How to use PHP to develop a simple online submission system
Introduction:
With the popularity and development of the Internet, more and more people are willing to use online Share your creations by submitting articles to get wider reading and feedback. This article will introduce how to use PHP to develop a simple online submission system to facilitate users to submit articles and manage submission content.
1. Design the database structure:
Before we begin, we need to design the database structure to store data related to submissions. Here, we can create a table named "articles". The structure contains the following fields:
- id: The unique identifier of the article, using an auto-incrementing primary key.
- title: The title of the article, using VARCHAR type, limiting the character length.
- content: The content of the article, using the TEXT type, used to store large text data.
- author: The author of the article, using VARCHAR type.
- submit_date: The submission date of the article, using the DATETIME type.
2. Create a database connection:
In the PHP code, we need to first create a connection to the database. You can create a database connection through the following code:
<?php $servername = "localhost"; $username = "root"; $password = ""; $dbname = "article_system"; // 创建连接 $conn = new mysqli($servername, $username, $password, $dbname); // 检测连接是否成功 if ($conn->connect_error) { die("连接失败: " . $conn->connect_error); } ?>
3. Create a submission page:
Next, we need to create a submission page so that users can fill in the article title and content and submit their submission. You can use the following code to create the HTML code of the submission page:
<!DOCTYPE html> <html> <head> <title>在线投稿系统</title> </head> <body> <h1 id="欢迎使用在线投稿系统">欢迎使用在线投稿系统</h1> <form action="submit_article.php" method="POST"> <label for="title">标题:</label> <input type="text" name="title" required><br> <label for="content">内容:</label><br> <textarea name="content" rows="10" cols="50" required></textarea><br> <input type="submit" value="提交"> </form> </body> </html>
4. Processing form submission:
In the submission page, we need to submit the title and content filled in by the user to the server for processing, and store the data Save into database. In order to implement this function, we can create a file named "submit_article.php" and write the following code in it:
<?php $servername = "localhost"; $username = "root"; $password = ""; $dbname = "article_system"; // 创建连接 $conn = new mysqli($servername, $username, $password, $dbname); // 检测连接是否成功 if ($conn->connect_error) { die("连接失败: " . $conn->connect_error); } // 处理表单提交 $title = $_POST['title']; $content = $_POST['content']; $author = "匿名作者"; $submit_date = date("Y-m-d H:i:s"); $sql = "INSERT INTO articles (title, content, author, submit_date) VALUES ('$title', '$content', '$author', '$submit_date')"; if ($conn->query($sql) === TRUE) { echo "投稿成功!"; } else { echo "投稿失败:" . $conn->error; } $conn->close(); ?>
5. Display the submission list:
In order to facilitate users to view the submitted contributions For articles, we can create a submission list page to display the submission content stored in the database. You can use the following code to create a submission list page:
<!DOCTYPE html> <html> <head> <title>投稿列表</title> </head> <body> <h1 id="投稿列表">投稿列表</h1> <?php $servername = "localhost"; $username = "root"; $password = ""; $dbname = "article_system"; // 创建连接 $conn = new mysqli($servername, $username, $password, $dbname); // 检测连接是否成功 if ($conn->connect_error) { die("连接失败: " . $conn->connect_error); } // 查询数据库数据 $sql = "SELECT * FROM articles ORDER BY submit_date DESC"; $result = $conn->query($sql); if ($result->num_rows > 0) { while($row = $result->fetch_assoc()) { echo "<h2 id="row-title">" . $row["title"] . "</h2>"; echo "<p>" . $row["content"] . "</p>"; echo "<p>作者:" . $row["author"] . "</p>"; echo "<p>提交日期:" . $row["submit_date"] . "</p>"; echo "<hr>"; } } else { echo "暂无投稿"; } $conn->close(); ?> </body> </html>
6. Summary:
Through the above steps, we have successfully developed a simple online submission system using PHP. Users can fill in the title and content of the article on the submission page. After clicking the submit button, the relevant information of the article will be saved into the database. In addition, we have also created a submission list page to display the list of submitted articles. This system can facilitate users to submit contributions, and manage and display the submission content.
Summarizing the above steps, we can see that it is not difficult to develop a simple online submission system using PHP. By designing the database structure, creating database connections, writing form processing scripts and displaying list pages, we can implement a basic submission system. Of course, depending on the needs, we can further add functions, such as user registration and login, comment functions, etc., to improve the user experience and the practicality of the system. I hope this article can be helpful to you, and I wish you successful development!
The above is the detailed content of How to use PHP to develop a simple online submission system. For more information, please follow other related articles on the PHP Chinese website!
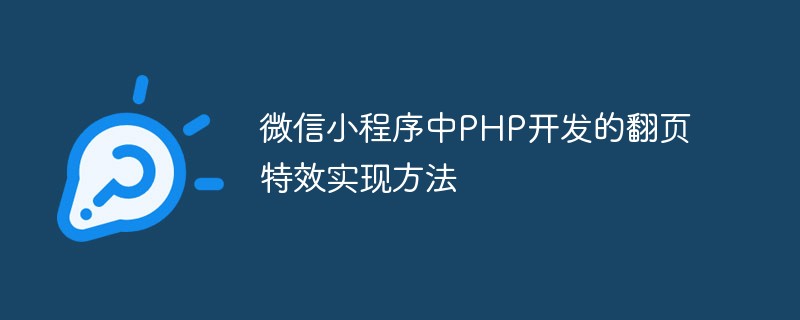
在微信小程序中,PHP开发的翻页特效是非常常见的功能。通过这种特效,用户可以轻松地在不同的页面之间进行切换,浏览更多的内容。在本文中,我们将介绍如何使用PHP来实现微信小程序中的翻页特效。我们将会讲解一些基本的PHP知识和技巧,以及一些实际的代码示例。理解基本的PHP语言知识在PHP中,我们经常会用到IF/ELSE语句、循环结构,以及函数等一些基本语言知识。
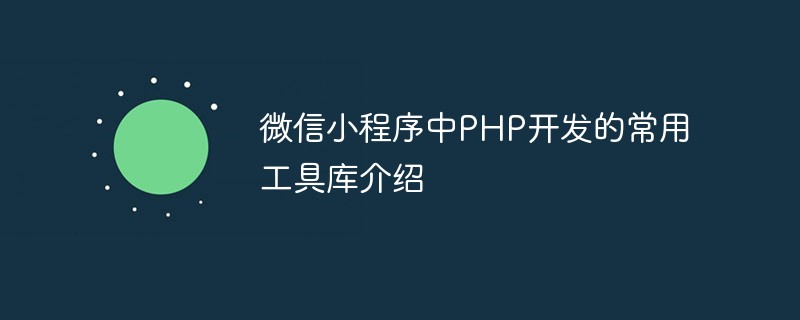
随着微信小程序的普及和发展,越来越多的开发者开始涉足其中。而PHP作为一种后端技术的代表,也在小程序中得到了广泛的运用。在小程序的开发中,PHP常用工具库也是很重要的一个部分。本文将介绍几款比较实用的PHP常用工具库,供大家参考。一、EasyWeChatEasyWeChat是一个开源的微信开发工具库,用于快速开发微信应用。它提供了一些常用的微信接口,如微信公
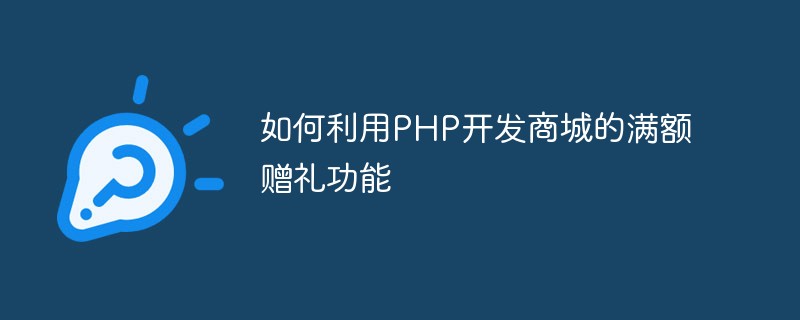
网上购物已经成为人们日常生活中不可或缺的一部分,因此,越来越多的企业开始关注电商领域。开发一款实用、易用的商城网站也成为了企业提高销售额、拓展市场的必要手段之一。在商城网站中,满额赠礼功能是提高用户购买欲望和促进销售增长的重要功能之一。本文将探讨如何利用PHP开发商城的满额赠礼功能。一、满额赠礼功能的实现思路在商城开发中,如何实现满额赠礼功能呢?简单来说就是
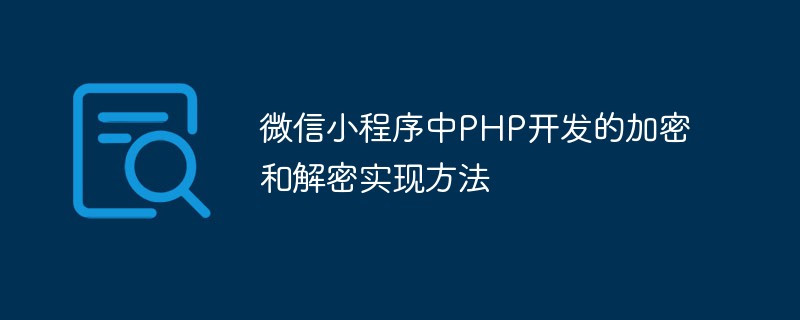
随着微信小程序在移动应用市场中越来越流行,它的开发也受到越来越多的关注。在小程序中,PHP作为一种常用的后端语言,经常用于处理敏感数据的加密和解密。本文将介绍在微信小程序中如何使用PHP实现加密和解密。一、什么是加密和解密?加密是将敏感数据转换为不可读的形式,以确保数据在传输过程中不被窃取或篡改。解密是将加密数据还原为原始数据。在小程序中,加密和解密通常包括
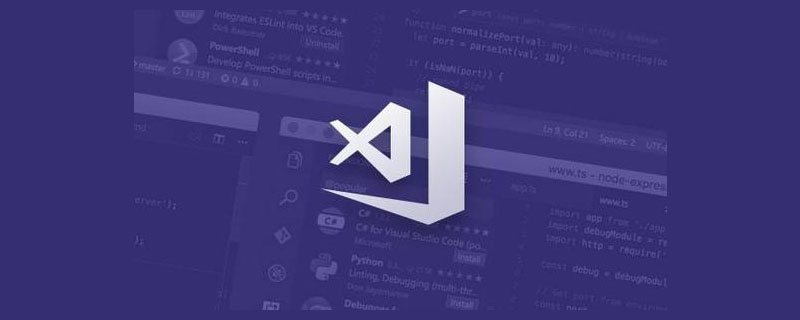
本篇文章给大家推荐一些VSCode+PHP开发中实用的插件。有一定的参考价值,有需要的朋友可以参考一下,希望对大家有所帮助。
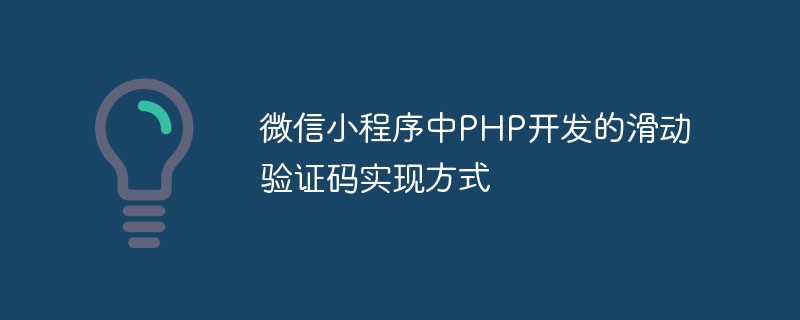
随着互联网的快速发展,网络安全问题也变得越来越严峻。针对恶意攻击、刷单等安全威胁,很多网站和应用程序都使用了验证码来保护用户信息和系统安全。在微信小程序中,如何实现一个安全可靠的滑动验证码呢?本文将介绍使用PHP开发的滑动验证码实现方式。一、滑动验证码的原理滑动验证码是指在验证用户身份时,通过用户在滑块上滑动完成验证过程。其原理是将一张图片分成两部分,一部分
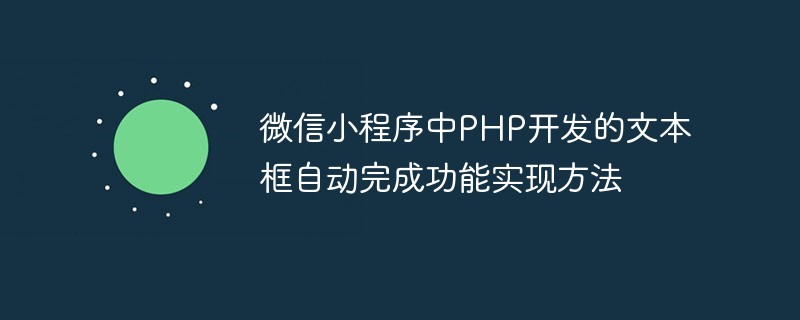
随着微信小程序的普及,各类开发需求也日渐增多。其中,文本框自动完成功能是小程序中常用的功能之一。虽然微信小程序提供了一些原生的组件,但是有一些特殊需求还是需要进行二次开发。本文将介绍如何使用PHP语言实现微信小程序中文本框自动完成功能。准备工作在开始开发之前,需要准备一些基本的环境和工具。首先,需要安装好PHP环境。其次,需要在微信小程序后台获取到自己的Ap
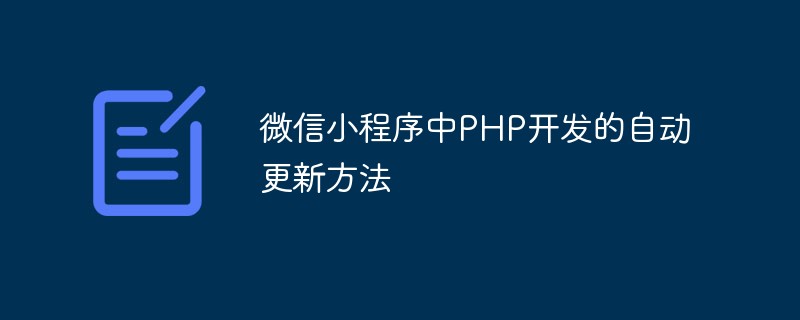
近年来,移动互联网的快速发展和移动终端的普及,让微信应用程序成为了人们生活中不可或缺的一部分。而在微信应用程序中,小程序更是以其轻量、快速、便捷的特点受到了广泛的欢迎。但是,对于小程序中的数据更新问题,却成为了一个比较头疼的问题。为了解决这一问题,我们可以使用PHP开发的自动更新方法来实现自动化数据更新。本篇文章就来探讨一下微信小程序中PHP开发的自动更新方


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
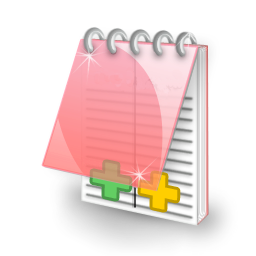
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
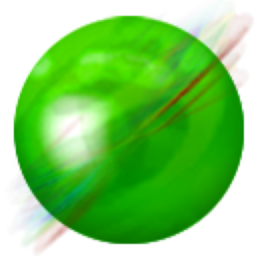
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
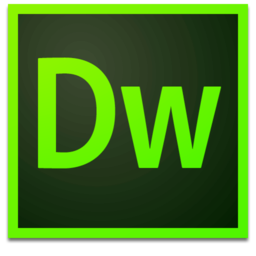
Dreamweaver Mac version
Visual web development tools
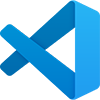
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
