


How to use MongoDB to implement data recommendation and personalization functions
How to use MongoDB to implement data recommendation and personalization functions
Overview:
With the development of the Internet, recommendation systems and personalization functions play an important role in user experience and plays an important role in business value. MongoDB is a flexible and easy-to-use non-relational database. Compared with other traditional relational databases, it has unique advantages in the implementation of recommendation and personalization functions. This article will introduce how to use MongoDB to implement data recommendation and personalization functions, and provide specific code examples.
- Data model design:
Before using MongoDB to implement recommendation and personalization functions, you first need to design and define the data model. For recommendation systems, a common data model is a matrix model based on user behavior and item attributes. In MongoDB, users and items can be represented by documents, where the user document contains the user's ID and a list of favorite item IDs, and the item document contains the item's ID and attribute information of the item.
The sample code is as follows:
// 用户文档 { "_id": "user1", "preferences": ["item1", "item2", "item3"] } // 物品文档 { "_id": "item1", "name": "item1", "category": "category1" }
- Data insertion and query:
Next, we need to insert the actual data into MongoDB and use query operations to Get recommendations and personalized results. When inserting data, we can use theinsertOne
andinsertMany
methods to insert single documents and multiple documents. When querying data, we can use thefind
method to perform the query, and implement sorting through methods such assort
,limit
, andskip
, paging and offset.
The sample code is as follows:
// 插入用户文档 db.users.insertOne({ "_id": "user1", "preferences": ["item1", "item2", "item3"] }) // 插入物品文档 db.items.insertOne({ "_id": "item1", "name": "item1", "category": "category1" }) // 查询用户喜好的前3个物品 db.users.findOne({ "_id": "user1" }, { "preferences": { "$slice": 3 } })
- Recommendation and personalization algorithm:
Through basic query operations of MongoDB, we can implement some simple recommendation and personalization functions , such as recommending and displaying items that may be of interest to users. But for more complex recommendation and personalization algorithms, we may need to use some additional tools or libraries to implement them. Common recommendation and personalization algorithms include collaborative filtering-based recommendation algorithms and content-based recommendation algorithms, which can be implemented through MongoDB query operations.
The sample code is as follows:
// 基于协同过滤的推荐算法 // 根据用户的喜好物品,找到与其相似的其他用户 var similarUsers = db.users.find({ "preferences": { "$in": ["item1"] } }) // 根据相似用户的喜好物品,推荐给当前用户可能感兴趣的物品 var recommendedItems = db.items.find({ "_id": { "$nin": ["item1", "item2", "item3"] }, "category": { "$in": ["category1"] } }) // 基于内容的推荐算法 // 根据当前用户的喜好物品,推荐相似的物品 var similarItems = db.items.find({ "category": { "$in": ["category1"] } }) // 推荐给用户相似物品 var recommendedItems = db.items.find({ "_id": { "$nin": ["item1", "item2", "item3"] }, "category": { "$in": ["category1"] } })
Summary:
Through MongoDB, we can implement data recommendation and personalization functions. When designing a data model, we can represent users and items through documents. When inserting and querying data, we can use MongoDB's insert and query operations to achieve this. For more complex recommendation and personalization algorithms, we can implement them through MongoDB query operations. But it should be noted that for large-scale data sets and complex algorithms, we may need to use some additional tools or libraries to process them. I hope this article can provide some reference and help for readers in using MongoDB to implement data recommendation and personalization functions.
(Note: The above code is only an example. When used in actual use, please make corresponding adjustments according to specific needs and data models.)
The above is the detailed content of How to use MongoDB to implement data recommendation and personalization functions. For more information, please follow other related articles on the PHP Chinese website!
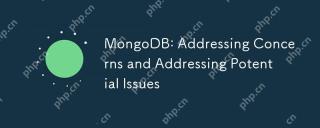
Common problems with MongoDB include data consistency, query performance, and security. The solutions are: 1) Use write and read attention mechanisms to ensure data consistency; 2) Optimize query performance through indexing, aggregation pipelines and sharding; 3) Use encryption, authentication and audit measures to improve security.
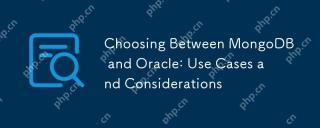
MongoDB is suitable for processing large-scale, unstructured data, and Oracle is suitable for scenarios that require strict data consistency and complex queries. 1.MongoDB provides flexibility and scalability, suitable for variable data structures. 2. Oracle provides strong transaction support and data consistency, suitable for enterprise-level applications. Data structure, scalability and performance requirements need to be considered when choosing.
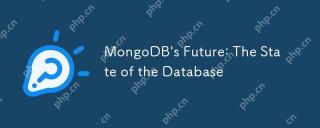
MongoDB's future is full of possibilities: 1. The development of cloud-native databases, 2. The fields of artificial intelligence and big data are focused, 3. The improvement of security and compliance. MongoDB continues to advance and make breakthroughs in technological innovation, market position and future development direction.
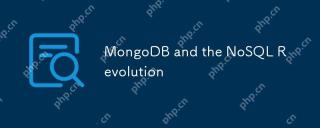
MongoDB is a document-based NoSQL database designed to provide high-performance, scalable and flexible data storage solutions. 1) It uses BSON format to store data, which is suitable for processing semi-structured or unstructured data. 2) Realize horizontal expansion through sharding technology and support complex queries and data processing. 3) Pay attention to index optimization, data modeling and performance monitoring when using it to give full play to its advantages.
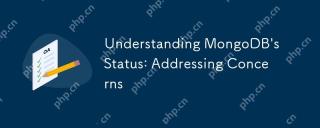
MongoDB is suitable for project needs, but it needs to be used optimized. 1) Performance: Optimize indexing strategies and use sharding technology. 2) Security: Enable authentication and data encryption. 3) Scalability: Use replica sets and sharding technologies.
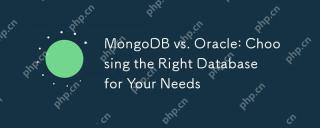
MongoDB is suitable for unstructured data and high scalability requirements, while Oracle is suitable for scenarios that require strict data consistency. 1.MongoDB flexibly stores data in different structures, suitable for social media and the Internet of Things. 2. Oracle structured data model ensures data integrity and is suitable for financial transactions. 3.MongoDB scales horizontally through shards, and Oracle scales vertically through RAC. 4.MongoDB has low maintenance costs, while Oracle has high maintenance costs but is fully supported.
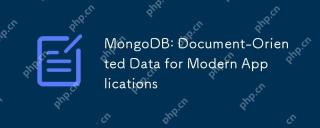
MongoDB has changed the way of development with its flexible documentation model and high-performance storage engine. Its advantages include: 1. Patternless design, allowing fast iteration; 2. The document model supports nesting and arrays, enhancing data structure flexibility; 3. The automatic sharding function supports horizontal expansion, suitable for large-scale data processing.
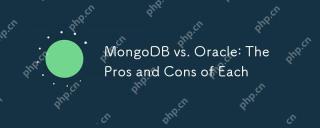
MongoDB is suitable for projects that iterate and process large-scale unstructured data quickly, while Oracle is suitable for enterprise-level applications that require high reliability and complex transaction processing. MongoDB is known for its flexible document storage and efficient read and write operations, suitable for modern web applications and big data analysis; Oracle is known for its strong data management capabilities and SQL support, and is widely used in industries such as finance and telecommunications.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
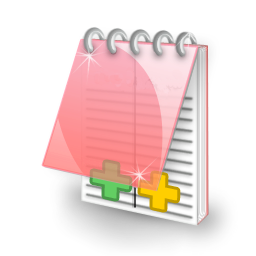
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Chinese version
Chinese version, very easy to use
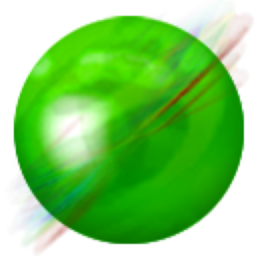
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
