


In this article, we delve into a fascinating problem of string manipulation and character encoding in computer science. The current task is to minimize the number of swaps between identically indexed characters of two strings such that the sum of the ASCII values of the characters in the two strings is an odd number. We solve this problem using C, a powerful and versatile programming language favored by many software developers.
Understanding ASCII
ASCII is the abbreviation of American Standard Code for Information Interchange and is a character encoding standard for electronic communications. ASCII codes represent text in computers, telecommunications equipment, and other devices that use text.
Problem Statement
We have two strings of equal length. The goal is to perform the minimum exchange of characters at the same position in both strings so that the sum of the ASCII values of the characters in each string is an odd number.
solution
Calculate ASCII sum − Calculate the sum of ASCII values for each string. Then, check if the sum is even or odd.
Determine exchange requirements − If the sum is already odd, no exchange is required. If the sum is even, a swap is required.
Find matching swaps − Find characters in two strings whose swapping would produce an odd sum. Track the number of exchanges.
Return results− Return the minimum number of exchanges required.
Example
This is the modified code suitable for all scenarios -
#include <bits/stdc++.h> using namespace std; int minSwaps(string str1, string str2) { int len = str1.length(); int ascii_sum1 = 0, ascii_sum2 = 0; for (int i = 0; i < len; i++) { ascii_sum1 += str1[i]; ascii_sum2 += str2[i]; } // If total sum is odd, it's impossible to have both sums odd if ((ascii_sum1 + ascii_sum2) % 2 != 0) return -1; // If both sums are odd already, no swaps are needed if (ascii_sum1 % 2 != 0 && ascii_sum2 % 2 != 0) return 0; // If both sums are even, we just need to make one of them odd if (ascii_sum1 % 2 == 0 && ascii_sum2 % 2 == 0) { for (int i = 0; i < len; i++) { // If we find an odd character in str1 and an even character in str2, or vice versa, swap them if ((str1[i] - '0') % 2 != (str2[i] - '0') % 2) return 1; } } // If we reach here, it means no eligible swaps were found return -1; } int main() { string str1 = "abc"; string str2 = "def"; int result = minSwaps(str1, str2); if(result == -1) { cout << "No valid swaps found.\n"; } else { cout << "Minimum swaps required: " << result << endl; } return 0; }
Output
No valid swaps found.
illustrate
Consider two strings -
str1 = "abc", str2 = "def"
We calculate the ASCII sum of str1 (294: a = 97, b = 98, c = 99) and str2 (303: d = 100, e = 101, f = 102). The ASCII sum is 597, which is an odd number. Therefore, it is impossible for both sums to be odd and the program will output "No valid swaps find".
The solution uses simple programming structures and logical reasoning to effectively solve the problem.
in conclusion
Minimizing swapping to obtain odd sums of ASCII values is an interesting problem that enhances our understanding of string manipulation, character encoding, and problem-solving skills. The solutions provided use the C programming language and demonstrate how to handle different scenarios in the problem statement.
One thing to note is that this solution assumes that both strings are the same length. If you don't do this, you'll need additional logic to handle this situation.
The above is the detailed content of Minimize the number of swaps of characters with the same index so that the sum of the ASCII values of the characters in the two strings is an odd number. For more information, please follow other related articles on the PHP Chinese website!
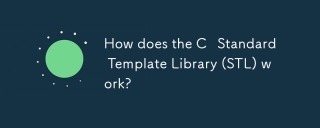
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
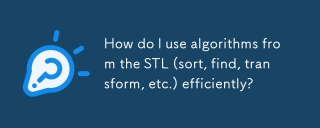
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
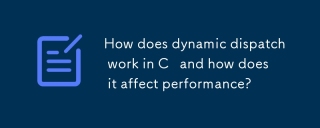
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
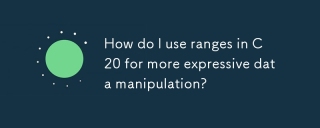
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
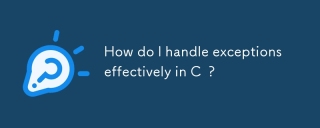
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
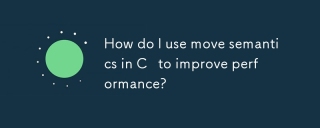
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
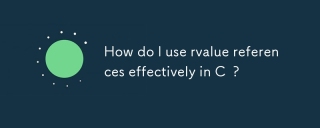
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
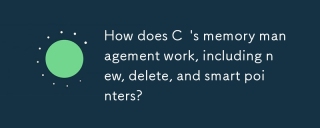
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
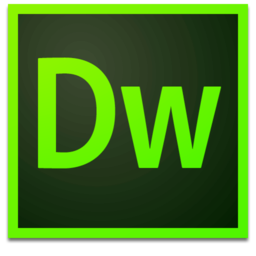
Dreamweaver Mac version
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor
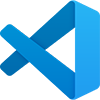
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Chinese version
Chinese version, very easy to use
