How to write custom storage engines and triggers in MySQL using Python
How to use Python to write custom storage engines and triggers in MySQL
Introduction:
MySQL is a powerful relational database management system. Allows users to interact with it using multiple programming languages. Among them, Python is a widely used scripting language with simple syntax, easy to learn and use. In MySQL, we can use Python to write custom storage engines and triggers to meet some special needs. This article will introduce in detail how to use Python to write custom storage engines and triggers, and provide specific code examples.
1. Custom storage engine
- Create a Python script file for a custom storage engine
First, we need to create a Python script file named "custom_engine.py" (You can name it according to actual needs). In this script file, we need to introduce the MySQLdb module and define a StorageEngine class inherited from MySQLdb, which is the entrance to the custom storage engine.
import MySQLdb class MyStorageEngine(MySQLdb.StorageEngine): def __init__(self): MySQLdb.StorageEngine.__init__(self) # 在此处进行一些初始化操作 pass def open(self, name, mode='r'): # 在此处编写自定义存储引擎的逻辑 pass def create(self, name): # 在此处编写自定义存储引擎的逻辑 pass def close(self, name): # 在此处编写自定义存储引擎的逻辑 pass def delete(self, name): # 在此处编写自定义存储引擎的逻辑 pass
- Register a custom storage engine
Next, we need to use MySQL's "CREATE FUNCTION" statement to register the custom storage engine into MySQL and define its related parameters . Assuming that our database is named "testdb", a custom storage engine can be registered through the following logic:
CREATE FUNCTION my_storage_engine RETURNS INTEGER SONAME 'custom_engine.so';
In the above statement, "my_storage_engine" is the name of the custom storage engine, "custom_engine. so" is a shared library file for a custom storage engine. It should be noted that the shared_library plug-in must be enabled, which can be checked by executing the "SHOW PLUGINS" command.
- Using a custom storage engine in the table
When creating a table in our database, you can specify the use of a custom storage engine. For example, we can create a table using a custom storage engine using the following statement:
CREATE TABLE my_table (id INT, name VARCHAR(100)) ENGINE=my_storage_engine;
In this example, "my_table" is the name of the table, and "id" and "name" are the Column, "ENGINE=my_storage_engine" specifies that the storage engine used by the table is our custom storage engine.
2. Custom triggers
- Create a Python script file for a custom trigger
Similar to creating a custom storage engine, we need to create a Python script file (for example "custom_trigger.py"), which defines a Trigger class inherited from MySQLdb, which is the entrance to custom triggers.
import MySQLdb class MyTrigger(MySQLdb.Trigger): def __init__(self): MySQLdb.Trigger.__init__(self) # 在此处进行一些初始化操作 pass def before_insert(self, row): # 在此处编写自定义触发器的逻辑 pass def after_insert(self, row): # 在此处编写自定义触发器的逻辑 pass def before_update(self, old_row, new_row): # 在此处编写自定义触发器的逻辑 pass def after_update(self, old_row, new_row): # 在此处编写自定义触发器的逻辑 pass def before_delete(self, row): # 在此处编写自定义触发器的逻辑 pass def after_delete(self, row): # 在此处编写自定义触发器的逻辑 pass
- Register a custom trigger
We can use MySQL's "CREATE TRIGGER" statement to register a custom trigger into MySQL and specify its related parameters. Assuming that our database is named "testdb", a custom trigger can be registered through the following logic:
CREATE TRIGGER my_trigger BEFORE INSERT ON my_table FOR EACH ROW BEGIN CALL my_trigger_func(NEW.id, NEW.name); END;
In the above statement, "my_trigger" is the name of the custom trigger, "my_table" is the name of the table where the trigger is located, and "my_trigger_func" is the callback function of the trigger.
- Using custom triggers in tables
When creating a table in our database, you can specify the use of custom triggers. For example, we can create a custom trigger on the "my_table" table using the following statement:
CREATE TABLE my_table (id INT, name VARCHAR(100));
In this example, the trigger will be fired before the insert operation on the "my_table" table.
Conclusion:
This article introduces how to use Python to write custom storage engines and triggers in MySQL, and provides specific code examples. By customizing storage engines and triggers, we can enhance the functionality and flexibility of MySQL according to actual needs. I hope this article helps you write custom storage engines and triggers in MySQL using Python.
The above is the detailed content of How to write custom storage engines and triggers in MySQL using Python. For more information, please follow other related articles on the PHP Chinese website!
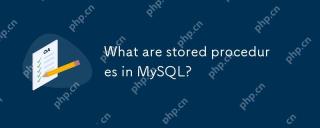
Stored procedures are precompiled SQL statements in MySQL for improving performance and simplifying complex operations. 1. Improve performance: After the first compilation, subsequent calls do not need to be recompiled. 2. Improve security: Restrict data table access through permission control. 3. Simplify complex operations: combine multiple SQL statements to simplify application layer logic.
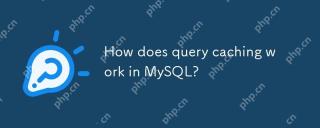
The working principle of MySQL query cache is to store the results of SELECT query, and when the same query is executed again, the cached results are directly returned. 1) Query cache improves database reading performance and finds cached results through hash values. 2) Simple configuration, set query_cache_type and query_cache_size in MySQL configuration file. 3) Use the SQL_NO_CACHE keyword to disable the cache of specific queries. 4) In high-frequency update environments, query cache may cause performance bottlenecks and needs to be optimized for use through monitoring and adjustment of parameters.
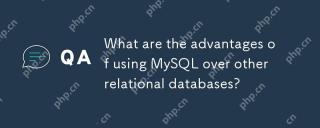
The reasons why MySQL is widely used in various projects include: 1. High performance and scalability, supporting multiple storage engines; 2. Easy to use and maintain, simple configuration and rich tools; 3. Rich ecosystem, attracting a large number of community and third-party tool support; 4. Cross-platform support, suitable for multiple operating systems.
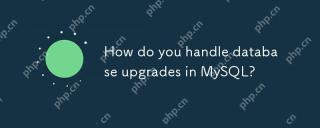
The steps for upgrading MySQL database include: 1. Backup the database, 2. Stop the current MySQL service, 3. Install the new version of MySQL, 4. Start the new version of MySQL service, 5. Recover the database. Compatibility issues are required during the upgrade process, and advanced tools such as PerconaToolkit can be used for testing and optimization.
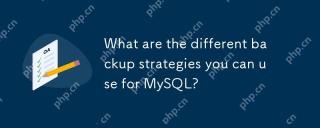
MySQL backup policies include logical backup, physical backup, incremental backup, replication-based backup, and cloud backup. 1. Logical backup uses mysqldump to export database structure and data, which is suitable for small databases and version migrations. 2. Physical backups are fast and comprehensive by copying data files, but require database consistency. 3. Incremental backup uses binary logging to record changes, which is suitable for large databases. 4. Replication-based backup reduces the impact on the production system by backing up from the server. 5. Cloud backups such as AmazonRDS provide automation solutions, but costs and control need to be considered. When selecting a policy, database size, downtime tolerance, recovery time, and recovery point goals should be considered.
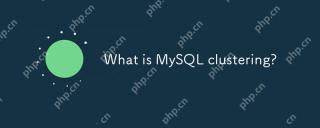
MySQLclusteringenhancesdatabaserobustnessandscalabilitybydistributingdataacrossmultiplenodes.ItusestheNDBenginefordatareplicationandfaulttolerance,ensuringhighavailability.Setupinvolvesconfiguringmanagement,data,andSQLnodes,withcarefulmonitoringandpe
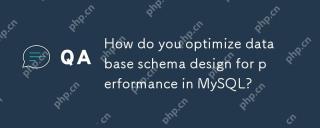
Optimizing database schema design in MySQL can improve performance through the following steps: 1. Index optimization: Create indexes on common query columns, balancing the overhead of query and inserting updates. 2. Table structure optimization: Reduce data redundancy through normalization or anti-normalization and improve access efficiency. 3. Data type selection: Use appropriate data types, such as INT instead of VARCHAR, to reduce storage space. 4. Partitioning and sub-table: For large data volumes, use partitioning and sub-table to disperse data to improve query and maintenance efficiency.
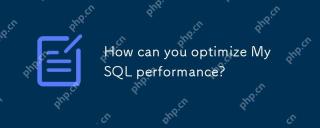
TooptimizeMySQLperformance,followthesesteps:1)Implementproperindexingtospeedupqueries,2)UseEXPLAINtoanalyzeandoptimizequeryperformance,3)Adjustserverconfigurationsettingslikeinnodb_buffer_pool_sizeandmax_connections,4)Usepartitioningforlargetablestoi


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
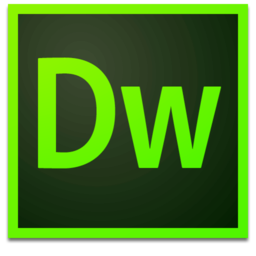
Dreamweaver Mac version
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
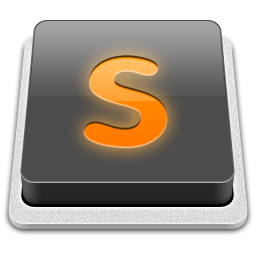
SublimeText3 Mac version
God-level code editing software (SublimeText3)
