


How to implement regular and automatic updates of test papers in online answering questions requires specific code examples
In modern society, with the development of the Internet, more and more exams and tests have moved online. In order to ensure the fairness and accuracy of the exam, regular and automatic updates of test papers have become important functions in the online answering system. This article will introduce how to implement regular and automatic updates of test papers in the online answering system, and give specific code examples.
1. Ideas for regular updating of test papers
The purpose of regular updating of test papers is to maintain the freshness and diversity of test questions and to prevent candidates from cheating by knowing the content of the test questions in advance. There are several implementation ideas for regular updating of test papers:
1. Test question bank update: Create a test question bank that contains various types of questions. Every once in a while, a part of the questions in the test question bank will be randomly selected to form a new test paper. This not only ensures the diversity of the test papers, but also achieves regular updates of the test papers.
2. Random selection of questions: Set a certain weight in the question bank, and randomly select questions based on the weight to form a test paper. The weight can be determined based on factors such as the difficulty and importance of the test questions to ensure that the test paper is balanced and moderately difficult.
3. Test paper tampering detection: After the test paper is generated, tampering detection is performed on the test questions to ensure that the content of the test questions has not been modified. Detection can be done by calculating the hash value or digital signature of the test question.
2. Code example for regular update of test papers
The following is a simple code example that demonstrates how to implement regular update of test papers:
import random # 试题库 questions = [ { 'id': 1, 'content': '题目1', 'difficulty': 2, 'subject': '数学', }, { 'id': 2, 'content': '题目2', 'difficulty': 3, 'subject': '数学', }, { 'id': 3, 'content': '题目3', 'difficulty': 1, 'subject': '英语', }, # 其他题目... ] def generate_paper(num_questions): # 随机抽取题目形成试卷 paper = random.sample(questions, num_questions) return paper # 每周更新试卷 def update_paper(): # 每周需要更新的题目数量 num_questions = 5 paper = generate_paper(num_questions) return paper # 主程序 def main(): # 生成试卷 paper = update_paper() # 打印试卷内容 for question in paper: print(question['content']) if __name__ == '__main__': main()
In the above code example,questions
is the test question bank, which contains all question information. The generate_paper
function implements the function of randomly selecting questions to form a test paper. The update_paper
function is responsible for updating the test papers every week, where num_questions
represents the number of questions that need to be updated every week. Finally, call the update_paper
function in the main
function to generate the test paper. After running the code, the generated test paper content will be printed.
3. Implementation ideas for automatic update of test papers
In order to realize automatic update of test papers, you can consider the following implementation ideas:
1. Scheduled tasks: Use the scheduled task framework (such as celery) Set up periodic tasks and update test papers regularly. The execution interval of tasks can be set according to specific needs.
2. Version control: Set the version number in the test question bank, and update the version number every time the test question is updated. The online question answering system checks the version number of the test question bank before each exam. If a new version is found, the test paper will be automatically updated.
3. API interface: Use the API interface to connect the test question bank to the online answering system to achieve real-time synchronization and updating of test questions. The online answering system calls the API interface to obtain the latest test questions before each answer.
The above are some common implementation ideas for automatic update of test papers. The specific implementation method can be selected according to specific needs and system architecture.
To sum up, regular and automatic updating of test papers is one of the essential functions in the online answering system. Developers can choose the appropriate implementation method based on specific needs and system requirements, and develop with specific code examples. The regular updating of test papers and the implementation of the automatic updating function not only improve the fairness and accuracy of the exam, but also increase the candidate's answering experience and participation.
The above is the detailed content of How to implement regular and automatic updates of test papers in online answering questions. For more information, please follow other related articles on the PHP Chinese website!
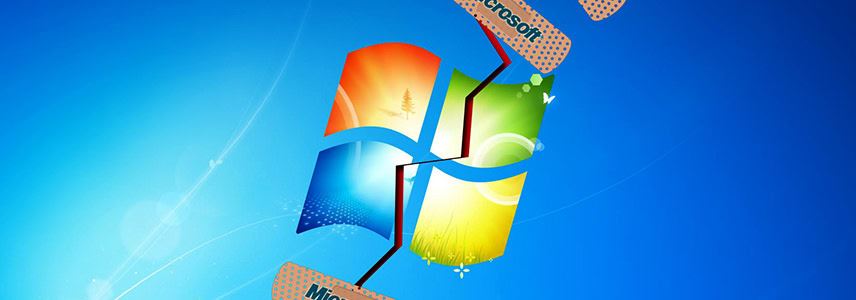
大家在系统上连接任何新的硬件设备时Windows都会自动尝试为其安装驱动程序,在系统内置的驱动包无法识别时还会自动尝试连接到WindowsUpdate去搜索和安装驱动。Windows也可以在无需用户交互的情况下,通过WindowsUpdate自动更新设备的驱动程序。这个功能看起来虽然方便,但在特定情况下,自动更新驱动这一特性反而会给用户造成麻烦。例如,用户的视频工作流如DaVinciResolve、AdobePremiere等需要用某个特定老版本的NvidiaStudio驱动,结果Windows
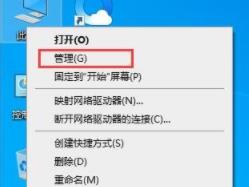
很多用户在日常使用电脑的时候总是会接收到系统的自动更新,不仅让电脑变慢还变卡,为此我们今天给大家带来了win11不想自动更新操作方法,如果自动更新一直影响你就来看看怎么关闭吧。windows11系统不想自动更新怎么弄1、首先右击桌面“此电脑”然后选择“管理”。2、在打开的“计算机管理”中,按依次点开“服务”→“应用程序”→“服务”→“Windowsupdate”。3、接下来双击“Windowsupdate”,将“启动类型”设置为“禁用”,点击“停止”服务并确定。4、点击“恢复”选项卡,将第一次失
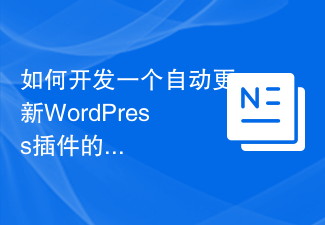
如何开发一个自动更新WordPress插件的功能WordPress是一个非常流行的开源内容管理系统(CMS),拥有丰富的插件市场来扩展其功能。为了确保插件始终保持最新和安全,开发者需要实现自动更新功能。在本文中,我们将介绍如何开发一个自动更新WordPress插件的功能,并提供代码示例来帮助您迅速上手。准备工作在开始开发之前,您需要准备以下几个关键的步骤:创
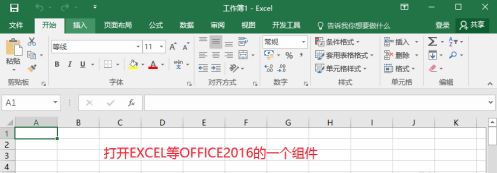
很多人在办公中都在使用office2016下载,但是你们知道office2016下载怎样关闭自动更新吗?下文小编就讲述了office2016下载关闭自动更新的方法,感兴趣的用户快来下文看看吧。先打开一个组件,比如可以打开Office2016的EXCEL电子表格软件,其它组件也是可以的。点击界面左上角的文件菜单,如图所示操作。在左侧这里找到账户选项打开进入,开始进行操作。点击更新选项中的禁用更新按钮。当Office更新这里显示为无法更新此产品的时候,说明我们设置完成。当我们以后需要更新的时候,我们
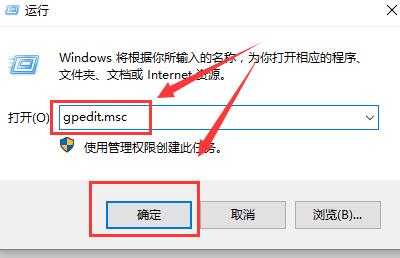
在使用win10系统的时候,我们都经常会遇到更新的问题,这个更新有时候很烦人,那么怎么才能解决这个问题呢,这个需要我们进入到本地组策略里面去设置。win10组策略关闭自动更新方法:1、按下键盘上的“win+R”打开运行,输入“gpedit.msc”点击确定。2、选择“管理模板”-“Windows组件”,双击进入3、然后找到“Windows更新”,双击进入。4、在右边找到“配置自动更新”,鼠标双击打开。5、然后勾选“已禁用”,然后点击“应用”,就行了。
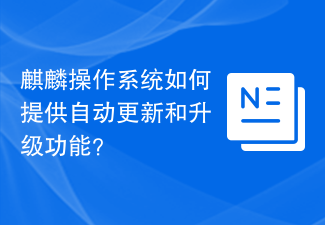
麒麟操作系统如何提供自动更新和升级功能?随着科技的不断进步和操作系统的日益重要,用户对于操作系统的稳定性、安全性和功能的要求也越来越高。为了满足用户的需求,操作系统需要提供自动更新和升级功能,及时修复漏洞和添加新功能。麒麟操作系统作为国产自主研发的操作系统,也不例外,它提供了自动更新和升级的功能。在麒麟操作系统中,自动更新和升级的功能主要通过软件包管理器和更
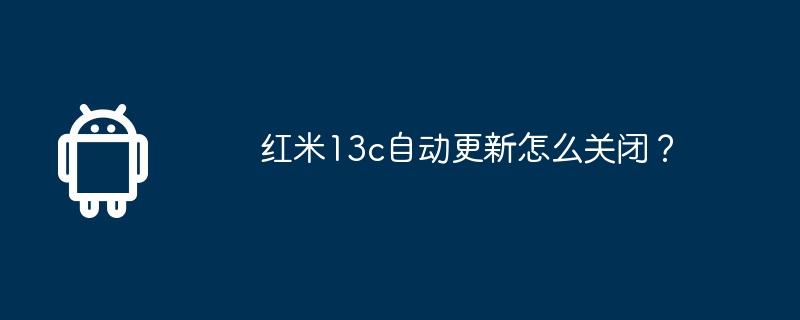
现在的手机是越来越强大的,功能越来越全面,也为用户们提供更为舒适的使用体验,Redmi13C作为最新发布的新机型,各种功能也是很多的,而且还为用户们带来了很多新的设计,那么红米13c怎么关闭自动更新呢?下面就让本站小编来为大家简单介绍功能吧,大家有需要的话可以来一起看看哦。红米13c怎么关闭自动更新?1.打开手机设置,点击我的设备。2.点击MIUI版本。3.点击右上角的三个点。4.点击系统更新设置。5.将自动下载和智能更新后面的开关关闭即可。关闭自动更新还是有必要了解一下的,以上这些就是红米13
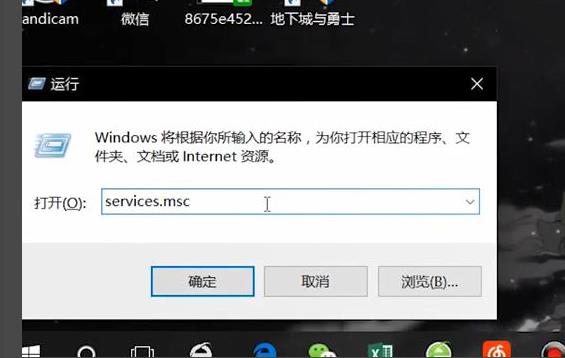
很多用户们在使用电脑的时候,有时候出现了故障只能在安全模式下操作,但是安全模式一直自动更新,带来了不必要的麻烦,其实关闭的方法也不是很难只要禁用一下就好了。win10安全模式怎么取消自动更新:1、按下键盘“Win+R”在运行框内输入“services.msc”点击“确定”2、在弹出的服务中找到“WindowsUpdate”3、把“启动类型”改为禁用,然后点击“应用”即可


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
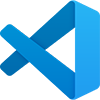
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
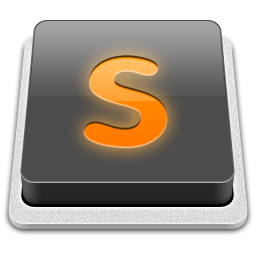
SublimeText3 Mac version
God-level code editing software (SublimeText3)
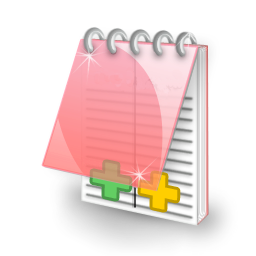
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
