Golang Development: Best Practices for Implementing Parallel Computing
Golang development: Best practices for implementing parallel computing, specific code examples are required
Overview:
Parallel computing is a method widely used in computer science Technology that improves program performance and efficiency by performing multiple tasks simultaneously. Golang is a programming language that supports concurrent programming. It has built-in rich concurrency primitives and library functions, making the implementation of parallel computing simpler and more efficient. This article will introduce some best practices for implementing parallel computing in Golang and give specific code examples.
The concept of parallel computing:
Parallel computing refers to a computing method in which multiple computing tasks run simultaneously within the same time period. In contrast, serial computing means that each computing task can only start execution after the previous task is completed. The advantage of parallel computing is that it can make fuller use of computing resources and improve processing power and efficiency.
Parallel computing in Golang:
Golang is an open source programming language with a concise and easy-to-read syntax and powerful concurrent programming capabilities. Golang implements concurrency through Goroutine and Channel mechanisms, which makes the implementation of parallel computing relatively simple.
The following are some best practice examples for implementing parallel computing:
- Use coroutines to implement parallel computing tasks:
func computeTask(id int, ch chan int) { // 任务具体逻辑 // ... // 将结果发送到通道 ch <- result } func main() { // 创建一个用于接收结果的通道 resultCh := make(chan int) // 启动多个协程执行计算任务 for i := 0; i < numTasks; i++ { go computeTask(i, resultCh) } // 接收并处理结果 for i := 0; i < numTasks; i++ { result := <-resultCh // 处理结果 } }
- Use WaitGroup Wait for all coroutines to complete computing tasks:
func computeTask(id int, wg *sync.WaitGroup, results []int) { // 任务具体逻辑 // ... // 存储结果到共享的切片 results[id] = result // 通知WaitGroup任务完成 wg.Done() } func main() { var wg sync.WaitGroup // 初始化共享结果切片 results := make([]int, numTasks) // 增加WaitGroup的计数值 wg.Add(numTasks) // 启动多个协程执行计算任务 for i := 0; i < numTasks; i++ { go computeTask(i, &wg, results) } // 等待所有协程执行完成 wg.Wait() // 处理结果 for _, result := range results { // 处理结果 } }
- Use concurrency-safe locks to protect shared resources:
var mutex sync.Mutex func computeTask(id int, results *[]int) { // 任务具体逻辑 // ... // 使用锁保护共享资源 mutex.Lock() (*results)[id] = result mutex.Unlock() } func main() { // 初始化共享结果切片 results := make([]int, numTasks) // 启动多个协程执行计算任务 for i := 0; i < numTasks; i++ { go computeTask(i, &results) } // 等待所有协程执行完成 time.Sleep(time.Second) // 处理结果 for _, result := range results { // 处理结果 } }
Summary:
By using Golang’s coroutines With the process and channel mechanism, we can easily implement parallel computing tasks. Parallel computing can make full use of computing resources and improve program performance and efficiency. When implementing parallel computing, attention needs to be paid to ensuring the concurrency security of shared resources, which can be achieved by using locks or other concurrency control mechanisms.
I hope that through the introduction of this article, readers will have an understanding of the best practices for implementing parallel computing in Golang, and can flexibly apply them in actual development.
The above is the detailed content of Golang Development: Best Practices for Implementing Parallel Computing. For more information, please follow other related articles on the PHP Chinese website!
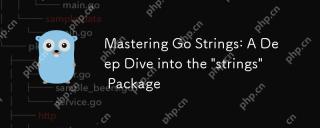
You should care about the "strings" package in Go because it provides tools for handling text data, splicing from basic strings to advanced regular expression matching. 1) The "strings" package provides efficient string operations, such as Join functions used to splice strings to avoid performance problems. 2) It contains advanced functions, such as the ContainsAny function, to check whether a string contains a specific character set. 3) The Replace function is used to replace substrings in a string, and attention should be paid to the replacement order and case sensitivity. 4) The Split function can split strings according to the separator and is often used for regular expression processing. 5) Performance needs to be considered when using, such as
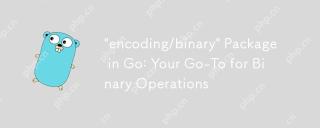
The"encoding/binary"packageinGoisessentialforhandlingbinarydata,offeringtoolsforreadingandwritingbinarydataefficiently.1)Itsupportsbothlittle-endianandbig-endianbyteorders,crucialforcross-systemcompatibility.2)Thepackageallowsworkingwithcus
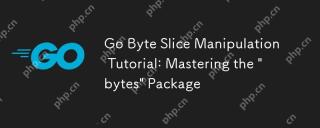
Mastering the bytes package in Go can help improve the efficiency and elegance of your code. 1) The bytes package is crucial for parsing binary data, processing network protocols, and memory management. 2) Use bytes.Buffer to gradually build byte slices. 3) The bytes package provides the functions of searching, replacing and segmenting byte slices. 4) The bytes.Reader type is suitable for reading data from byte slices, especially in I/O operations. 5) The bytes package works in collaboration with Go's garbage collector, improving the efficiency of big data processing.
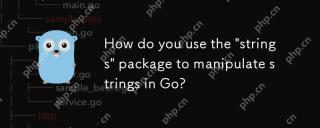
You can use the "strings" package in Go to manipulate strings. 1) Use strings.TrimSpace to remove whitespace characters at both ends of the string. 2) Use strings.Split to split the string into slices according to the specified delimiter. 3) Merge string slices into one string through strings.Join. 4) Use strings.Contains to check whether the string contains a specific substring. 5) Use strings.ReplaceAll to perform global replacement. Pay attention to performance and potential pitfalls when using it.
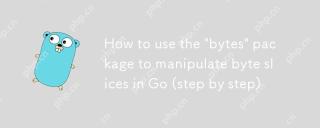
ThebytespackageinGoishighlyeffectiveforbyteslicemanipulation,offeringfunctionsforsearching,splitting,joining,andbuffering.1)Usebytes.Containstosearchforbytesequences.2)bytes.Splithelpsbreakdownbyteslicesusingdelimiters.3)bytes.Joinreconstructsbytesli
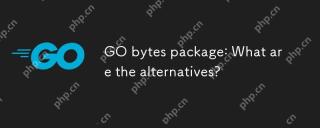
ThealternativestoGo'sbytespackageincludethestringspackage,bufiopackage,andcustomstructs.1)Thestringspackagecanbeusedforbytemanipulationbyconvertingbytestostringsandback.2)Thebufiopackageisidealforhandlinglargestreamsofbytedataefficiently.3)Customstru
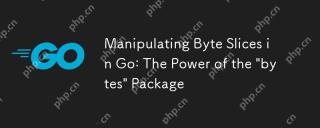
The"bytes"packageinGoisessentialforefficientlymanipulatingbyteslices,crucialforbinarydata,networkprotocols,andfileI/O.ItoffersfunctionslikeIndexforsearching,Bufferforhandlinglargedatasets,Readerforsimulatingstreamreading,andJoinforefficient
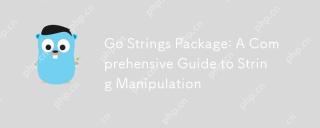
Go'sstringspackageiscrucialforefficientstringmanipulation,offeringtoolslikestrings.Split(),strings.Join(),strings.ReplaceAll(),andstrings.Contains().1)strings.Split()dividesastringintosubstrings;2)strings.Join()combinesslicesintoastring;3)strings.Rep


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
