How to use the binary search algorithm in C
The binary search algorithm (Binary Search) is an efficient search algorithm that divides an ordered data set into Both halves search in the middle position of the data set each time. By comparing the value in the middle position with the target value, the search range is continuously narrowed until the target value is found or the target value is determined not to exist. The following will introduce how to use the binary search algorithm in C and give specific code examples.
- Determine the search scope
Before using the binary search algorithm, you first need to make sure that the data set to be searched is ordered. For example, we have an ordered array of integers nums in which we want to search for a certain target value target. - Define binary search function
In C, we can define a function to implement the binary search algorithm. The input parameters of this function include the array to be searched, the starting and ending positions of the array, and the target value target. The return value of the function is the index of the target value in the array. If the target value does not exist, a specific value (such as -1) can be returned.
The specific function definition is as follows:
int binarySearch(int nums[], int start, int end, int target) { // 定义二分搜索的起始位置和结束位置 int left = start; int right = end; while (left <= right) { // 计算中间位置 int mid = left + (right - left) / 2; // 如果中间位置的值等于目标值,直接返回索引 if (nums[mid] == target) { return mid; } // 如果中间位置的值大于目标值,更新结束位置 else if (nums[mid] > target) { right = mid - 1; } // 如果中间位置的值小于目标值,更新起始位置 else { left = mid + 1; } } // 目标值不存在,返回-1 return -1; }
- Calling the binary search function
By calling the binary search function, we can get the index of the target value in the array. For example, we have an ordered array nums and we want to search for the target value target. You can use the following code to call the binary search function:
int nums[] = {1, 3, 5, 7, 9}; int n = sizeof(nums) / sizeof(nums[0]); int target = 5; int index = binarySearch(nums, 0, n - 1, target); if (index != -1) { cout << "目标值的索引为:" << index << endl; } else { cout << "目标值不存在!" << endl; }
In the above code, we first define an ordered array nums, and then calculate the length n of the array. Then the target value target is defined, and the binary search function binarySearch is called to search the index of the target value. Finally, the output is based on the result returned by the function.
Through the above steps, we can use the binary search algorithm in C to perform efficient search operations. In actual applications, the binary search function can be called according to specific scenarios and requirements, and further processing can be performed based on the returned results.
Summary
The binary search algorithm is an efficient search algorithm suitable for ordered data collections. In C, we can search by defining a binary search function and passing in the array to be searched, the starting position, the ending position and the target value. By continuously updating the search range, the index of the target value can eventually be found. We hope that the introduction and code examples in this article can help readers better understand and apply the binary search algorithm.
The above is the detailed content of How to use binary search algorithm in C++. For more information, please follow other related articles on the PHP Chinese website!
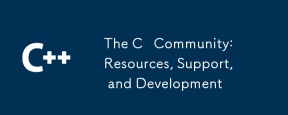
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
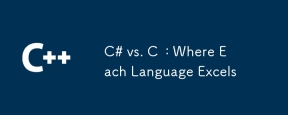
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
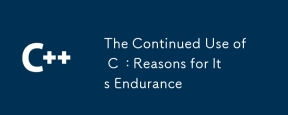
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
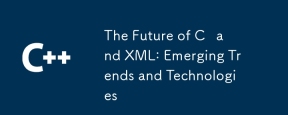
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
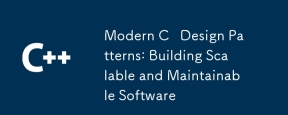
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
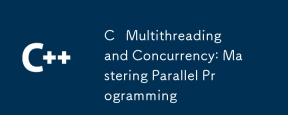
C The core concepts of multithreading and concurrent programming include thread creation and management, synchronization and mutual exclusion, conditional variables, thread pooling, asynchronous programming, common errors and debugging techniques, and performance optimization and best practices. 1) Create threads using the std::thread class. The example shows how to create and wait for the thread to complete. 2) Synchronize and mutual exclusion to use std::mutex and std::lock_guard to protect shared resources and avoid data competition. 3) Condition variables realize communication and synchronization between threads through std::condition_variable. 4) The thread pool example shows how to use the ThreadPool class to process tasks in parallel to improve efficiency. 5) Asynchronous programming uses std::as
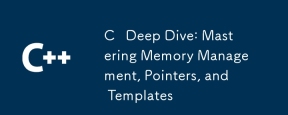
C's memory management, pointers and templates are core features. 1. Memory management manually allocates and releases memory through new and deletes, and pay attention to the difference between heap and stack. 2. Pointers allow direct operation of memory addresses, and use them with caution. Smart pointers can simplify management. 3. Template implements generic programming, improves code reusability and flexibility, and needs to understand type derivation and specialization.
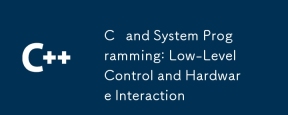
C is suitable for system programming and hardware interaction because it provides control capabilities close to hardware and powerful features of object-oriented programming. 1)C Through low-level features such as pointer, memory management and bit operation, efficient system-level operation can be achieved. 2) Hardware interaction is implemented through device drivers, and C can write these drivers to handle communication with hardware devices.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
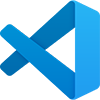
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
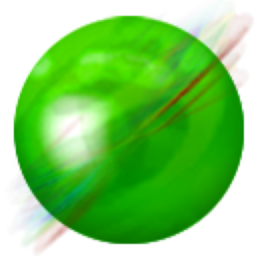
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
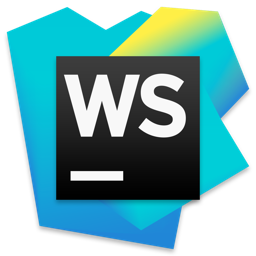
WebStorm Mac version
Useful JavaScript development tools