


How to develop a simple online investment platform using MySQL and Go language
How to use MySQL and Go language to develop a simple online investment platform
Introduction:
As a digital financial service, the development of online investment platforms is increasingly attracted people's attention. This article will introduce how to use MySQL and Go language to develop a simple online investment platform, including database design and related code examples.
- Database design:
First of all, we need to design the structure of the database to store data such as user information, investment product information, and transaction records. The following is an example database table design:
- User table (User):
Fields: User ID (ID), Username (Username), Password (Password), Email (Email), Account Balance (Balance), Registration Time (CreatedAt)
Primary Key: User ID (ID) - Investment Product Table (Investment):
Field: Product ID (ID), Product name (Name), investment amount (Amount), expected interest rate (ExpectedReturn), investment term (InvestmentTerm)
Primary key: Product ID (ID) - Transaction record table (Transaction):
field : Transaction ID (ID), User ID (UserID), Product ID (InvestmentID), Transaction time (Time), Transaction amount (Amount)
Primary key: Transaction ID (ID)
Foreign key: User ID (UserID) ), product ID (InvestmentID)
- Database connection configuration:
In Go language, we can use third-party libraries such as "database/sql" and "github.com/go -sql-driver/mysql" to connect to the MySQL database. The following is a sample code for a simple database connection configuration:
import (
"database/sql" "fmt" "log" _ "github.com/go-sql-driver/mysql"
)
func connectDB() (*sql.DB, error) {
db, err := sql.Open("mysql", "username:password@tcp(localhost:3306)/database_name") if err != nil { log.Fatal(err) return nil, err } err = db.Ping() if err != nil { log.Fatal(err) return nil, err } fmt.Println("Connected to the database!") return db, nil
}
- User registration function:
User registration is one of the basic functions of the online investment platform. The following is the code for an example user registration function:
import (
"database/sql" "fmt" "log" "net/http"
)
func registerUser(w http.ResponseWriter, r *http.Request) {
username := r.FormValue("username") password := r.FormValue("password") email := r.FormValue("email") db, err := connectDB() if err != nil { log.Fatal(err) http.Error(w, "Internal Server Error", http.StatusInternalServerError) return } defer db.Close() stmt, err := db.Prepare("INSERT INTO User (Username, Password, Email) VALUES (?, ?, ?)") if err != nil { log.Fatal(err) http.Error(w, "Internal Server Error", http.StatusInternalServerError) return } defer stmt.Close() _, err = stmt.Exec(username, password, email) if err != nil { log.Fatal(err) http.Error(w, "Internal Server Error", http.StatusInternalServerError) return } fmt.Fprintln(w, "Registration successful!")
}
- Investment product display function:
Displaying investment products is another basic function of the online investment platform. The following is the code for an example investment product display function:
import (
"database/sql" "fmt" "log" "net/http"
)
type Investment struct {
ID int Name string Amount float64 ExpectedReturn float64 InvestmentTerm int
}
func getInvestmentList(w http.ResponseWriter, r *http.Request) {
db, err := connectDB() if err != nil { log.Fatal(err) http.Error(w, "Internal Server Error", http.StatusInternalServerError) return } defer db.Close() rows, err := db.Query("SELECT * FROM Investment") if err != nil { log.Fatal(err) http.Error(w, "Internal Server Error", http.StatusInternalServerError) return } defer rows.Close() var investments []Investment for rows.Next() { var investment Investment err := rows.Scan(&investment.ID, &investment.Name, &investment.Amount, &investment.ExpectedReturn, &investment.InvestmentTerm) if err != nil { log.Fatal(err) http.Error(w, "Internal Server Error", http.StatusInternalServerError) return } investments = append(investments, investment) } for _, investment := range investments { fmt.Fprintf(w, "ID: %d, Name: %s, Amount: %.2f, Expected Return: %.2f%%, Investment Term: %d months
", investment.ID, investment.Name, investment.Amount, investment.ExpectedReturn, investment.InvestmentTerm)
}
}
- Summary:
This article introduces how to use MySQL and Go language to develop a simple online investment platform. Through database design and corresponding code examples, we can realize user Basic functions such as registration, investment product display, etc. Of course, this is just a simple example, and the actual online investment platform requires more complex functions and more comprehensive security measures. However, through this example, readers can understand the use of MySQL and Go language Have a basic understanding and inspiration of the process of developing an online investment platform.
The above is the detailed content of How to develop a simple online investment platform using MySQL and Go language. For more information, please follow other related articles on the PHP Chinese website!
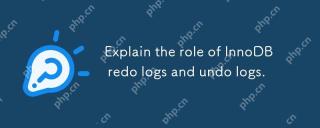
InnoDB uses redologs and undologs to ensure data consistency and reliability. 1.redologs record data page modification to ensure crash recovery and transaction persistence. 2.undologs records the original data value and supports transaction rollback and MVCC.
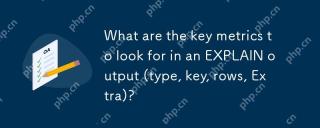
Key metrics for EXPLAIN commands include type, key, rows, and Extra. 1) The type reflects the access type of the query. The higher the value, the higher the efficiency, such as const is better than ALL. 2) The key displays the index used, and NULL indicates no index. 3) rows estimates the number of scanned rows, affecting query performance. 4) Extra provides additional information, such as Usingfilesort prompts that it needs to be optimized.
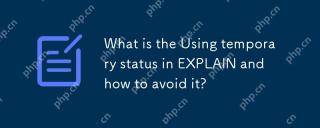
Usingtemporary indicates that the need to create temporary tables in MySQL queries, which are commonly found in ORDERBY using DISTINCT, GROUPBY, or non-indexed columns. You can avoid the occurrence of indexes and rewrite queries and improve query performance. Specifically, when Usingtemporary appears in EXPLAIN output, it means that MySQL needs to create temporary tables to handle queries. This usually occurs when: 1) deduplication or grouping when using DISTINCT or GROUPBY; 2) sort when ORDERBY contains non-index columns; 3) use complex subquery or join operations. Optimization methods include: 1) ORDERBY and GROUPB
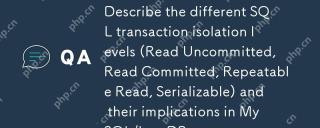
MySQL/InnoDB supports four transaction isolation levels: ReadUncommitted, ReadCommitted, RepeatableRead and Serializable. 1.ReadUncommitted allows reading of uncommitted data, which may cause dirty reading. 2. ReadCommitted avoids dirty reading, but non-repeatable reading may occur. 3.RepeatableRead is the default level, avoiding dirty reading and non-repeatable reading, but phantom reading may occur. 4. Serializable avoids all concurrency problems but reduces concurrency. Choosing the appropriate isolation level requires balancing data consistency and performance requirements.
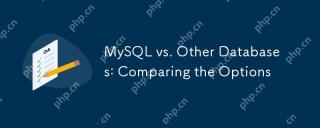
MySQL is suitable for web applications and content management systems and is popular for its open source, high performance and ease of use. 1) Compared with PostgreSQL, MySQL performs better in simple queries and high concurrent read operations. 2) Compared with Oracle, MySQL is more popular among small and medium-sized enterprises because of its open source and low cost. 3) Compared with Microsoft SQL Server, MySQL is more suitable for cross-platform applications. 4) Unlike MongoDB, MySQL is more suitable for structured data and transaction processing.
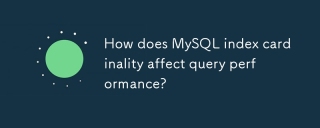
MySQL index cardinality has a significant impact on query performance: 1. High cardinality index can more effectively narrow the data range and improve query efficiency; 2. Low cardinality index may lead to full table scanning and reduce query performance; 3. In joint index, high cardinality sequences should be placed in front to optimize query.
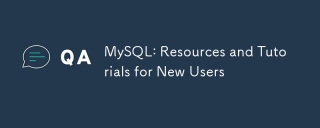
The MySQL learning path includes basic knowledge, core concepts, usage examples, and optimization techniques. 1) Understand basic concepts such as tables, rows, columns, and SQL queries. 2) Learn the definition, working principles and advantages of MySQL. 3) Master basic CRUD operations and advanced usage, such as indexes and stored procedures. 4) Familiar with common error debugging and performance optimization suggestions, such as rational use of indexes and optimization queries. Through these steps, you will have a full grasp of the use and optimization of MySQL.
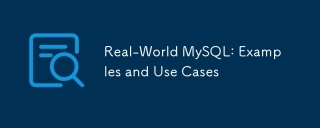
MySQL's real-world applications include basic database design and complex query optimization. 1) Basic usage: used to store and manage user data, such as inserting, querying, updating and deleting user information. 2) Advanced usage: Handle complex business logic, such as order and inventory management of e-commerce platforms. 3) Performance optimization: Improve performance by rationally using indexes, partition tables and query caches.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Chinese version
Chinese version, very easy to use
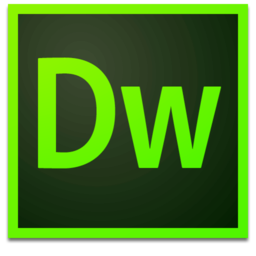
Dreamweaver Mac version
Visual web development tools