How to use the radix sorting algorithm in C
The radix sorting algorithm is a non-comparative sorting algorithm that divides the elements to be sorted into a finite set of digits to complete the sorting. In C, we can use the radix sort algorithm to sort a set of integers. Below we will discuss in detail how to implement the radix sort algorithm, with specific code examples.
- Algorithm idea
The idea of the radix sorting algorithm is to divide the elements to be sorted into a limited set of digital bits, and then sort the elements on each bit in turn. After the sorting on each bit is completed, the elements are reorganized according to the order of that bit, and then the sorting of the next bit is continued until all bits are sorted. - Specific implementation steps
(1) First, we need to determine the number of digits in the maximum value among all elements to be sorted. This will determine how many rounds of sorting we need to do.
(2) Then, we need to create an auxiliary array and a count array. The auxiliary array is used to store temporary results during the sorting process, and the counting array is used to record the number of occurrences of each number.
(3) Next, we need to perform multiple rounds of sorting. Each round of sorting reorganizes the array according to the current bit size.
(4) In each round of sorting, we need to traverse the array to be sorted, use the current bit value of each element as an index, and put the elements into the corresponding bucket.
(5) Then, we need to count the number of elements in each bucket, which can be recorded using a counting array.
(6) Next, we need to determine the position of the elements in each bucket in the auxiliary array by counting the array. This can be determined by counting the prefix sum of elements in the array.
(7) Finally, we overwrite the elements in the auxiliary array into the array to be sorted to complete a round of sorting.
(8) Repeat steps (3) to (7) until all bits are sorted.
- Code example
The following is a code example that uses C to implement the radix sorting algorithm:
#include <iostream> #include <vector> using namespace std; void radixSort(vector<int>& arr) { int maxVal = *max_element(arr.begin(), arr.end()); int digit = 1; vector<int> temp(arr.size()); while (maxVal / digit > 0) { vector<int> count(10, 0); for (int i = 0; i < arr.size(); i++) { count[(arr[i] / digit) % 10]++; } for (int i = 1; i < 10; i++) { count[i] += count[i - 1]; } for (int i = arr.size() - 1; i >= 0; i--) { temp[count[(arr[i] / digit) % 10] - 1] = arr[i]; count[(arr[i] / digit) % 10]--; } for (int i = 0; i < arr.size(); i++) { arr[i] = temp[i]; } digit *= 10; } } int main() { vector<int> arr = { 170, 45, 75, 90, 802, 24, 2, 66 }; radixSort(arr); cout << "排序结果:"; for (int i = 0; i < arr.size(); i++) { cout << arr[i] << " "; } cout << endl; return 0; }
In the above example code, we first find the array to be sorted The maximum value to determine how many rounds of sorting are required. Then we created an auxiliary array and a count array. Next, we perform multiple rounds of sorting to reassemble the array according to the current bit size. Finally, we output the sorted results.
Summary:
Through the radix sorting algorithm, we can sort a set of integers in C. The core idea of the radix sorting algorithm is to divide the elements to be sorted into a limited set of numerical bits, and then sort the elements on each bit in turn. This non-comparative sorting algorithm can effectively handle the sorting problem of a set of integers.
The above is the detailed content of How to use radix sort algorithm in C++. For more information, please follow other related articles on the PHP Chinese website!
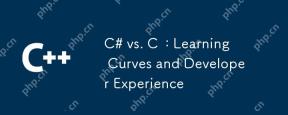
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
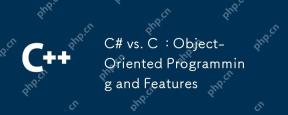
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
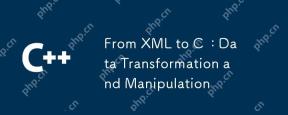
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
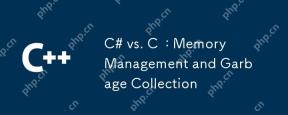
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
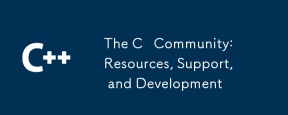
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
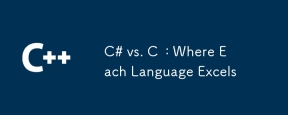
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
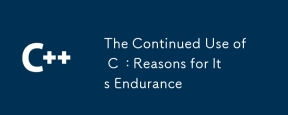
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
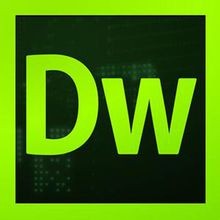
Dreamweaver CS6
Visual web development tools
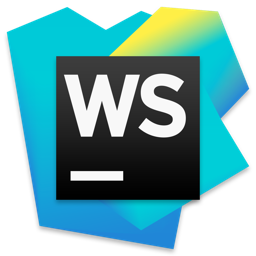
WebStorm Mac version
Useful JavaScript development tools
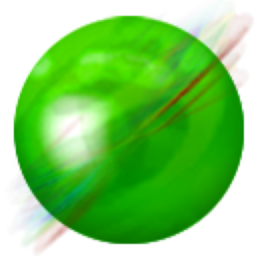
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor