How to use PHP to develop a simple online photo album function
How to use PHP to develop a simple online photo album function
Photo album is a common function for displaying pictures online. It can help us browse, share and download pictures easily . This article will introduce how to use PHP to develop a simple online photo album and provide specific code examples.
1. Build a development environment
First, we need to build a development environment. You can choose to install an integrated development environment (IDE) such as XAMPP, WAMP or MAMP, which has built-in Apache server, MySQL database and PHP interpreter.
2. Create a database
Next, we need to create a database to store the album data. You can use phpMyAdmin or other database management tools to create a new database and name it "photo_album" (or any other name you like).
In this database, we can create two tables, one for storing album information and the other for storing photo information. Here, we create two tables: albums and photos.
- albums table
The albums table is used to store basic information of the album, including fields such as album ID, album name, and creation time. The table can be created using the following SQL statement:
CREATE TABLE albums(
id INT(11) PRIMARY KEY AUTO_INCREMENT,
name VARCHAR(100) NOT NULL,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
- photos table
photos table is used to store specific photo information in each album, including photo ID, photo name, file name, album ID and Upload time and other fields. The table can be created using the following SQL statement:
CREATE TABLE photos(
id INT(11) PRIMARY KEY AUTO_INCREMENT,
name VARCHAR(100) NOT NULL,
filename VARCHAR(255) NOT NULL,
album_id INT(11) NOT NULL,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
FOREIGN KEY (album_id) REFERENCES albums(id) ON DELETE CASCADE
);
3. Create an album page
Next, we can create a file named "index.php" to display the album list and upload new albums. You can use the following code:
<?php // 导入数据库连接配置文件 require_once 'config.php'; // 查询相册列表 $query = "SELECT * FROM albums"; $result = mysqli_query($conn, $query); ?> <!DOCTYPE html> <html> <head> <title>相册列表</title> <style> .album { margin-bottom: 20px; } </style> </head> <body> <h1 id="相册列表">相册列表</h1> <?php while ($row = mysqli_fetch_assoc($result)): ?> <div class="album"> <h2><?php echo $row['name']; ?></h2> <p>创建时间:<?php echo $row['created_at']; ?></p> <p><a href="view_album.php?id=<?php echo $row['id']; ?>">查看相册</a></p> </div> <?php endwhile; ?> <h2 id="上传新相册">上传新相册</h2> <form action="upload_album.php" method="post"> <label for="name">相册名称:</label> <input type="text" name="name" id="name" required> <input type="submit" value="上传"> </form> </body> </html>
4. Create an album details page
Create a file named "view_album.php" to display the photos in the specified album. You can use the following code:
<?php // 导入数据库连接配置文件 require_once 'config.php'; // 获取相册ID $albumId = $_GET['id']; // 查询相册名称 $query = "SELECT name FROM albums WHERE id = $albumId"; $result = mysqli_query($conn, $query); $albumName = mysqli_fetch_assoc($result)['name']; // 查询相册中的照片列表 $query = "SELECT * FROM photos WHERE album_id = $albumId"; $result = mysqli_query($conn, $query); ?> <!DOCTYPE html> <html> <head> <title>相册详情 - <?php echo $albumName; ?></title> <style> .photo { margin-bottom: 20px; } </style> </head> <body> <h1><?php echo $albumName; ?></h1> <?php while ($row = mysqli_fetch_assoc($result)): ?> <div class="photo"> <h2><?php echo $row['name']; ?></h2> <p>上传时间:<?php echo $row['created_at']; ?></p> <img src="uploads/<?php echo $row['filename']; ? alt="How to use PHP to develop a simple online photo album function" >" alt="<?php echo $row['name']; ?>" width="200"> </div> <?php endwhile; ?> <h2 id="上传新照片">上传新照片</h2> <form action="upload_photo.php" method="post" enctype="multipart/form-data"> <input type="hidden" name="album_id" value="<?php echo $albumId; ?>"> <label for="name">照片名称:</label> <input type="text" name="name" id="name" required> <br> <label for="file">照片文件:</label> <input type="file" name="file" id="file" required> <br> <input type="submit" value="上传"> </form> </body> </html>
5. Upload albums and photos
Next, we need to write the code to upload albums and photos. You can create a file named "upload_album.php" to handle the logic of uploading albums. The code is as follows:
<?php // 导入数据库连接配置文件 require_once 'config.php'; if ($_SERVER['REQUEST_METHOD'] === 'POST') { $name = $_POST['name']; // 插入相册信息 $query = "INSERT INTO albums(name) VALUES ('$name')"; mysqli_query($conn, $query); // 重定向到相册首页 header('Location: index.php'); }
Similarly, you can create a file named "upload_photo.php" to handle the logic of uploading photos. The code is as follows:
<?php // 导入数据库连接配置文件 require_once 'config.php'; if ($_SERVER['REQUEST_METHOD'] === 'POST') { $name = $_POST['name']; $albumId = $_POST['album_id']; $filename = $_FILES['file']['name']; // 上传照片文件到服务器 move_uploaded_file($_FILES['file']['tmp_name'], 'uploads/' . $filename); // 插入照片信息 $query = "INSERT INTO photos(name, filename, album_id) VALUES ('$name', '$filename', $albumId)"; mysqli_query($conn, $query); // 重定向到相册详情页面 header("Location: view_album.php?id=$albumId"); }
6. Completion
At this point, a simple online photo album function has been developed. You can visit the "index.php" page in your browser to view the album list. Click to enter the album to view photos and upload new photos. I hope this article can help you use PHP to develop photo album functions!
The above is the detailed content of How to use PHP to develop a simple online photo album function. For more information, please follow other related articles on the PHP Chinese website!
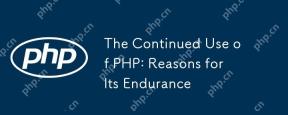
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
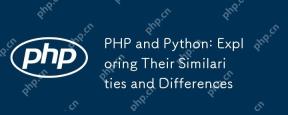
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
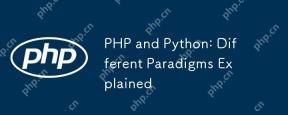
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
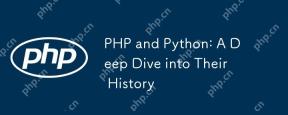
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
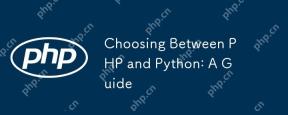
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
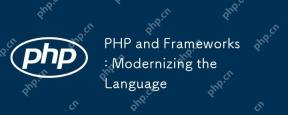
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
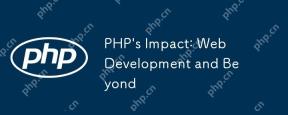
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
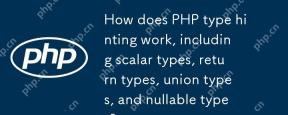
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.