How to use Java to implement the heap sort algorithm
Heap sort is a sorting algorithm based on the heap data structure, which takes advantage of the properties of the heap for sorting. Heap sort is divided into two main steps: building the heap and sorting.
Building a heap:
First, we need to build a large root heap or a small root heap based on the array to be sorted. For ascending sort, we need to build a large root heap; for descending sort, we need to build a small root heap.
The property of a large root heap is: the value of a node is greater than or equal to the value of its child nodes.
The properties of the small root heap are: the value of a node is less than or equal to the value of its child nodes.
The process of building a large root heap is as follows:
public static void buildHeap(int[] array, int length) { for (int i = length / 2 - 1; i >= 0; i--) { heapify(array, length, i); } } public static void heapify(int[] array, int length, int i) { int largest = i; int left = 2 * i + 1; int right = 2 * i + 2; if (left < length && array[left] > array[largest]) { largest = left; } if (right < length && array[right] > array[largest]) { largest = right; } if (largest != i) { int temp = array[i]; array[i] = array[largest]; array[largest] = temp; heapify(array, length, largest); } }
Sort:
After building the large root heap, we need to exchange the top element of the heap with the last element of the array, and shrink the heap range, and then heapize the heap again, repeating this process until the heap is empty. The final array is ordered.
public static void heapSort(int[] array) { int length = array.length; buildHeap(array, length); for (int i = length - 1; i >= 0; i--) { int temp = array[i]; array[i] = array[0]; array[0] = temp; heapify(array, i, 0); } }
Usage example:
public static void main(String[] args) { int[] array = {4, 10, 3, 5, 1}; heapSort(array); System.out.println(Arrays.toString(array)); }
The output result is: [1, 3, 4, 5, 10], which is the result of ascending order.
The time complexity of heap sorting is O(nlogn), where n is the number of elements to be sorted. Heap sort is a stable sorting algorithm suitable for sorting large-scale data.
The above is the detailed content of How to implement heap sort algorithm using java. For more information, please follow other related articles on the PHP Chinese website!
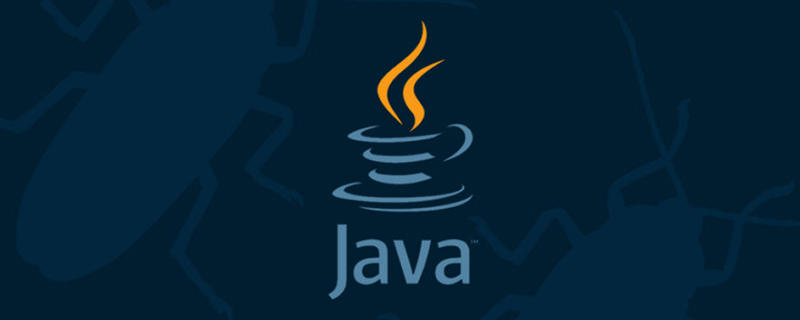
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于结构化数据处理开源库SPL的相关问题,下面就一起来看一下java下理想的结构化数据处理类库,希望对大家有帮助。
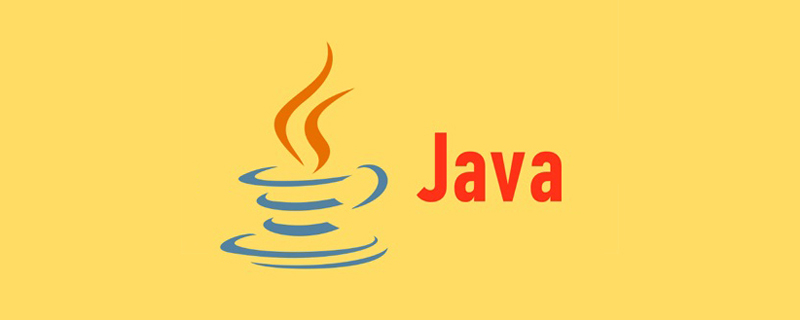
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于PriorityQueue优先级队列的相关知识,Java集合框架中提供了PriorityQueue和PriorityBlockingQueue两种类型的优先级队列,PriorityQueue是线程不安全的,PriorityBlockingQueue是线程安全的,下面一起来看一下,希望对大家有帮助。
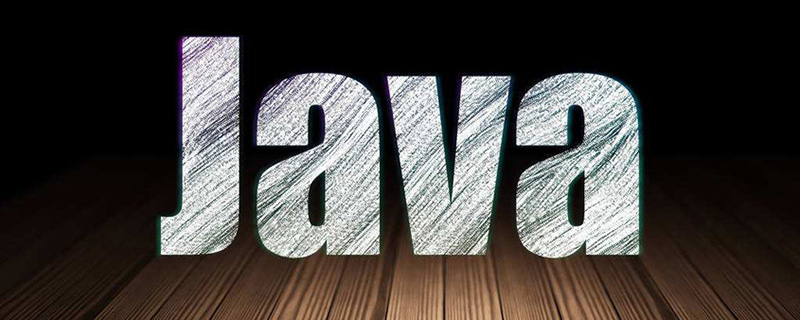
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于java锁的相关问题,包括了独占锁、悲观锁、乐观锁、共享锁等等内容,下面一起来看一下,希望对大家有帮助。
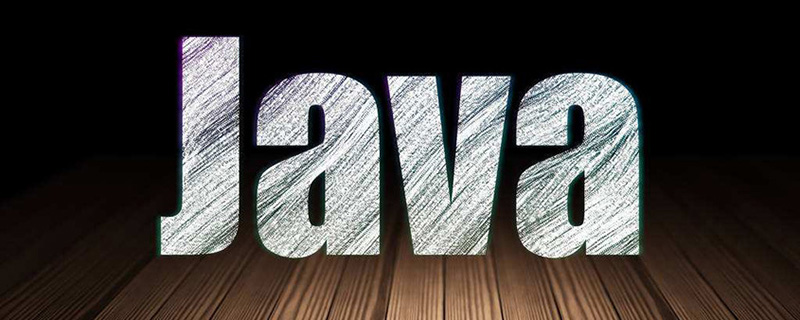
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于多线程的相关问题,包括了线程安装、线程加锁与线程不安全的原因、线程安全的标准类等等内容,希望对大家有帮助。
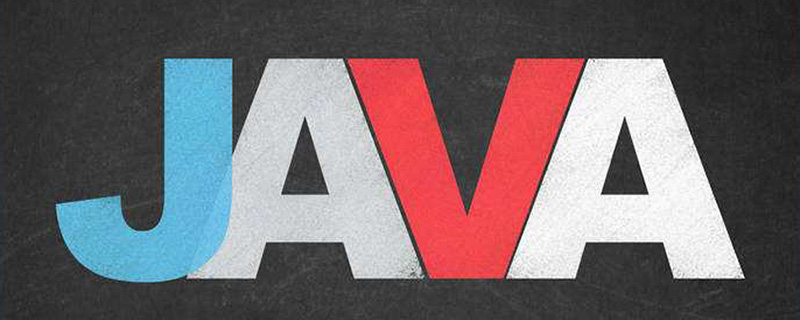
本篇文章给大家带来了关于Java的相关知识,其中主要介绍了关于关键字中this和super的相关问题,以及他们的一些区别,下面一起来看一下,希望对大家有帮助。
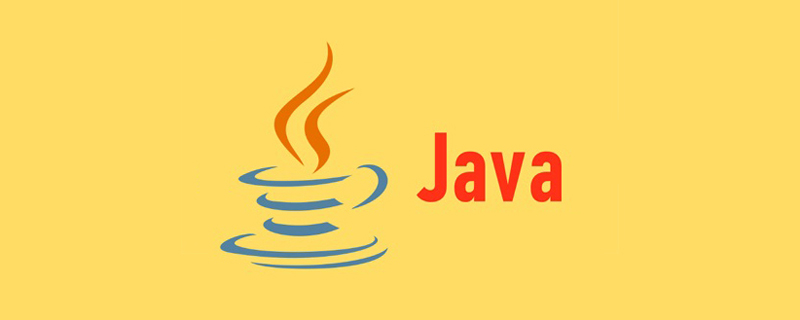
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于枚举的相关问题,包括了枚举的基本操作、集合类对枚举的支持等等内容,下面一起来看一下,希望对大家有帮助。
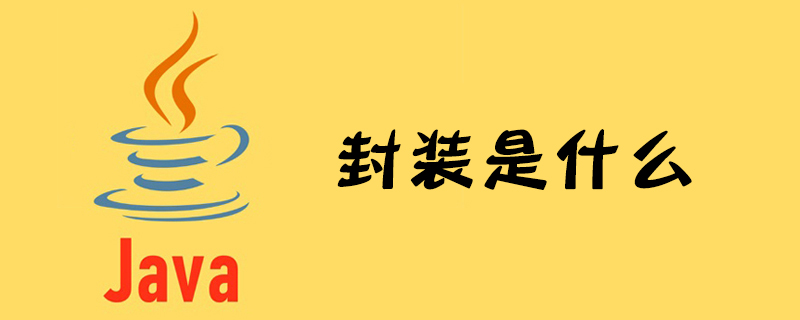
封装是一种信息隐藏技术,是指一种将抽象性函式接口的实现细节部分包装、隐藏起来的方法;封装可以被认为是一个保护屏障,防止指定类的代码和数据被外部类定义的代码随机访问。封装可以通过关键字private,protected和public实现。
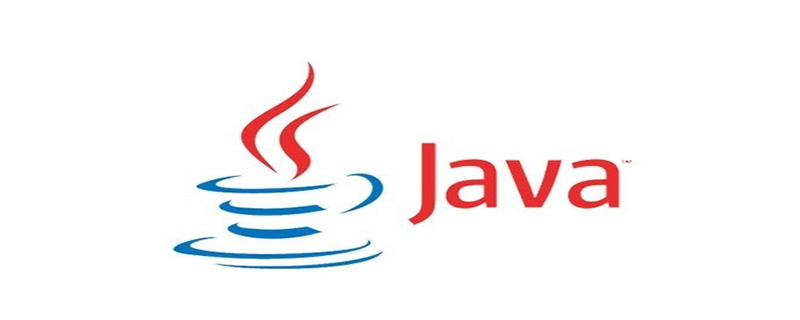
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于平衡二叉树(AVL树)的相关知识,AVL树本质上是带了平衡功能的二叉查找树,下面一起来看一下,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor
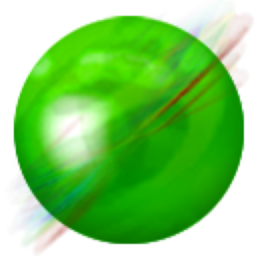
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
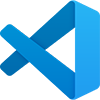
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
