Dynamic value is the value we assign to the dynamic variable. A dynamic variable is a variable that is hard-coded in the code without a specific name, and its address is determined while the code is running. The name "dynamic" refers to a value that can be manipulated and changed.
Here we will see how to create a dynamic value (also part of the object value) in JavaScript and change the dynamic variable name without accessing the group in the future. It means that we declare a variable and then we use the same variable as one of the keys in the object and in the future if we need to change the name of the variable we can change it without accessing the object.
To accomplish the above task, we just assign the variable name in the object using square brackets [ ] as shown below -
Syntax
The following is the syntax for creating dynamic values and objects -
const key = 'KeyName'; const obj = { [key] : 'value'};
Here key and value are the key-value pairs used to create objects," obj” and keyName > are the values of the key.
Algorithm
Step 1 - Define the keys used to create the object.
Step 2 - Create the object and use the keys defined above.
-
Step 3 - Apply JSON.stringify() to the object created above to display the object.
Example 1
We can use the following HTML program to view the declaration of dynamic variables.
<!DOCTYPE html> <html> <body> <h2 id="JavaScript-Dynamic-values"> JavaScript Dynamic values </h2> <div id = "result"> </div> <script> const key1 = "Haircolour"; const key2 = "Eyecolour"; const person = { f_name : "Rohan", l_name :"Joshi", [key1] : "Black", [key2] : "Brown" }; // Converting the object value to show its value in html str = JSON.stringify(person); document.getElementById("result").innerHTML = str; // To print value of object in console console.log(person); </script> </body> </html>
So, in the above code, we can see that we declared two keys f_name and l_name in the object without using curly brackets [], and we used curly brackets for key1 and key2 variables , because these two are dynamic values.
In the output, we can see that the name of the key1 variable is Eyecolour and the value of the key2 variable is Haircolour.
Example 2
Here is another code that will shed more light on how to change the name of a dynamic variable without accessing the object. Here we just swap the names of two dynamic variables in the code.
<!DOCTYPE html> <html> <body> <h2 id="JavaScript-Dynamic-values"> JavaScript Dynamic values </h2> <div id = "result"> </div> <script> const key1 = "Haircolour"; const key2 = "Eyecolour"; const person = { f_name : "Rohan", l_name :"Joshi", [key1] : "Black", [key2] : "Brown" }; // Converting the object value to show its value in html str = JSON.stringify(person); document.getElementById("result").innerHTML = str; // To print value of object in console console.log(person); </script> </body> </html>
In the output we can see that the values of the two variables key1 and key2 remain the same but their names are changed without accessing the objects, so this is what we have in JavaScript How to create dynamic values and objects in .
The above is the detailed content of How to create dynamic values and objects in JavaScript?. For more information, please follow other related articles on the PHP Chinese website!
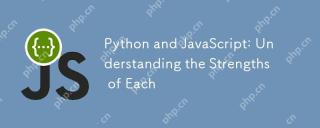
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
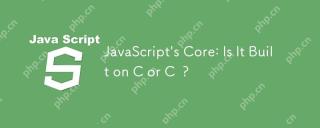
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
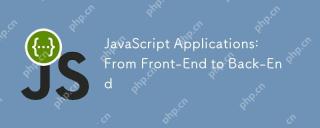
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
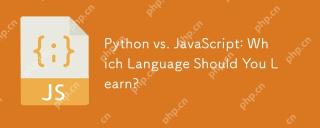
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
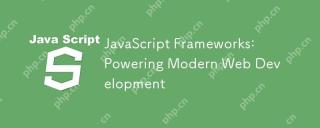
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
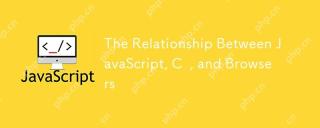
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
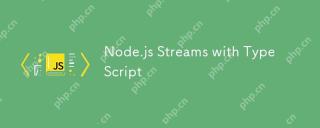
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe
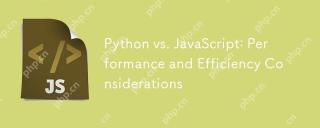
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
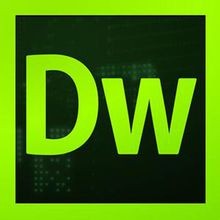
Dreamweaver CS6
Visual web development tools

Atom editor mac version download
The most popular open source editor
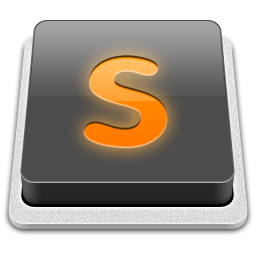
SublimeText3 Mac version
God-level code editing software (SublimeText3)
