How to use PHP to implement a simple message board version 2.0
With the rapid development of the Internet, message boards have become an important part of many websites. Message boards not only provide a platform for users to interact with the website, but also help website administrators understand users' real-time feedback and opinions. In this article, we will introduce how to use PHP to implement a simple message board version 2.0, including the functions of publishing, displaying and deleting messages.
1. Preparation
Before you start, make sure you have installed PHP and a MySQL database server. At the same time, you also need to create a new database on your web server and create a user to access this database.
2. Create a database table
First, create a table named "messages" in your MySQL database to store message information. This table can contain the following fields:
- id: the unique identifier of the message, using an auto-incrementing primary key.
- name: The name of the person who left the message.
- email: The email address of the person who left the message.
- content: The content of the message.
- created_at: The creation time of the message, using MySQL's "timestamp" type.
3. Create a message board page
Create a file named "index.php" and write the following HTML code:
<!DOCTYPE html> <html> <head> <title>留言板</title> </head> <body> <h1 id="欢迎来到留言板">欢迎来到留言板</h1> <h2 id="发布留言">发布留言</h2> <form action="post_message.php" method="post"> <label for="name">姓名:</label> <input type="text" name="name" id="name"><br> <label for="email">邮箱:</label> <input type="email" name="email" id="email"><br> <label for="content">内容:</label><br> <textarea name="content" id="content" rows="5" cols="50"></textarea><br> <input type="submit" value="发布留言"> </form> <h2 id="留言列表">留言列表</h2> <?php // 在这里编写PHP代码用于显示留言列表 ?> </body> </html>
4. Process the posting of messages
Create a file named "post_message.php" and write the following PHP code:
<?php // 连接到数据库 $conn = new mysqli("localhost", "username", "password", "database"); // 检查连接是否成功 if ($conn->connect_error) { die("连接数据库失败: " . $conn->connect_error); } // 检查是否有通过POST请求提交的留言 if ($_SERVER["REQUEST_METHOD"] == "POST") { $name = $_POST["name"]; $email = $_POST["email"]; $content = $_POST["content"]; // 准备SQL语句,并将留言插入到数据库中 $sql = "INSERT INTO messages (name, email, content) VALUES ('$name', '$email', '$content')"; if ($conn->query($sql) === TRUE) { echo "留言发布成功"; header("Location: index.php"); } else { echo "出现错误: " . $conn->error; } } // 关闭与数据库的连接 $conn->close(); ?>
5. Display the message list
Add the following PHP code in the "index.php" file , used to display the message list:
<?php // 连接到数据库 $conn = new mysqli("localhost", "username", "password", "database"); // 检查连接是否成功 if ($conn->connect_error) { die("连接数据库失败: " . $conn->connect_error); } // 查询数据库中的留言列表 $sql = "SELECT * FROM messages"; $result = $conn->query($sql); if ($result->num_rows > 0) { while ($row = $result->fetch_assoc()) { echo "<p>姓名:" . $row["name"] . "</p>"; echo "<p>邮箱:" . $row["email"] . "</p>"; echo "<p>内容:" . $row["content"] . "</p>"; echo "<hr>"; } } else { echo "暂时没有留言"; } // 关闭与数据库的连接 $conn->close(); ?>
6. Deletion of messages
Add the following PHP code in the "index.php" file to realize the deletion function of messages:
<?php // 连接到数据库 $conn = new mysqli("localhost", "username", "password", "database"); // 检查连接是否成功 if ($conn->connect_error) { die("连接数据库失败: " . $conn->connect_error); } // 检查是否有通过GET请求提交的删除操作 if ($_SERVER["REQUEST_METHOD"] == "GET" && isset($_GET["delete"])) { $deleteId = $_GET["delete"]; // 准备SQL语句,并从数据库中删除指定的留言 $sql = "DELETE FROM messages WHERE id = $deleteId"; if ($conn->query($sql) === TRUE) { echo "留言删除成功"; header("Location: index.php"); } else { echo "出现错误: " . $conn->error; } } // 关闭与数据库的连接 $conn->close(); ?>
Now, you can try to access the "index.php" file to test the functions of publishing, displaying and deleting messages.
Summary:
Through the introduction of this article, you have learned to use PHP to implement a simple message board version 2.0. You can further customize and expand according to your own needs, such as adding editing functions, user login functions, etc. Hope this article can be helpful to you!
The above is the detailed content of How to implement a simple message board version 2.0 using PHP. For more information, please follow other related articles on the PHP Chinese website!
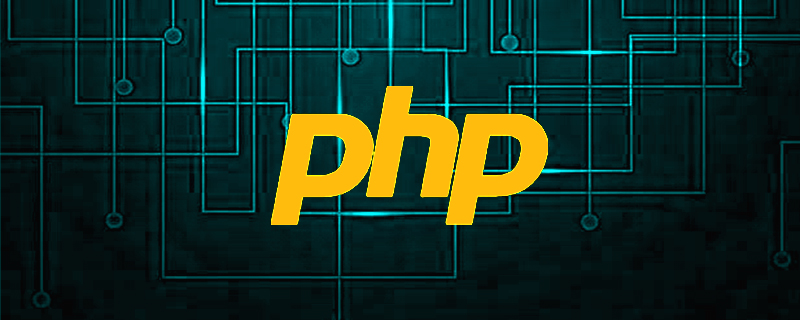
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
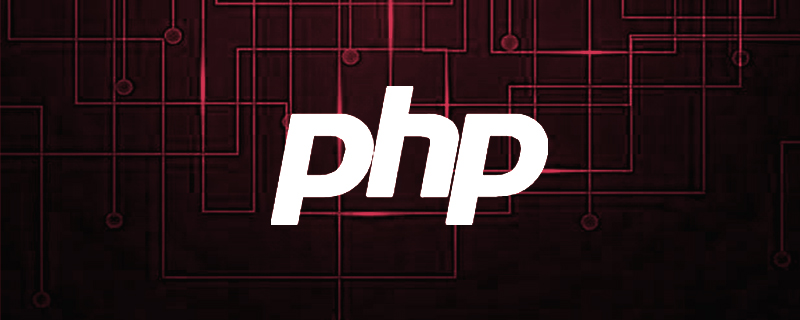
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
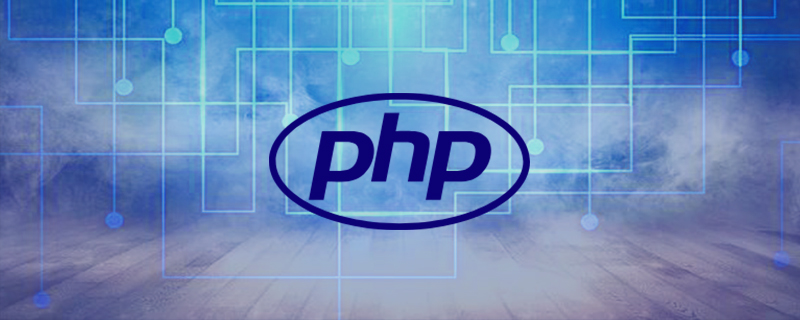
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
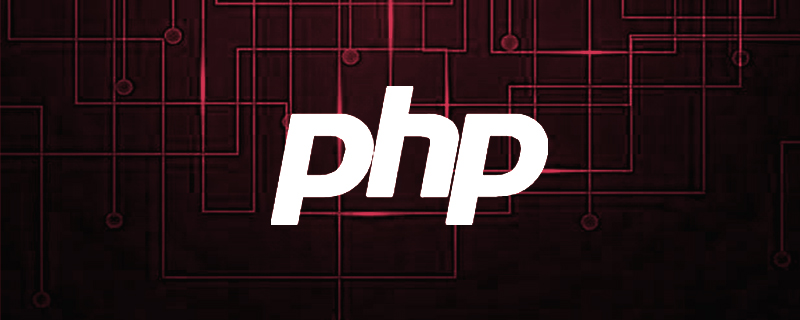
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
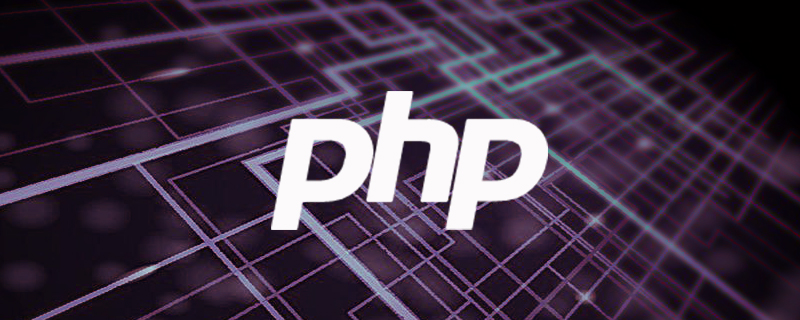
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
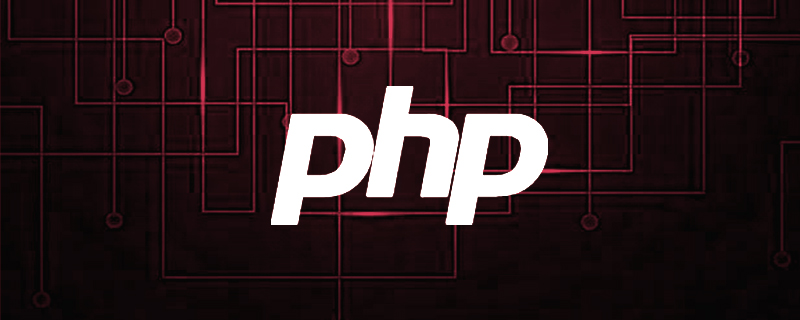
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
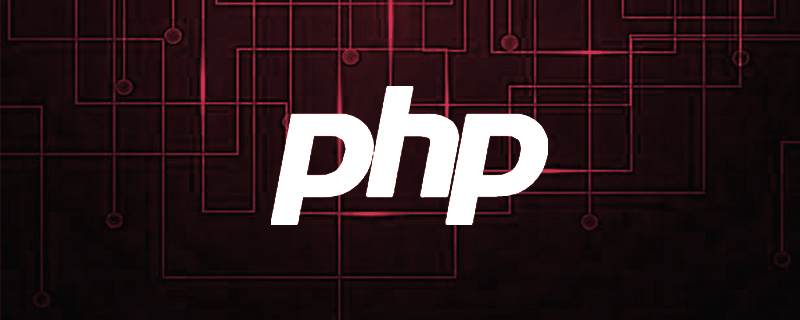
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。
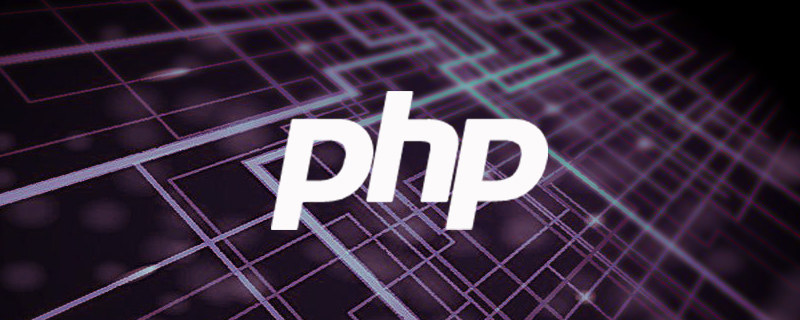
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
