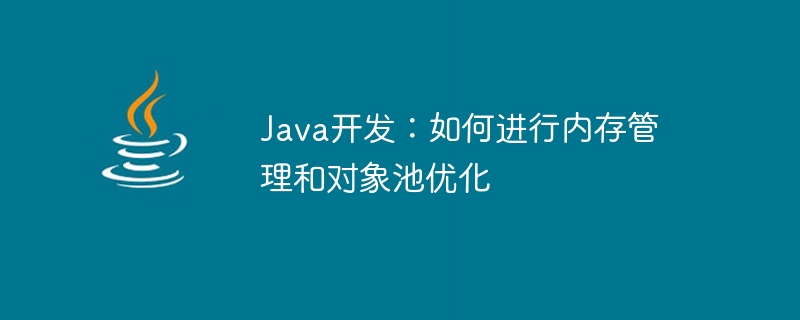
Java development: How to perform memory management and object pool optimization
Background
In Java development, memory management and object pool optimization are very important topics. Effectively managing memory and optimizing object pools can improve application performance and scalability. This article will introduce how to perform memory management and object pool optimization, and provide specific code examples.
1. Memory management
- Avoid creating too many objects
In Java, creating objects requires allocating memory space. Frequent creation and destruction of objects will lead to frequent allocation and recycling of memory, increasing the overhead of memory management. Therefore, we should try to avoid unnecessary object creation. Here are a few ways to avoid object creation:
- Use primitive types instead of wrapped types: Operations on primitive types are more efficient than wrapped types. For example, use int instead of Integer.
- Use StringBuilder or StringBuffer instead of String for string operations: This can avoid creating a large number of intermediate string objects.
- For objects in a loop, consider extracting the object outside the loop to avoid creating the object in each loop.
- Release unused objects in a timely manner
The garbage collection mechanism in Java will automatically recycle objects that are no longer used, but the triggering of the garbage collection mechanism is controlled by the JVM and Not always in a timely manner. Therefore, we can manually release objects that are no longer used to reclaim memory in a timely manner. The following are several ways to manually release objects:
- Use null assignment: When an object is no longer used, its reference can be assigned null to notify the garbage collection mechanism that the object can be recycled. The memory space of the object.
- Use the System.gc() method: Calling the gc() method of the System class can actively trigger the garbage collection mechanism.
- Using weak references and soft references
Java provides weak references and soft references to manage the life cycle of objects. Using weak references and soft references allows objects to be reclaimed by the garbage collection mechanism when memory is insufficient. The following are several scenarios for using weak references and soft references:
- Objects in the cache: For objects in the cache, soft references can be used to manage them. When there is insufficient memory, the JVM can Recycle these objects.
- Listener: For some listeners, weak references can be used to manage them. When the listening object is no longer referenced, the resources can be automatically released.
2. Object pool optimization
Object pool is a technology that reuses objects, which can reduce the cost of creating and destroying objects and improve performance and scalability.
- Implementation method of object pool
Object pool can be implemented by manually managing objects or using a third-party library. The following are several common object pool implementation methods:
- Manual implementation: Object pools can be implemented using data structures such as arrays, linked lists, or queues. When an object is needed, it is obtained from the object pool; when it is finished, the object is put back into the object pool.
- Using Apache Commons Pool: Apache Commons Pool is a very popular open source object pool library that can be used to implement object pooling.
- Application scenarios of object pool
Object pool is very suitable in the following scenarios:
- Database connection pool: for the creation of database connections And destruction is very resource-consuming. Using an object pool can avoid frequent creation and destruction of connection objects.
- Thread pool: For scenarios where threads are frequently created and destroyed, thread pools can be used to reuse thread objects and improve performance and scalability.
- Http connection pool: For scenarios with frequent Http requests, using the Http connection pool can avoid frequent creation and closing of connections.
- Object reuse: For some objects that need to be created and destroyed frequently, using an object pool can avoid frequent creation and destruction overhead.
Code Example
The following is an example of using Apache Commons Pool to implement an object pool:
class ConnectionFactory {
public Connection createConnection() {
// 创建连接
return new Connection();
}
public void closeConnection(Connection conn) {
// 关闭连接
}
}
class Connection {
// 连接对象
public void doSomething() {
// 执行操作
}
}
class ConnectionPool {
private GenericObjectPool<Connection> pool;
public ConnectionPool() {
ConnectionFactory factory = new ConnectionFactory();
pool = new GenericObjectPool<>(factory);
// 设置对象池的配置
pool.setMaxTotal(10);
pool.setMaxIdle(5);
// 其他配置...
}
public Connection getConnection() {
try {
return pool.borrowObject();
} catch (Exception e) {
// 处理异常
}
return null;
}
public void releaseConnection(Connection conn) {
try {
pool.returnObject(conn);
} catch (Exception e) {
// 处理异常
}
}
}
class Main {
public static void main(String[] args) {
ConnectionPool pool = new ConnectionPool();
Connection conn1 = pool.getConnection();
conn1.doSomething();
pool.releaseConnection(conn1);
Connection conn2 = pool.getConnection();
conn2.doSomething();
pool.releaseConnection(conn2);
// 其他操作...
}
}
This article introduces how to perform memory management and object pool optimization, and provides specific code example. Through reasonable memory management and optimized object pools, the performance and scalability of Java applications can be effectively improved. In actual development, different methods can be used to optimize the performance of memory management and object pools according to specific circumstances.
The above is the detailed content of Java development: How to perform memory management and object pool optimization. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn