


How to write custom stored procedures and functions in MySQL using JavaScript
How to write custom stored procedures and functions in MySQL using JavaScript
MySQL is a popular relational database management system that provides powerful stored procedures and function functionality. Normally, we use the SQL language provided by MySQL to write stored procedures and functions. However, sometimes we may need to use JavaScript to write custom stored procedures and functions to implement more complex logic and business requirements in MySQL.
To write custom stored procedures and functions in MySQL using JavaScript, we need to use the MySQL plug-in: SpiderMonkey. SpiderMonkey is a JavaScript engine in the Mozilla project. It can be embedded into MySQL, allowing us to use JavaScript to write database logic.
Here are the steps on how to write custom stored procedures and functions using JavaScript:
Step 1: Install the SpiderMonkey plug-in
First, we need to install the SpiderMonkey plug-in in MySQL. You can download the appropriate plug-in version from MySQL's official website and install it according to the corresponding installation guide. After the installation is complete, we can enable this plug-in in the MySQL configuration file.
Step 2: Create a custom stored procedure
Let’s first look at an example written in JavaScript. This example is a custom stored procedure used to insert a row into a specified table. New record:
DELIMITER $$ CREATE PROCEDURE insert_record() BEGIN DECLARE js_code text; SET js_code = ' var conn = new MySqlConnection(); conn.connect("localhost", "user", "password", "database"); conn.query("INSERT INTO table (column1, column2) VALUES (value1, value2);"); conn.close(); '; CALL js_exec(js_code); END$$ DELIMITER ;
In this example, we first use the DELIMITER statement to modify MySQL's statement delimiter to support JavaScript syntax. Then, use the CREATE PROCEDURE statement to create a custom stored procedure named insert_record. In the declaration part of the stored procedure, we define a variable named js_code to store JavaScript code. In the main part of the stored procedure, we call a JavaScript execution function called js_exec, which can execute the JavaScript code we pass in.
Step 3: Create a custom function
In addition to stored procedures, we can also use JavaScript to write custom functions. The following is an example written in JavaScript. This example is a custom function that calculates the sum of a specified column:
DELIMITER $$ CREATE FUNCTION calculate_sum(column_name varchar(255)) RETURNS decimal(10,2) DETERMINISTIC BEGIN DECLARE js_code text; DECLARE total decimal(10,2); SET js_code = ' var conn = new MySqlConnection(); conn.connect("localhost", "user", "password", "database"); var result = conn.query("SELECT SUM(" + column_name + ") FROM table;"); total = parseFloat(result.getField(0)); conn.close(); '; SET total = 0; CALL js_exec(js_code); RETURN total; END$$ DELIMITER ;
In this example, we use the CREATE FUNCTION statement to create a custom function named calculate_sum. Define functions. The function has a parameter called column_name, which specifies the column for which the sum needs to be calculated. The function uses JavaScript code to query the sum of the specified column in the specified table and stores the result in the total variable. Finally, the js_exec function is called to execute the JavaScript code and return the calculated sum.
Summary
Using JavaScript to write custom stored procedures and functions can provide MySQL with more flexible and powerful logical processing capabilities. By using the SpiderMonkey plug-in, we can write stored procedures and functions directly in MySQL using JavaScript language. The above are the basic steps and sample code for writing custom stored procedures and functions in MySQL using JavaScript. Hope this helps!
The above is the detailed content of How to write custom stored procedures and functions in MySQL using JavaScript. For more information, please follow other related articles on the PHP Chinese website!
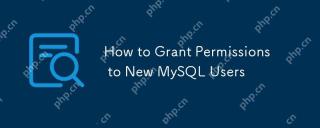
TograntpermissionstonewMySQLusers,followthesesteps:1)AccessMySQLasauserwithsufficientprivileges,2)CreateanewuserwiththeCREATEUSERcommand,3)UsetheGRANTcommandtospecifypermissionslikeSELECT,INSERT,UPDATE,orALLPRIVILEGESonspecificdatabasesortables,and4)
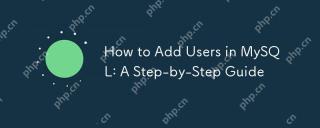
ToaddusersinMySQLeffectivelyandsecurely,followthesesteps:1)UsetheCREATEUSERstatementtoaddanewuser,specifyingthehostandastrongpassword.2)GrantnecessaryprivilegesusingtheGRANTstatement,adheringtotheprincipleofleastprivilege.3)Implementsecuritymeasuresl
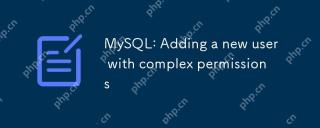
ToaddanewuserwithcomplexpermissionsinMySQL,followthesesteps:1)CreatetheuserwithCREATEUSER'newuser'@'localhost'IDENTIFIEDBY'password';.2)Grantreadaccesstoalltablesin'mydatabase'withGRANTSELECTONmydatabase.TO'newuser'@'localhost';.3)Grantwriteaccessto'
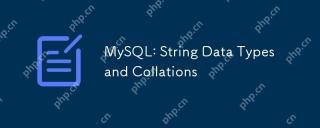
The string data types in MySQL include CHAR, VARCHAR, BINARY, VARBINARY, BLOB, and TEXT. The collations determine the comparison and sorting of strings. 1.CHAR is suitable for fixed-length strings, VARCHAR is suitable for variable-length strings. 2.BINARY and VARBINARY are used for binary data, and BLOB and TEXT are used for large object data. 3. Sorting rules such as utf8mb4_unicode_ci ignores upper and lower case and is suitable for user names; utf8mb4_bin is case sensitive and is suitable for fields that require precise comparison.
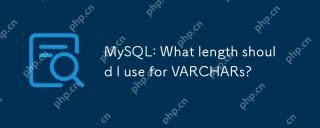
The best MySQLVARCHAR column length selection should be based on data analysis, consider future growth, evaluate performance impacts, and character set requirements. 1) Analyze the data to determine typical lengths; 2) Reserve future expansion space; 3) Pay attention to the impact of large lengths on performance; 4) Consider the impact of character sets on storage. Through these steps, the efficiency and scalability of the database can be optimized.
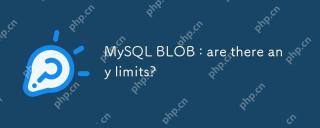
MySQLBLOBshavelimits:TINYBLOB(255bytes),BLOB(65,535bytes),MEDIUMBLOB(16,777,215bytes),andLONGBLOB(4,294,967,295bytes).TouseBLOBseffectively:1)ConsiderperformanceimpactsandstorelargeBLOBsexternally;2)Managebackupsandreplicationcarefully;3)Usepathsinst
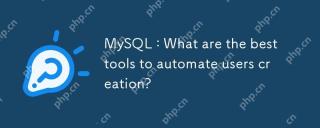
The best tools and technologies for automating the creation of users in MySQL include: 1. MySQLWorkbench, suitable for small to medium-sized environments, easy to use but high resource consumption; 2. Ansible, suitable for multi-server environments, simple but steep learning curve; 3. Custom Python scripts, flexible but need to ensure script security; 4. Puppet and Chef, suitable for large-scale environments, complex but scalable. Scale, learning curve and integration needs should be considered when choosing.
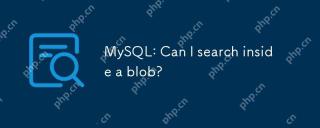
Yes,youcansearchinsideaBLOBinMySQLusingspecifictechniques.1)ConverttheBLOBtoaUTF-8stringwithCONVERTfunctionandsearchusingLIKE.2)ForcompressedBLOBs,useUNCOMPRESSbeforeconversion.3)Considerperformanceimpactsanddataencoding.4)Forcomplexdata,externalproc


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
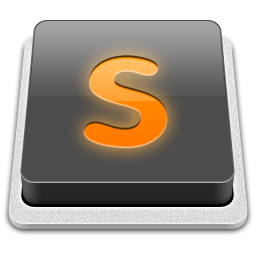
SublimeText3 Mac version
God-level code editing software (SublimeText3)

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor
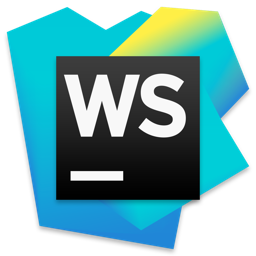
WebStorm Mac version
Useful JavaScript development tools
