How to use Java to develop a Jackson-based JSON parser
Introduction:
JSON (JavaScript Object Notation) is a lightweight data exchange format , it has become one of the commonly used data exchange formats on the Internet. In Java development, we can use the Jackson library to process JSON data. This article will introduce how to use Java to develop a Jackson-based JSON parser and provide specific code examples.
1. Import the Jackson library
Before we begin, we need to import the Jackson library first. The latest version of the Jackson library can be downloaded from the official website (https://github.com/FasterXML/jackson), or introduced using Maven or Gradle.
2. Create a Java class
Next, we can create a Java class to implement the JSON parser. For example, you can create a class called JsonParser.
import com.fasterxml.jackson.databind.ObjectMapper; public class JsonParser { public static void main(String[] args) { String json = "{"name":"John", "age":30, "city":"New York"}"; try { ObjectMapper objectMapper = new ObjectMapper(); Person person = objectMapper.readValue(json, Person.class); System.out.println("Name: " + person.getName()); System.out.println("Age: " + person.getAge()); System.out.println("City: " + person.getCity()); } catch (Exception e) { e.printStackTrace(); } } } class Person { private String name; private int age; private String city; // getters and setters }
In the above sample code, we first created a class named JsonParser and wrote the logic of JSON parsing in the main method. We used the ObjectMapper class, which is one of the core classes in the Jackson library to convert JSON strings into Java objects.
We define a Person class to receive parsed data. The Person class has three attributes: name (string type), age (integer type) and city (string type).
In the main method, we first create an ObjectMapper object and use its readValue method to parse the JSON string into a Person object. Then, we can use the getter method of the Person object to obtain the parsed data and output it to the console.
3. Run and test
After completing the above code, we can execute the program and see the console output the parsed JSON data. In this example, the output result should be:
Name: John Age: 30 City: New York
4. Extended use
In addition to the basic JSON parsing function, the Jackson library also provides rich functions, such as support for date format, customization Serialization and deserialization, etc. You can extend the parser according to your specific needs.
Summary:
This article introduces how to use Java to develop a Jackson-based JSON parser and provides specific code examples. By using the Jackson library, we can easily parse JSON strings into Java objects, thereby conveniently processing JSON data. I hope this article will be helpful when you use JSON parsers in development.
The above is the detailed content of How to develop a Jackson-based JSON parser using Java. For more information, please follow other related articles on the PHP Chinese website!
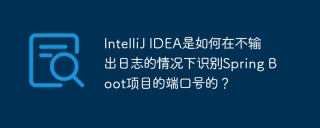
Start Spring using IntelliJIDEAUltimate version...
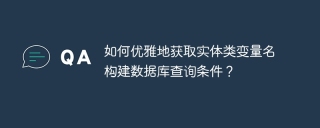
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
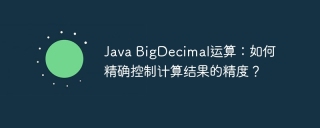
Java...
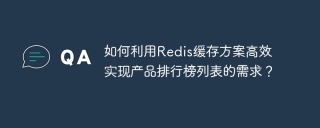
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
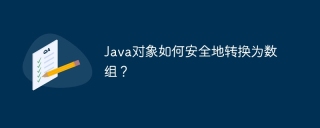
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
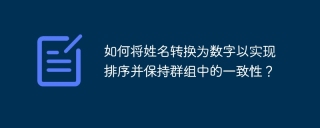
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
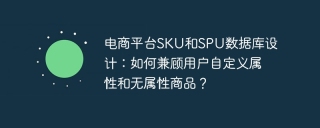
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
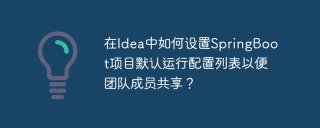
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
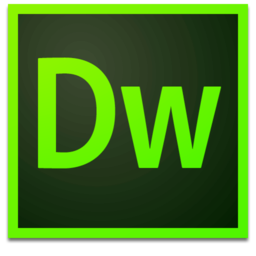
Dreamweaver Mac version
Visual web development tools
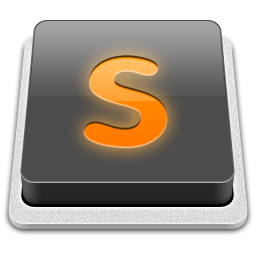
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
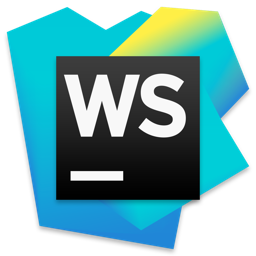
WebStorm Mac version
Useful JavaScript development tools