


PHP email detection: Determine whether the email has been sent successfully.
PHP Email Detection: Determine whether the email has been sent successfully.
When developing web applications, it is often necessary to send emails to communicate with users. Whether it is registration confirmation, password reset or notification, the email function is an indispensable part. However, sometimes we cannot ensure whether the email is actually sent successfully, so we need to perform email detection and determine whether the email has been sent successfully. This article will introduce how to use PHP to implement this function.
1. Use SMTP server to send emails
First of all, we need to use SMTP server to send emails, because the SMTP protocol provides a reliable email sending mechanism. In PHP, we can use the SMTP class library to implement this function.
require 'path/to/phpmailer/autoload.php'; use PHPMailerPHPMailerPHPMailer; use PHPMailerPHPMailerException; $mail = new PHPMailer(true); try { $mail->SMTPDebug = 0; // Enable verbose debug output $mail->isSMTP(); // Set mailer to use SMTP $mail->Host = 'smtp.example.com'; // Specify main and backup SMTP servers $mail->SMTPAuth = true; // Enable SMTP authentication $mail->Username = 'your-email@example.com'; // SMTP username $mail->Password = 'your-email-password'; // SMTP password $mail->SMTPSecure = 'tls'; // Enable TLS encryption, `ssl` also accepted $mail->Port = 587; // TCP port to connect to $mail->setFrom('your-email@example.com', 'Your Name'); $mail->addAddress('recipient@example.com', 'Recipient Name'); $mail->isHTML(true); // Set email format to HTML $mail->Subject = 'Test Subject'; $mail->Body = 'This is a test email.'; $mail->send(); echo 'Email has been sent.'; } catch (Exception $e) { echo 'Email could not be sent. Error: ', $mail->ErrorInfo; }
This code uses the PHPMailer class library, configures the relevant information of the SMTP server, and sends a test email.
2. Email Status Detection
Sending an email does not mean that the email has actually been received, so we need to use email status detection to determine whether the email has been successfully sent. In PHP, we can get the mail status through the response of the SMTP server.
if ($mail->send()) { $response = $mail->getSMTPInstance()->getLastResponse(); if (preg_match('/^250/', $response)) { echo 'Email has been sent.'; } else { echo 'Email could not be sent. Response: ' . $response; } } else { echo 'Email could not be sent. Error: ', $mail->ErrorInfo; }
After sending the email, this code obtains the response from the SMTP server through the getSMTPInstance()
method, and uses regular expressions to determine whether the response begins with 250
. If so, it means the email was sent successfully.
3. Email delivery status feedback
In addition to judging whether the email is sent successfully through the response of the SMTP server, we can also obtain more detailed information through email delivery status feedback. In PHP, this can be achieved using a return receipt email.
$mail->addCustomHeader('Return-Receipt-To: your-email@example.com'); $mail->addCustomHeader('Disposition-Notification-To: your-email@example.com'); if ($mail->send()) { echo 'Email has been sent.'; } else { echo 'Email could not be sent.'; }
This code adds information about the receipt email through the addCustomHeader()
method before sending the email. When the recipient opens the email and confirms reading, we will receive a receipt email through which we can confirm whether the email has been received and read.
Summary:
Through the above method, we can determine whether the email has been sent successfully. In actual development, we can choose appropriate methods for email detection based on different needs to ensure the reliability and timeliness of emails.
(Note: The email address and password in the above example should be replaced with the real email address and password, and make sure the SMTP server is configured correctly.)
The above is the detailed content of PHP email detection: Determine whether the email has been sent successfully.. For more information, please follow other related articles on the PHP Chinese website!
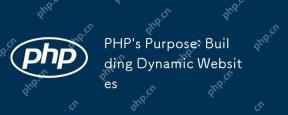
PHP is used to build dynamic websites, and its core functions include: 1. Generate dynamic content and generate web pages in real time by connecting with the database; 2. Process user interaction and form submissions, verify inputs and respond to operations; 3. Manage sessions and user authentication to provide a personalized experience; 4. Optimize performance and follow best practices to improve website efficiency and security.

PHP uses MySQLi and PDO extensions to interact in database operations and server-side logic processing, and processes server-side logic through functions such as session management. 1) Use MySQLi or PDO to connect to the database and execute SQL queries. 2) Handle HTTP requests and user status through session management and other functions. 3) Use transactions to ensure the atomicity of database operations. 4) Prevent SQL injection, use exception handling and closing connections for debugging. 5) Optimize performance through indexing and cache, write highly readable code and perform error handling.
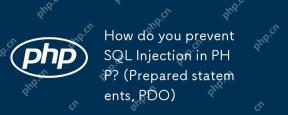
Using preprocessing statements and PDO in PHP can effectively prevent SQL injection attacks. 1) Use PDO to connect to the database and set the error mode. 2) Create preprocessing statements through the prepare method and pass data using placeholders and execute methods. 3) Process query results and ensure the security and performance of the code.
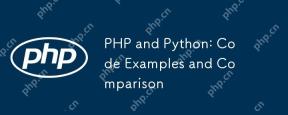
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
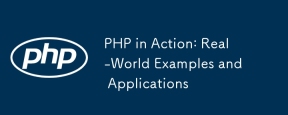
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
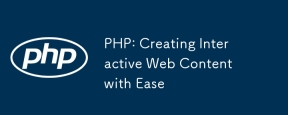
PHP makes it easy to create interactive web content. 1) Dynamically generate content by embedding HTML and display it in real time based on user input or database data. 2) Process form submission and generate dynamic output to ensure that htmlspecialchars is used to prevent XSS. 3) Use MySQL to create a user registration system, and use password_hash and preprocessing statements to enhance security. Mastering these techniques will improve the efficiency of web development.
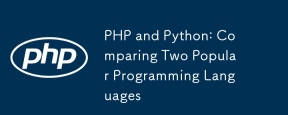
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
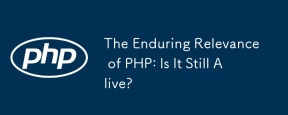
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
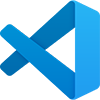
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
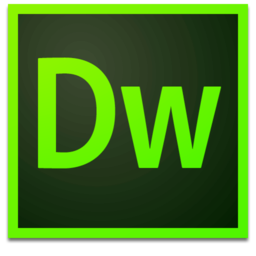
Dreamweaver Mac version
Visual web development tools

Atom editor mac version download
The most popular open source editor