


Using PHP and Vue to implement automatic increase of member points after payment
Title: How to use PHP and Vue to automatically increase member points after payment
Introduction:
With the popularity of e-commerce, more and more Businesses began to use membership systems to attract and retain customers. As a common member benefit, the points system can motivate customers to spend and increase their loyalty. In this article, we will introduce how to use PHP and Vue to automatically increase member points after payment, and provide specific code examples.
1. Create database and data table
First, we need to create a data table in the database to store member information and points. We can use the following SQL statement to create a data table named members
:
CREATE TABLE members ( id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(50) NOT NULL, points INT DEFAULT 0 );
2. PHP backend code implementation
Next, we need to write PHP backend code to process Member points will be automatically increased after successful payment. We can use the following code as a reference:
// 连接数据库 $host = 'localhost'; $dbName = 'your_db_name'; $username = 'your_username'; $password = 'your_password'; $conn = new PDO("mysql:host=$host;dbname=$dbName;charset=utf8", $username, $password); // 从请求中获取会员ID和支付金额 $memberId = $_POST['member_id']; $amount = $_POST['amount']; // 根据会员ID查询当前积分 $stmt = $conn->prepare("SELECT points FROM members WHERE id = :id"); $stmt->bindParam(':id', $memberId); $stmt->execute(); $points = $stmt->fetch(PDO::FETCH_ASSOC)['points']; // 更新积分 $newPoints = $points + $amount; $stmt = $conn->prepare("UPDATE members SET points = :points WHERE id = :id"); $stmt->bindParam(':id', $memberId); $stmt->bindParam(':points', $newPoints); $stmt->execute(); // 返回更新后的积分 $response = [ 'status' => 'success', 'points' => $newPoints ]; echo json_encode($response);
3. Vue front-end code implementation
Finally, we need to use Vue to write the front-end code to handle the automatic increase of member points after successful payment. We can use the following code as a reference:
<template> <div> <button @click="handlePayment">支付</button> <p>当前积分:{{ points }}</p> </div> </template> <script> export default { data() { return { memberID: 1, amount: 100, points: 0 }; }, methods: { handlePayment() { // 发送支付请求到后端 axios.post('/api/payment', { member_id: this.memberID, amount: this.amount }).then(response => { // 更新积分 this.points = response.data.points; }).catch(error => { console.error(error); }); } }, mounted() { // 页面加载时获取当前积分 axios.get(`/api/members/${this.memberID}`) .then(response => { this.points = response.data.points; }).catch(error => { console.error(error); }); } }; </script>
Conclusion:
The method of using PHP and Vue to automatically increase member points after payment is not complicated. Through the above PHP back-end code and Vue front-end code examples, we can easily implement the function of automatically increasing points after payment in the membership system. Such an implementation can not only motivate customers to consume, but also improve their loyalty, thereby increasing the company's revenue and market competitiveness. Please make appropriate modifications and configurations according to the actual situation to meet project needs.
The above is the detailed content of Using PHP and Vue to implement automatic increase of member points after payment. For more information, please follow other related articles on the PHP Chinese website!
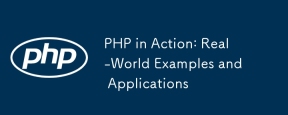
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
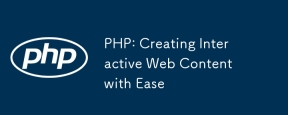
PHP makes it easy to create interactive web content. 1) Dynamically generate content by embedding HTML and display it in real time based on user input or database data. 2) Process form submission and generate dynamic output to ensure that htmlspecialchars is used to prevent XSS. 3) Use MySQL to create a user registration system, and use password_hash and preprocessing statements to enhance security. Mastering these techniques will improve the efficiency of web development.
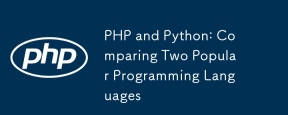
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
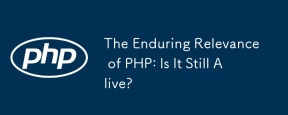
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
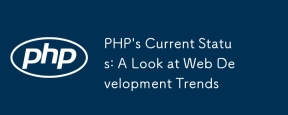
PHP remains important in modern web development, especially in content management and e-commerce platforms. 1) PHP has a rich ecosystem and strong framework support, such as Laravel and Symfony. 2) Performance optimization can be achieved through OPcache and Nginx. 3) PHP8.0 introduces JIT compiler to improve performance. 4) Cloud-native applications are deployed through Docker and Kubernetes to improve flexibility and scalability.
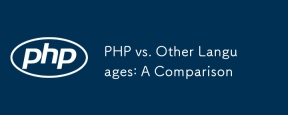
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
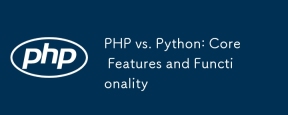
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.
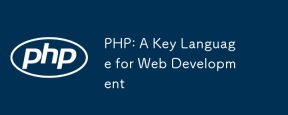
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
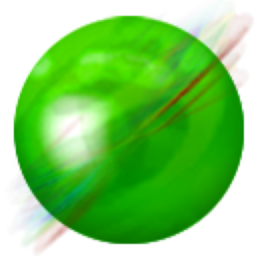
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
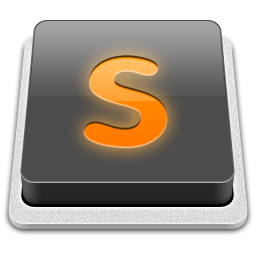
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.