How to develop user session management functionality using Redis and PHP
How to use Redis and PHP to develop user session management functions
Introduction:
User session management is an important function in web application development, it can help us Track and manage user login status while providing secure authentication and authorization capabilities. In this article, we will introduce how to use Redis and PHP to implement user session management functions, and attach specific code examples.
1. What is Redis?
Redis (Remote Dictionary Server) is an open source high-performance key-value storage database. It supports various data structures such as Strings, Hashes, Lists, Sets and Sorted Sets, and provides a wealth of operation commands. The advantages of Redis are fast speed, low memory usage, and support for persistence. It is suitable for scenarios such as data caching, message queues, and session storage.
2. Why choose Redis to manage user sessions?
- Superior performance: Redis is based on memory operations, has fast read and write speeds, and can handle high concurrency scenarios.
- Persistence support: Redis supports data persistence, ensuring data reliability.
- Rich data structures and commands: Redis provides rich data structures and commands to facilitate user session management functions.
3. Steps to use Redis and PHP to implement user session management:
- Installation and configuration of Redis
First, you need to install Redis on the server and configure the relevant parameter. You can learn how to install and configure Redis through the official documentation. - Connecting to Redis
Connecting to Redis in PHP requires the use of the Redis extension. You can learn how to install and configure the Redis extension through the official documentation. The following is a sample code for connecting to Redis:
<?php $redis = new Redis(); $redis->connect('localhost', 6379);
- Storing and retrieving session data
Storing and retrieving user session data through Redis. We can use a hash data structure to store the user's session information, such as user ID, user name, etc. The following is a sample code for storing and obtaining user session data:
<?php $sessionId = 'session123'; $userId = 1; $username = 'Alice'; // 存储会话数据 $redis->hSet('sessions', $sessionId, json_encode(array('id' => $userId, 'username' => $username))); // 获取会话数据 $sessionData = $redis->hGet('sessions', $sessionId); $sessionData = json_decode($sessionData, true); echo $sessionData['username']; // 输出 Alice
- Set session expiration time
In order to ensure the security and validity of the session, we can set the session expiration time . When the session expires, the user needs to log in again. The following is a sample code to set the session expiration time:
<?php $sessionId = 'session123'; $expTime = 3600; // 过期时间为 1 小时 // 设置会话过期时间 $redis->expire('sessions', $expTime);
- Update session data and expiration time
We can update the session data and expiration time every time the user visits the website, to ensure the validity of the session. The following is a sample code:
<?php $sessionId = 'session123'; // 更新会话数据 $redis->hSet('sessions', $sessionId, json_encode(array('id' => $userId, 'username' => $username))); // 更新会话过期时间 $redis->expire('sessions', $expTime);
- Delete session data
When the user logs out or the session expires, we can delete the corresponding session data. The following is a sample code:
<?php $sessionId = 'session123'; // 删除会话数据 $redis->hDel('sessions', $sessionId);
4. Summary
By using Redis and PHP to implement user session management functions, high performance, reliability and security of user login and authorization functions can be provided . This article describes how to install and configure Redis, and use PHP to connect to Redis, store and obtain session data, set expiration time, update session data and expiration time, and delete session data. Hopefully these sample codes can help you implement user session management functionality during development.
The above is the detailed content of How to develop user session management functionality using Redis and PHP. For more information, please follow other related articles on the PHP Chinese website!
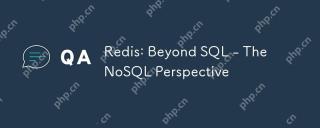
Redis goes beyond SQL databases because of its high performance and flexibility. 1) Redis achieves extremely fast read and write speed through memory storage. 2) It supports a variety of data structures, such as lists and collections, suitable for complex data processing. 3) Single-threaded model simplifies development, but high concurrency may become a bottleneck.
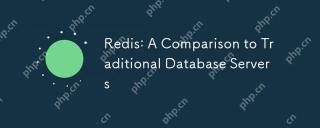
Redis is superior to traditional databases in high concurrency and low latency scenarios, but is not suitable for complex queries and transaction processing. 1.Redis uses memory storage, fast read and write speed, suitable for high concurrency and low latency requirements. 2. Traditional databases are based on disk, support complex queries and transaction processing, and have strong data consistency and persistence. 3. Redis is suitable as a supplement or substitute for traditional databases, but it needs to be selected according to specific business needs.
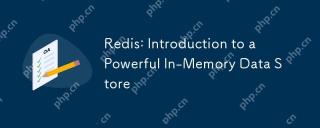
Redisisahigh-performancein-memorydatastructurestorethatexcelsinspeedandversatility.1)Itsupportsvariousdatastructureslikestrings,lists,andsets.2)Redisisanin-memorydatabasewithpersistenceoptions,ensuringfastperformanceanddatasafety.3)Itoffersatomicoper
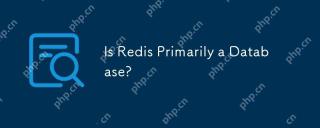
Redis is primarily a database, but it is more than just a database. 1. As a database, Redis supports persistence and is suitable for high-performance needs. 2. As a cache, Redis improves application response speed. 3. As a message broker, Redis supports publish-subscribe mode, suitable for real-time communication.
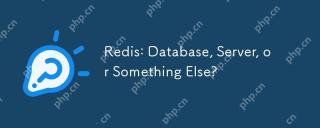
Redisisamultifacetedtoolthatservesasadatabase,server,andmore.Itfunctionsasanin-memorydatastructurestore,supportsvariousdatastructures,andcanbeusedasacache,messagebroker,sessionstorage,andfordistributedlocking.
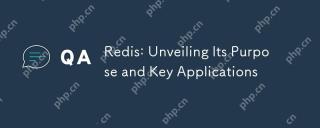
Redisisanopen-source,in-memorydatastructurestoreusedasadatabase,cache,andmessagebroker,excellinginspeedandversatility.Itiswidelyusedforcaching,real-timeanalytics,sessionmanagement,andleaderboardsduetoitssupportforvariousdatastructuresandfastdataacces
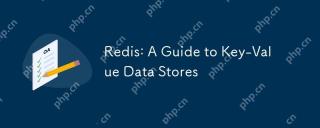
Redis is an open source memory data structure storage used as a database, cache and message broker, suitable for scenarios where fast response and high concurrency are required. 1.Redis uses memory to store data and provides microsecond read and write speed. 2. It supports a variety of data structures, such as strings, lists, collections, etc. 3. Redis realizes data persistence through RDB and AOF mechanisms. 4. Use single-threaded model and multiplexing technology to handle requests efficiently. 5. Performance optimization strategies include LRU algorithm and cluster mode.
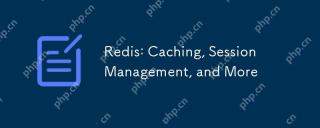
Redis's functions mainly include cache, session management and other functions: 1) The cache function stores data through memory to improve reading speed, and is suitable for high-frequency access scenarios such as e-commerce websites; 2) The session management function shares session data in a distributed system and automatically cleans it through an expiration time mechanism; 3) Other functions such as publish-subscribe mode, distributed locks and counters, suitable for real-time message push and multi-threaded systems and other scenarios.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version
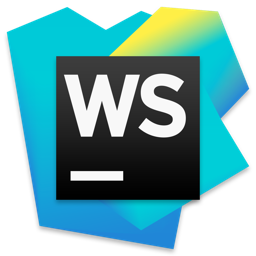
WebStorm Mac version
Useful JavaScript development tools
