


How to handle bill splitting and merging in accounting systems - Methods to implement bill splitting and merging using PHP
How to deal with bill splitting and merging in accounting systems - Methods of using PHP to implement bill splitting and merging
In modern business operations, accounting systems It is one of the must-have tools for every business. In the accounting system, we often encounter situations that require the splitting and merging of bills. This article will introduce how to use PHP to implement bill splitting and merging, and provide specific code examples.
1. Bill splitting method
Bill splitting refers to splitting a bill into multiple sub-bills, and each sub-bill contains part of the original bill. Split bills usually occur in the following situations:
- When accounting, you need to split an expense or income into multiple different categories or items.
- Within an enterprise, a bill needs to be split by department or person.
- Business trip expense reimbursement requires splitting a total expense into multiple different expense items.
Implementing bill splitting in PHP can be achieved through data models and algorithms. The following is a sample code:
<?php class Bill { private $id; private $amount; private $categories; public function __construct($id, $amount) { $this->id = $id; $this->amount = $amount; $this->categories = []; // 初始化为空数组 } public function addCategory($category, $percentage) { $this->categories[$category] = $percentage; } public function splitBill() { $subBills = []; foreach ($this->categories as $category => $percentage) { $subBillAmount = $this->amount * $percentage / 100; $subBills[$category] = new Bill($this->id, $subBillAmount); } return $subBills; } } // 示例用法 $originalBill = new Bill(1, 100); $originalBill->addCategory('餐饮费', 50); // 拆分成50%的餐饮费 $originalBill->addCategory('交通费', 30); // 拆分成30%的交通费 $originalBill->addCategory('住宿费', 20); // 拆分成20%的住宿费 $subBills = $originalBill->splitBill(); print_r($subBills); ?>
In the above example, we defined a Bill
class, which contains attributes such as the bill's ID, amount (amount), and categories (categories). addCategory()
The method is used to add bill categories and corresponding percentages. splitBill()
The method splits according to the classification percentage and returns the split sub-bills.
In this way, we can complete the bill splitting by instantiating the Bill
object and calling the corresponding method.
2. Method of bill consolidation
Bill consolidation refers to merging multiple bills into one total bill. Consolidated bills usually occur in the following situations:
- Merge multiple split sub-bills into one overall bill.
- Merge multiple bills of the same category for statistics and analysis.
Implementing bill consolidation in PHP can also be achieved through data models and algorithms. The following is a sample code:
<?php class Bill { private $id; private $amount; public function __construct($id, $amount) { $this->id = $id; $this->amount = $amount; } public function mergeBill($bill) { $this->amount += $bill->amount; // 合并账单金额 } } // 示例用法 $mainBill = new Bill(1, 100); $subBill1 = new Bill(2, 50); $subBill2 = new Bill(3, 30); $subBill3 = new Bill(4, 20); $mainBill->mergeBill($subBill1); $mainBill->mergeBill($subBill2); $mainBill->mergeBill($subBill3); echo $mainBill->amount; ?>
In the above example, we also define a Bill
class, which contains the ID and amount attributes of the bill. mergeBill()
The method is used to merge the incoming bill object into the main bill and update the total amount.
By instantiating the Bill
object, we can call the mergeBill()
method to merge the sub-bills into the main bill, and finally get the combined total bill amount.
Summary:
Bill splitting and merging in the accounting system is a common operation and an important means to improve accounting efficiency and accuracy. This article introduces the method of bill splitting and merging through example code using PHP language. In practical applications, corresponding adjustments and optimizations can be made according to specific needs and business logic to achieve more accurate and efficient bill processing.
The above is the detailed content of How to handle bill splitting and merging in accounting systems - Methods to implement bill splitting and merging using PHP. For more information, please follow other related articles on the PHP Chinese website!
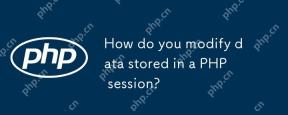
TomodifydatainaPHPsession,startthesessionwithsession_start(),thenuse$_SESSIONtoset,modify,orremovevariables.1)Startthesession.2)Setormodifysessionvariablesusing$_SESSION.3)Removevariableswithunset().4)Clearallvariableswithsession_unset().5)Destroythe
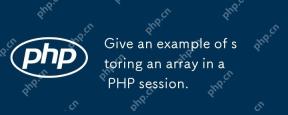
Arrays can be stored in PHP sessions. 1. Start the session and use session_start(). 2. Create an array and store it in $_SESSION. 3. Retrieve the array through $_SESSION. 4. Optimize session data to improve performance.
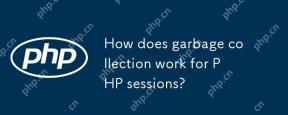
PHP session garbage collection is triggered through a probability mechanism to clean up expired session data. 1) Set the trigger probability and session life cycle in the configuration file; 2) You can use cron tasks to optimize high-load applications; 3) You need to balance the garbage collection frequency and performance to avoid data loss.
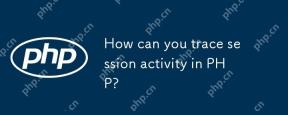
Tracking user session activities in PHP is implemented through session management. 1) Use session_start() to start the session. 2) Store and access data through the $_SESSION array. 3) Call session_destroy() to end the session. Session tracking is used for user behavior analysis, security monitoring, and performance optimization.
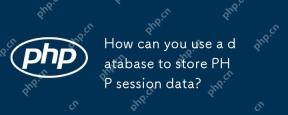
Using databases to store PHP session data can improve performance and scalability. 1) Configure MySQL to store session data: Set up the session processor in php.ini or PHP code. 2) Implement custom session processor: define open, close, read, write and other functions to interact with the database. 3) Optimization and best practices: Use indexing, caching, data compression and distributed storage to improve performance.
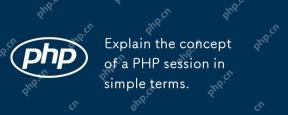
PHPsessionstrackuserdataacrossmultiplepagerequestsusingauniqueIDstoredinacookie.Here'showtomanagethemeffectively:1)Startasessionwithsession_start()andstoredatain$_SESSION.2)RegeneratethesessionIDafterloginwithsession_regenerate_id(true)topreventsessi
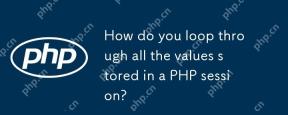
In PHP, iterating through session data can be achieved through the following steps: 1. Start the session using session_start(). 2. Iterate through foreach loop through all key-value pairs in the $_SESSION array. 3. When processing complex data structures, use is_array() or is_object() functions and use print_r() to output detailed information. 4. When optimizing traversal, paging can be used to avoid processing large amounts of data at one time. This will help you manage and use PHP session data more efficiently in your actual project.
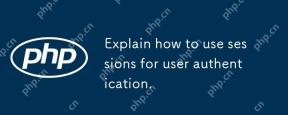
The session realizes user authentication through the server-side state management mechanism. 1) Session creation and generation of unique IDs, 2) IDs are passed through cookies, 3) Server stores and accesses session data through IDs, 4) User authentication and status management are realized, improving application security and user experience.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
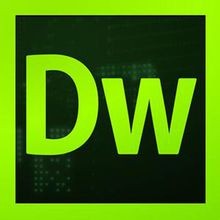
Dreamweaver CS6
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
