


How to write custom triggers, storage engines and functions in MySQL using Python
How to use Python to write custom triggers, storage engines and functions in MySQL
MySQL is a widely used relational database management system, and Python is A powerful scripting language. Combining the two, we can implement custom triggers, storage engines and functions by writing Python scripts. This article will introduce how to use Python to write custom triggers, storage engines and functions in MySQL, and provide specific code examples.
1. Custom triggers
A trigger is a series of operations that are automatically executed when a specific event (such as INSERT, UPDATE or DELETE) occurs. Custom triggers can be written using Python. Here is an example that demonstrates how to write a trigger in MySQL using Python that automatically updates other tables when a new record is inserted:
import MySQLdb def update_other_table(trigger_table, update_table): db = MySQLdb.connect(host="localhost", user="root", passwd="password", db="test") cursor = db.cursor() # 获取插入的记录信息 cursor.execute("SELECT * FROM %s ORDER BY id DESC LIMIT 1" % trigger_table) result = cursor.fetchone() # 更新其他表 cursor.execute("UPDATE %s SET column1='%s' WHERE column2='%s'" % (update_table, result[1], result[2])) db.commit() db.close() # 创建触发器 def create_trigger(trigger_table, update_table): db = MySQLdb.connect(host="localhost", user="root", passwd="password", db="test") cursor = db.cursor() # 创建触发器 cursor.execute("DELIMITER //") cursor.execute("CREATE TRIGGER my_trigger AFTER INSERT ON %s FOR EACH ROW BEGIN CALL update_other_table('%s', '%s'); END //") cursor.execute("DELIMITER ;") db.commit() db.close() # 测试触发器 create_trigger("table1", "table2")
In the above example, we first connect to the database through the MySQLdb module. Then, a function update_other_table() is defined to update other tables. This function obtains the inserted record information by executing SQL statements and uses this information to update other tables. Next, we define a function create_trigger() for creating triggers. In this function, we create a trigger using the CREATE TRIGGER statement and call the update_other_table() function using the CALL statement. Finally, we create the trigger by calling the create_trigger() function.
2. Custom storage engine
The storage engine is a mechanism used to manage tables in MySQL. MySQL supports multiple storage engines, including the default InnoDB and MyISAM. We can use Python to write custom storage engines to meet specific needs. Here is an example that demonstrates how to write a simple storage engine in MySQL using Python that can save data to a text file as records are inserted:
import MySQLdb class MyStorageEngine: def __init__(self): self.file = open("data.txt", "a") def create(self, table_name, fields): pass def open(self, table_name): pass def close(self, table_name): pass def write_row(self, table_name, values): row_data = " ".join(values) + " " self.file.write(row_data) # 注册存储引擎 def register_storage_engine(engine_name): db = MySQLdb.connect(host="localhost", user="root", passwd="password", db="test") cursor = db.cursor() # 创建存储引擎 cursor.execute("INSTALL PLUGIN %s SONAME 'my_engine.so'" % engine_name) db.commit() db.close() # 测试存储引擎 register_storage_engine("my_engine")
In the above example, we defined a file named The MyStorageEngine class implements the methods required by the storage engine. In the write_row() method of this class, we write the recorded values in tab-delimited form to a text file named data.txt. Then, we define a function register_storage_engine() to register the storage engine. In this function, we create a storage engine using the INSTALL PLUGIN statement. Finally, we register the storage engine by calling the register_storage_engine() function.
3. Custom functions
MySQL allows users to define their own functions for use in SQL statements. We can write custom functions using Python to meet specific needs. Here is an example that demonstrates how to write a simple custom function in MySQL using Python to calculate the sum of two numbers:
import MySQLdb # 自定义函数 def my_sum(a, b): return a + b # 注册自定义函数 def register_function(function_name): db = MySQLdb.connect(host="localhost", user="root", passwd="password", db="test") cursor = db.cursor() # 创建自定义函数 cursor.execute("CREATE FUNCTION %s RETURNS INTEGER SONAME 'my_function.so'" % function_name) db.commit() db.close() # 测试自定义函数 register_function("my_sum")
In the above example, we defined a function called my_sum() function that calculates the sum of two numbers. Then, we define a function register_function() for registering custom functions. In this function, we create a custom function using the CREATE FUNCTION statement. Finally, we register the custom function by calling the register_function() function.
Summary:
The above is an introduction and code examples for using Python to write custom triggers, storage engines and functions in MySQL. By writing Python scripts, we can extend and customize MySQL functions more flexibly. I hope this article will help you apply Python programming in MySQL.
The above is the detailed content of How to write custom triggers, storage engines and functions in MySQL using Python. For more information, please follow other related articles on the PHP Chinese website!
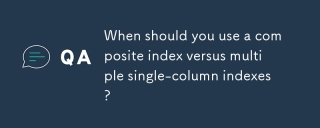
In database optimization, indexing strategies should be selected according to query requirements: 1. When the query involves multiple columns and the order of conditions is fixed, use composite indexes; 2. When the query involves multiple columns but the order of conditions is not fixed, use multiple single-column indexes. Composite indexes are suitable for optimizing multi-column queries, while single-column indexes are suitable for single-column queries.
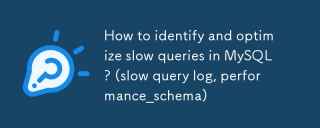
To optimize MySQL slow query, slowquerylog and performance_schema need to be used: 1. Enable slowquerylog and set thresholds to record slow query; 2. Use performance_schema to analyze query execution details, find out performance bottlenecks and optimize.
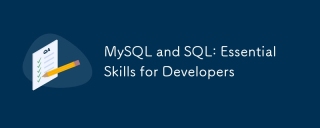
MySQL and SQL are essential skills for developers. 1.MySQL is an open source relational database management system, and SQL is the standard language used to manage and operate databases. 2.MySQL supports multiple storage engines through efficient data storage and retrieval functions, and SQL completes complex data operations through simple statements. 3. Examples of usage include basic queries and advanced queries, such as filtering and sorting by condition. 4. Common errors include syntax errors and performance issues, which can be optimized by checking SQL statements and using EXPLAIN commands. 5. Performance optimization techniques include using indexes, avoiding full table scanning, optimizing JOIN operations and improving code readability.
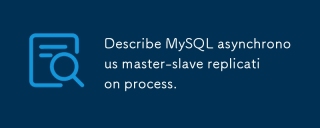
MySQL asynchronous master-slave replication enables data synchronization through binlog, improving read performance and high availability. 1) The master server record changes to binlog; 2) The slave server reads binlog through I/O threads; 3) The server SQL thread applies binlog to synchronize data.
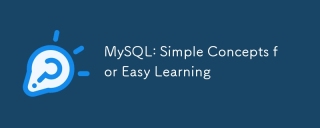
MySQL is an open source relational database management system. 1) Create database and tables: Use the CREATEDATABASE and CREATETABLE commands. 2) Basic operations: INSERT, UPDATE, DELETE and SELECT. 3) Advanced operations: JOIN, subquery and transaction processing. 4) Debugging skills: Check syntax, data type and permissions. 5) Optimization suggestions: Use indexes, avoid SELECT* and use transactions.
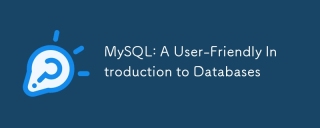
The installation and basic operations of MySQL include: 1. Download and install MySQL, set the root user password; 2. Use SQL commands to create databases and tables, such as CREATEDATABASE and CREATETABLE; 3. Execute CRUD operations, use INSERT, SELECT, UPDATE, DELETE commands; 4. Create indexes and stored procedures to optimize performance and implement complex logic. With these steps, you can build and manage MySQL databases from scratch.
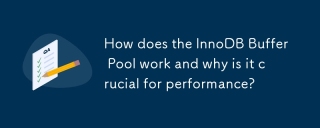
InnoDBBufferPool improves the performance of MySQL databases by loading data and index pages into memory. 1) The data page is loaded into the BufferPool to reduce disk I/O. 2) Dirty pages are marked and refreshed to disk regularly. 3) LRU algorithm management data page elimination. 4) The read-out mechanism loads the possible data pages in advance.
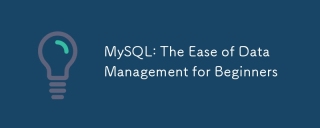
MySQL is suitable for beginners because it is simple to install, powerful and easy to manage data. 1. Simple installation and configuration, suitable for a variety of operating systems. 2. Support basic operations such as creating databases and tables, inserting, querying, updating and deleting data. 3. Provide advanced functions such as JOIN operations and subqueries. 4. Performance can be improved through indexing, query optimization and table partitioning. 5. Support backup, recovery and security measures to ensure data security and consistency.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
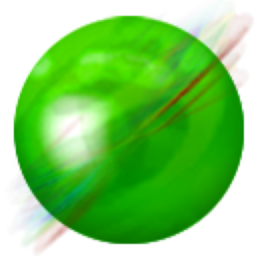
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use