Java development: How to handle distributed computing of large-scale data, specific code examples are needed
With the advent of the big data era, the need to process large-scale data has also growing day by day. In a traditional stand-alone computing environment, it is difficult to meet this demand. Therefore, distributed computing has become an important means of processing big data. Java, as a popular programming language, plays an important role in distributed computing.
In this article, we will introduce how to use Java for distributed computing of large-scale data and provide specific code examples. First, we need to build a distributed computing environment based on Hadoop. Then, we will demonstrate how to handle distributed computing of large-scale data through a simple WordCount example.
- Building a distributed computing environment (based on Hadoop)
To implement distributed computing, you first need to build a distributed computing environment. Here we choose to use Hadoop, a widely used open source distributed computing framework.
First, we need to download and install Hadoop. The latest release version can be obtained from the Hadoop official website (https://hadoop.apache.org/). After downloading, follow the instructions in the official documentation to install and configure.
After the installation is complete, we need to start the Hadoop cluster. Open the command line terminal, switch to the sbin directory of the Hadoop installation directory, and execute the following command to start the Hadoop cluster:
./start-dfs.sh // 启动HDFS ./start-yarn.sh // 启动YARN
After the startup is completed, you can view the Hadoop cluster status and http: //localhost:8088 to access the YARN resource manager.
- Example: WordCount Distributed Computing
WordCount is a classic example program used to count the number of occurrences of each word in text. Below we will use Java to perform distributed calculation of WordCount.
First, create a Java project and introduce the Hadoop jar package.
Create a WordCount class in the project and write the implementation of Map and Reduce in it.
import java.io.IOException; import org.apache.hadoop.io.IntWritable; import org.apache.hadoop.io.Text; import org.apache.hadoop.mapreduce.Mapper; import org.apache.hadoop.mapreduce.Reducer; import org.apache.hadoop.mapreduce.Job; import org.apache.hadoop.fs.Path; import org.apache.hadoop.conf.Configuration; import org.apache.hadoop.mapreduce.lib.input.FileInputFormat; import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat; public class WordCount { public static class WordCountMapper extends Mapper<Object, Text, Text, IntWritable>{ private final static IntWritable one = new IntWritable(1); private Text word = new Text(); public void map(Object key, Text value, Context context) throws IOException, InterruptedException { String[] words = value.toString().split(" "); for (String word : words) { this.word.set(word); context.write(this.word, one); } } } public static class WordCountReducer extends Reducer<Text, IntWritable, Text, IntWritable>{ private IntWritable result = new IntWritable(); public void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException { int sum = 0; for (IntWritable val : values) { sum += val.get(); } result.set(sum); context.write(key, result); } } public static void main(String[] args) throws Exception { Configuration conf = new Configuration(); Job job = Job.getInstance(conf, "word count"); job.setJarByClass(WordCount.class); job.setMapperClass(WordCountMapper.class); job.setCombinerClass(WordCountReducer.class); job.setReducerClass(WordCountReducer.class); job.setOutputKeyClass(Text.class); job.setOutputValueClass(IntWritable.class); FileInputFormat.addInputPath(job, new Path(args[0])); FileOutputFormat.setOutputPath(job, new Path(args[1])); System.exit(job.waitForCompletion(true) ? 0 : 1); } }
Next, we need to prepare the input data. Create an input directory on the Hadoop cluster and place the text files that require statistics into this directory.
Finally, we can use the following command to submit the WordCount job to run on the Hadoop cluster:
hadoop jar WordCount.jar WordCount <input-directory> <output-directory>
Replace
After the run is completed, we can view the result file in the output directory, which contains each word and its corresponding number of occurrences.
This article introduces the basic steps for distributed computing of large-scale data using Java, and provides a specific WordCount example. It is hoped that readers can better understand and apply distributed computing technology through the introduction and examples of this article, so as to process large-scale data more efficiently.
The above is the detailed content of Java development: How to handle distributed computing of large-scale data. For more information, please follow other related articles on the PHP Chinese website!
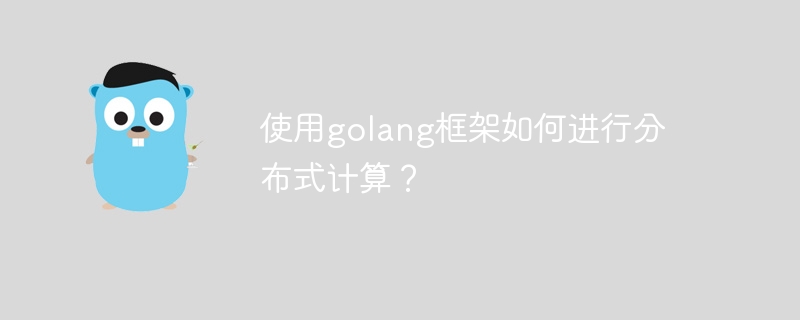
使用GoLang实现分布式计算的分步指南:安装分布式计算框架(如Celery或Luigi)创建封装任务逻辑的GoLang函数定义任务队列将任务提交到队列设置任务处理程序函数
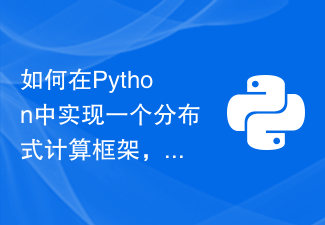
标题:Python中的分布式计算框架实现及任务调度与结果收集机制摘要:分布式计算是一个有效利用多台计算机资源来加速任务处理的方法。本文将介绍如何使用Python实现一个简单的分布式计算框架,包括任务调度和结果收集的机制与策略,并提供相关代码示例。正文:一、分布式计算框架的概述分布式计算是一种利用多台计算机共同处理任务而达到加速计算的目的。在分布式计算框架中,
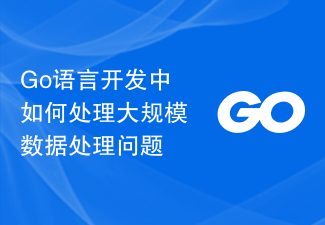
Go语言作为一门高效、并发性强的编程语言,逐渐在大规模数据处理领域得到了广泛的应用。本文将探讨在使用Go语言进行大规模数据处理时,如何处理相关的问题。首先,对于大规模数据的处理,我们需要考虑数据的输入和输出。在Go语言中,文件读写模块提供了丰富的功能,可以轻松地实现数据的读取和写入。当处理大规模数据时,我们可以选择按行读取数据,逐行进行处理,这样可以避免一次
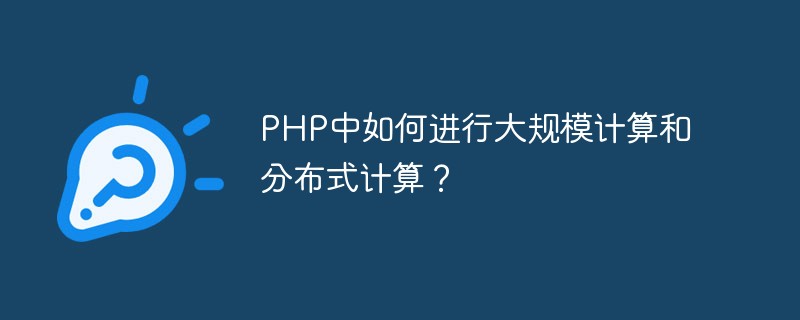
随着互联网的不断发展,Web应用程序的规模越来越大,需要处理更多的数据和更多的请求。为了满足这些需求,计算大规模数据和分布式计算成为了一个必不可少的需求。而PHP作为一门高效、易用、灵活的语言,也在不断发展和改进自身的运行方式,逐渐成为计算大规模数据和分布式计算的重要工具。本篇文章将介绍PHP中大规模计算和分布式计算的概念及实现方式。我们将讨论如何使用PHP
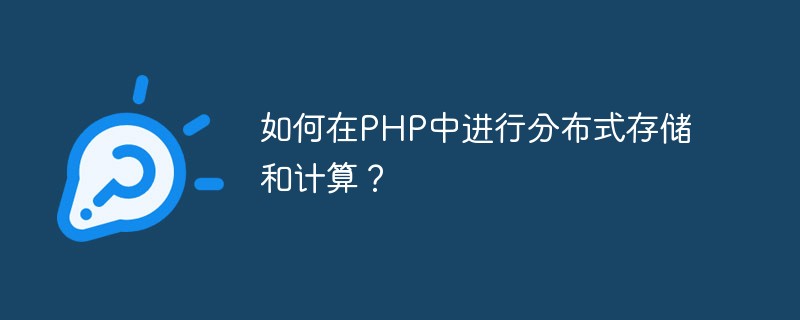
随着互联网的快速发展和数据量的急剧增加,单机存储和计算已经不能满足现代大规模数据的需求。分布式存储和计算成为解决大型数据处理的重要方法,而PHP作为一门流行的后端开发语言,则需要掌握如何在分布式环境下进行存储和计算。一、分布式存储:在分布式环境下需要将数据分散地存储在多个服务器上,并保证数据的一致性、可靠性和高可用性。以下是几种常见的分布式存储方案:HDFS
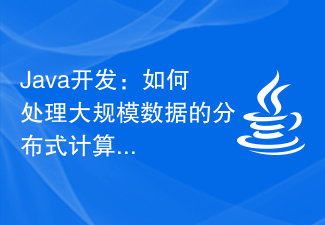
Java开发:如何处理大规模数据的分布式计算,需要具体代码示例随着大数据时代的到来,处理大规模数据的需求也日益增长。在传统的单机计算环境下,很难满足这种需求。因此,分布式计算成为了处理大数据的重要手段,其中Java作为一门流行的编程语言,在分布式计算中扮演着重要的角色。在本文中,我们将介绍如何使用Java进行大规模数据的分布式计算,并提供具体的代码示例。首先
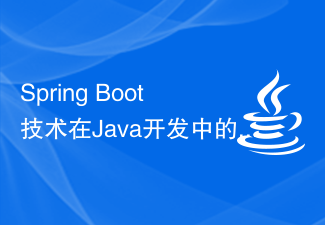
在当今软件开发领域中,Java一直处于一种主导地位。尽管Java平台已经存在了已经有二十年的历史,但它依然不断地发展与推进。近年来,一种新的框架——SpringBoot,正在Java开发中越来越受欢迎。本文将介绍SpringBoot是什么,以及它在Java开发中的应用和实践。什么是SpringBoot?SpringBoot是一种基于Spring框架的
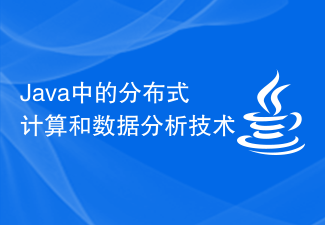
随着大数据时代的到来,数据量的爆炸式增长给传统的计算方式带来了巨大冲击。为了解决这个问题,分布式计算和数据分析技术应运而生。Java作为一种通用的编程语言,已经在分布式计算和数据分析领域表现出了良好的性能。一、分布式计算技术分布式计算是一种将计算任务分成几个子任务处理的技术,各子任务可以在不同计算机上运行,然后将它们的输出结果合并成最终结果。这种技术可以显著


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
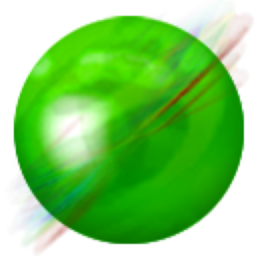
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

Notepad++7.3.1
Easy-to-use and free code editor
