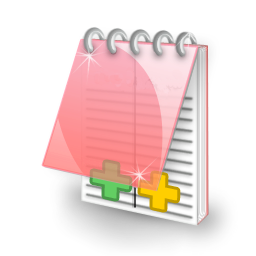
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 Linux new version
SublimeText3 Linux latest version

Zend Studio 13.0.1
Powerful PHP integrated development environment