How to use MySQL and Java to implement an online book lending system
Introduction:
With the advancement of informatization in modern society, more and more people choose Borrow books on the Internet. In order to facilitate users to borrow books, an efficient and reliable online book lending system needs to be established. MySQL and Java are currently one of the most widely used relational databases and programming languages. This article will introduce how to use MySQL and Java to implement an online book lending system and provide specific code examples.
- Database Design
Before you start writing code, you first need to design a suitable database model. The following is a simple database model example:
- Table Book: stores basic information about books, including book ID, title, author, publisher and other fields.
- Table User: stores basic user information, including user ID, user name, password and other fields.
- Table Borrow: stores borrowing records, including borrowing ID, book ID, user ID, borrowing date, return date and other fields.
- Create database and tables
First, create a database in MySQL, for example, named "library", and then create the above three tables. You can use the following SQL statement to create a table:
CREATE TABLE Book ( bookId INT AUTO_INCREMENT PRIMARY KEY, title VARCHAR(255), author VARCHAR(255), publisher VARCHAR(255) ); CREATE TABLE User ( userId INT AUTO_INCREMENT PRIMARY KEY, username VARCHAR(255), password VARCHAR(255) ); CREATE TABLE Borrow ( borrowId INT AUTO_INCREMENT PRIMARY KEY, bookId INT, userId INT, borrowDate DATE, returnDate DATE, FOREIGN KEY (bookId) REFERENCES Book(bookId), FOREIGN KEY (userId) REFERENCES User(userId) );
- Java code implementation
Next, we start using Java to implement the online book lending system. Here are some Java code examples:
- Add books:
public class BookDao { public void addBook(Book book) { // 连接数据库 Connection connection = // 连接数据库代码 // 执行插入操作 String sql = "INSERT INTO Book (title, author, publisher) VALUES (?, ?, ?)"; PreparedStatement statement = connection.prepareStatement(sql); statement.setString(1, book.getTitle()); statement.setString(2, book.getAuthor()); statement.setString(3, book.getPublisher()); statement.executeUpdate(); // 关闭连接 connection.close(); } }
- Borrow books:
public class BorrowDao { public void borrowBook(int bookId, int userId) { // 连接数据库 Connection connection = // 连接数据库代码 // 执行插入操作 String sql = "INSERT INTO Borrow (bookId, userId, borrowDate) VALUES (?, ?, ?)"; PreparedStatement statement = connection.prepareStatement(sql); statement.setInt(1, bookId); statement.setInt(2, userId); statement.setDate(3, new Date(System.currentTimeMillis())); statement.executeUpdate(); // 关闭连接 connection.close(); } }
- Return books:
public class BorrowDao { public void returnBook(int borrowId) { // 连接数据库 Connection connection = // 连接数据库代码 // 执行更新操作 String sql = "UPDATE Borrow SET returnDate = ? WHERE borrowId = ?"; PreparedStatement statement = connection.prepareStatement(sql); statement.setDate(1, new Date(System.currentTimeMillis())); statement.setInt(2, borrowId); statement.executeUpdate(); // 关闭连接 connection.close(); } }
The above are just some simple sample codes. In actual development, more complete code needs to be written according to specific needs. You can also use Java's database operation framework, such as MyBatis or Hibernate, to simplify database operations.
Summary:
This article introduces how to use MySQL and Java to implement an online book lending system, and provides specific database design and Java code examples. Through this system, users can conveniently borrow books on the Internet, improving borrowing efficiency and user experience. Of course, developing a complete online book lending system also requires consideration of many other factors, such as user authentication, book search, etc., but the code examples provided in this article can be used as a starting point to help readers further in-depth learning and development.
The above is the detailed content of How to implement an online book lending system using MySQL and Java. For more information, please follow other related articles on the PHP Chinese website!
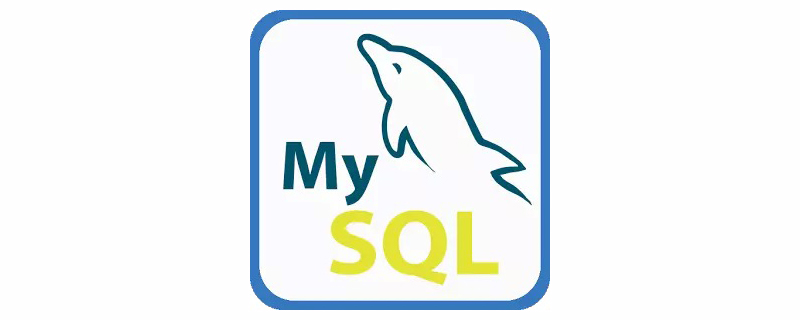
本篇文章给大家带来了关于mysql的相关知识,其中主要介绍了关于架构原理的相关内容,MySQL Server架构自顶向下大致可以分网络连接层、服务层、存储引擎层和系统文件层,下面一起来看一下,希望对大家有帮助。
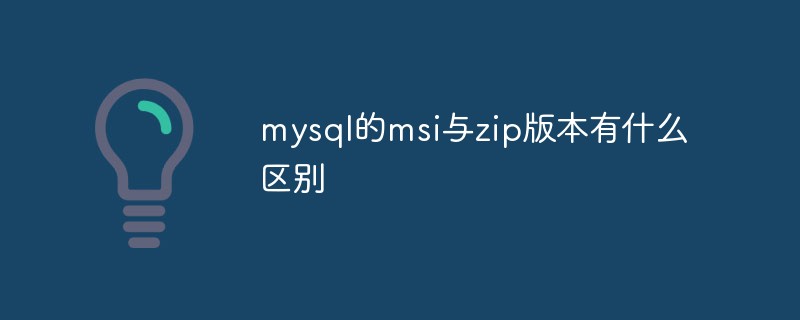
mysql的msi与zip版本的区别:1、zip包含的安装程序是一种主动安装,而msi包含的是被installer所用的安装文件以提交请求的方式安装;2、zip是一种数据压缩和文档存储的文件格式,msi是微软格式的安装包。
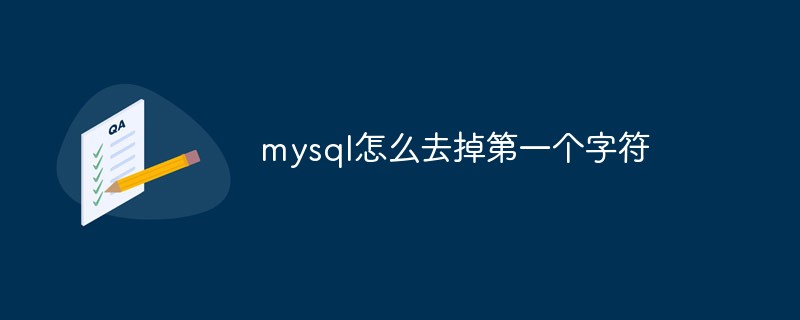
方法:1、利用right函数,语法为“update 表名 set 指定字段 = right(指定字段, length(指定字段)-1)...”;2、利用substring函数,语法为“select substring(指定字段,2)..”。
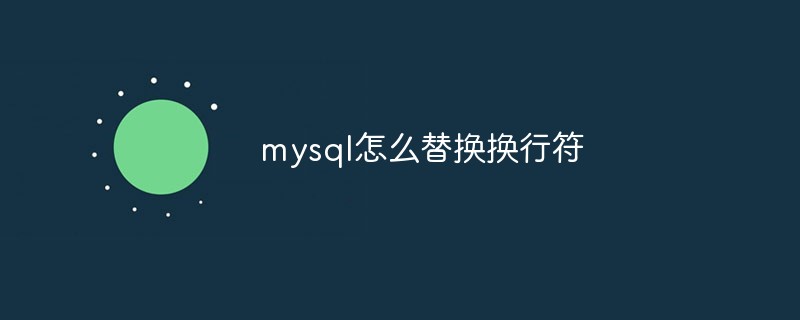
在mysql中,可以利用char()和REPLACE()函数来替换换行符;REPLACE()函数可以用新字符串替换列中的换行符,而换行符可使用“char(13)”来表示,语法为“replace(字段名,char(13),'新字符串') ”。
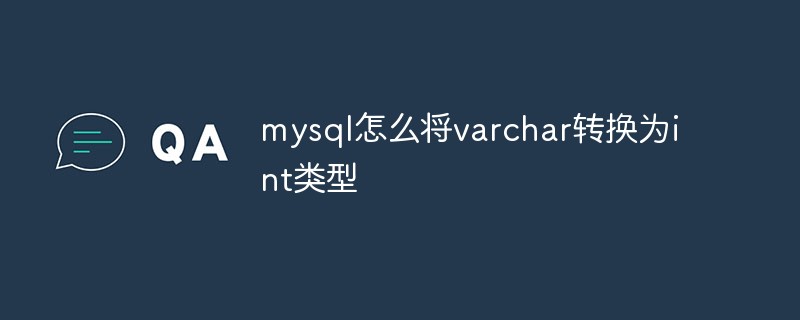
转换方法:1、利用cast函数,语法“select * from 表名 order by cast(字段名 as SIGNED)”;2、利用“select * from 表名 order by CONVERT(字段名,SIGNED)”语句。
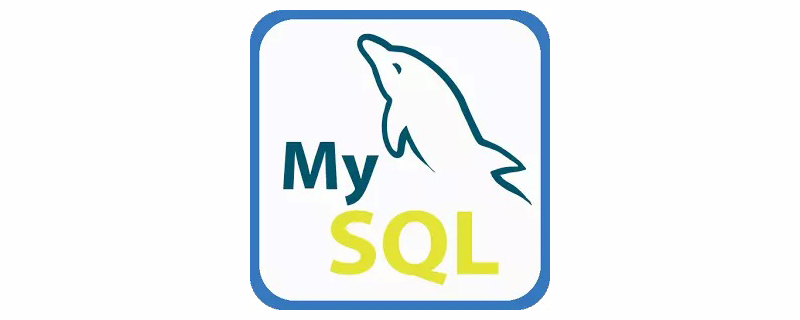
本篇文章给大家带来了关于mysql的相关知识,其中主要介绍了关于MySQL复制技术的相关问题,包括了异步复制、半同步复制等等内容,下面一起来看一下,希望对大家有帮助。
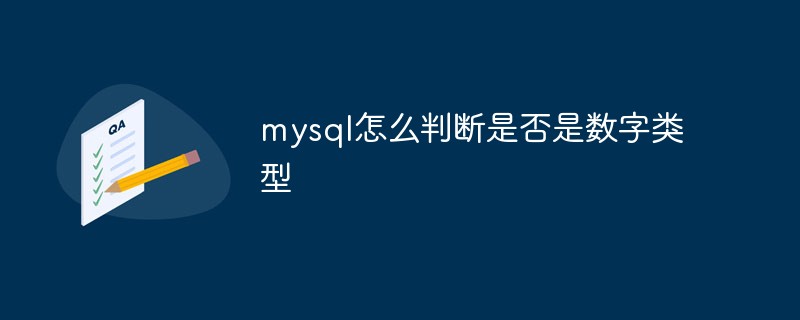
在mysql中,可以利用REGEXP运算符判断数据是否是数字类型,语法为“String REGEXP '[^0-9.]'”;该运算符是正则表达式的缩写,若数据字符中含有数字时,返回的结果是true,反之返回的结果是false。
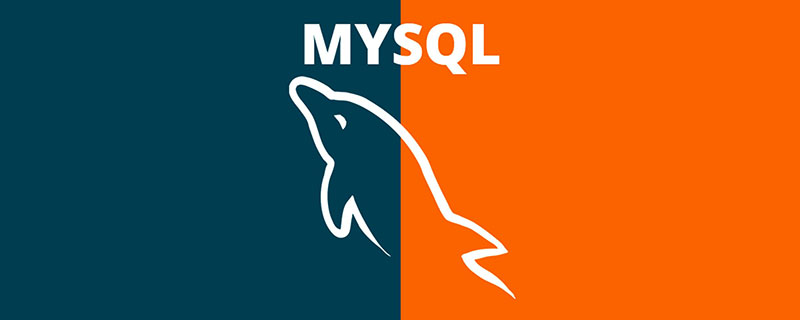
本篇文章给大家带来了关于mysql的相关知识,其中主要介绍了mysql高级篇的一些问题,包括了索引是什么、索引底层实现等等问题,下面一起来看一下,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
