


How to use Java technology to implement high-performance database search strategies and implementation methods?
Introduction:
In modern software systems, the database is a very important component. As the amount of data increases and users' requirements for speed and performance continue to increase, how to efficiently search the database has become a key issue. This article will introduce how to use Java technology to implement high-performance database search strategies and implementation methods, and provide specific code examples.
1. Optimize database search strategy
- Reasonable setting of database index
Index is the key to accelerating database search. When designing a database, indexes should be set appropriately based on actual needs. The selection of indexes should take into account the frequency of queries, the frequency of data updates, and the balance between queries and updates. Generally speaking, primary key and foreign key fields should be used as indexes, and frequently searched fields should also be indexed.
- Optimization of database query statements
Writing efficient database query statements is the key to improving search performance. Here are some ways to optimize query statements:
- Avoid using SELECT * and try to query only the fields you need.
- Use the WHERE clause to filter data to reduce the amount of query data.
- Use the LIMIT clause to limit the size of the result set.
- Avoid unnecessary JOIN operations.
- Use appropriate aggregate functions and GROUP BY to group data.
- Optimization of paging queries
In practical applications, it is often necessary to perform paging queries on large amounts of data. In order to improve performance, you can optimize through the following methods:
- Use appropriate indexes to avoid full table scans.
- Use LIMIT and OFFSET to control the amount of data in each query.
- To avoid repeatedly querying the same data, you can use caching technology to cache results.
2. Implementation method and code examples
The following provides an implementation method of high-performance database search based on Java technology, and uses code examples to illustrate the specific implementation.
- Use JDBC to connect to the database
Connecting to the database through JDBC is a common way to access the database using Java technology. The following is a simple example:
// 导入 Java.sql 包 import java.sql.*; public class DatabaseSearch { public static void main(String[] args) { Connection conn = null; try { // 加载数据库驱动 Class.forName("com.mysql.jdbc.Driver"); // 连接到数据库 conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/test", "root", "password"); // 执行查询语句 Statement stmt = conn.createStatement(); ResultSet rs = stmt.executeQuery("SELECT * FROM users"); // 处理查询结果 while (rs.next()) { System.out.println(rs.getString("username")); } // 关闭数据库连接 rs.close(); stmt.close(); conn.close(); } catch (ClassNotFoundException e) { e.printStackTrace(); } catch (SQLException e) { e.printStackTrace(); } } }
- Using the MyBatis ORM framework
MyBatis is an open source Java persistence framework that simplifies database operations and improves performance. The following is an example of using MyBatis:
// 导入 MyBatis 包 import org.apache.ibatis.session.*; public class DatabaseSearch { public static void main(String[] args) { try { // 加载 MyBatis 配置文件 InputStream inputStream = Resources.getResourceAsStream("mybatis-config.xml"); SqlSessionFactory sessionFactory = new SqlSessionFactoryBuilder().build(inputStream); // 获取数据库会话 SqlSession session = sessionFactory.openSession(); // 执行数据库查询 List<User> users = session.selectList("UserMapper.getAll"); // 处理查询结果 for (User user : users) { System.out.println(user.getUsername()); } // 关闭会话 session.close(); } catch (IOException e) { e.printStackTrace(); } } }
In the above example, mybatis-config.xml is the configuration file of MyBatis, and UserMapper is the interface that defines database mapping and query.
Conclusion:
This article introduces how to use Java technology to implement high-performance database search strategies and implementation methods. By properly setting database indexes, optimizing query statements, and using appropriate paging query methods, the performance of database search can be greatly improved. Through Java's JDBC and MyBatis framework, we can easily implement these optimization strategies and provide specific code examples.
It should be noted that further performance tuning and optimization measures may be required for different application scenarios and data volumes. Therefore, in practical applications, it is necessary to comprehensively consider the specific situation and select appropriate database search strategies and implementation methods.
The above is the detailed content of How to use Java technology to implement high-performance database search strategies and implementation methods?. For more information, please follow other related articles on the PHP Chinese website!
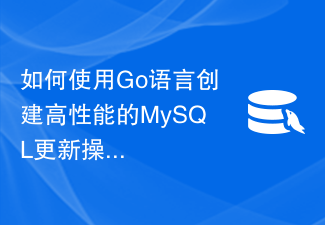
在现代的Web应用程序中,数据库是不可避免的一部分。MySQL是一种常用的关系型数据库管理系统,与许多编程语言兼容。Go语言是一种自带并发性能且易于编写的编程语言。在本文中,我们将介绍如何结合Go语言和MySQL创建高性能的数据库更新操作。连接MySQL数据库在开始之前,您需要确保已经安装并配置了MySQL数据库。我们使用Go语言内置的MySQL驱动程序来连
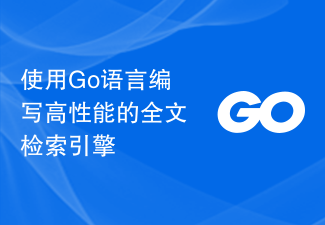
随着互联网时代的到来,全文检索引擎越来越受到人们的重视。在无数的网页、文档和数据中,我们需要快速地找到所需的内容,这就需要使用高效的全文检索引擎。Go语言是一种以效率而闻名的编程语言,它的设计目标是提高代码的执行效率和性能。因此,使用Go语言编写全文检索引擎可以大大提高其运行效率和性能。本文将介绍如何使用Go语言编写高性能的全文检索引擎。一、理解全文检索引擎
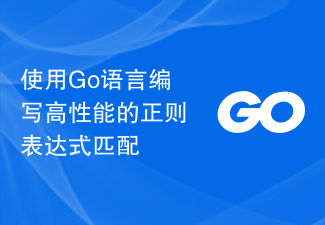
随着数据量的不断增大,正则表达式匹配成为了程序中常用的操作之一。而在Go语言中,由于其天然的并行ism,以及与底层系统的交互性和高效性,使得Go语言的正则表达式匹配极具优势。那么如何使用Go语言编写高性能的正则表达式匹配呢?一、了解正则表达式在使用正则表达式前,我们首先需要了解正则表达式,了解其基本语法规则以及常用的匹配字符,使我们能够在编写正则表达式时更加
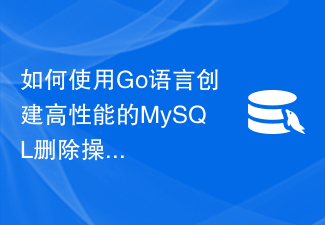
随着数据量的增加,数据库中的删除操作往往会成为程序的瓶颈。为了提高删除操作的性能,可以考虑使用Go语言。Go语言是一种高效的编程语言,能够很好地支持并发和网络编程,同时也有很强的类型检查功能,可靠性和安全性都很高。下面,我们将介绍如何使用Go语言创建高性能的MySQL删除操作。使用Go的并发机制首先,可以使用Go语言的goroutine并发机制来加速删除操作
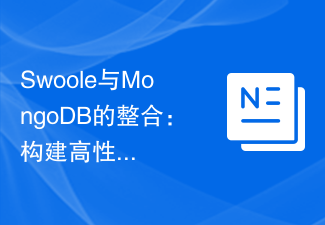
在现代企业应用程序开发中,需要处理海量数据和高并发的访问请求。为了满足这些需求,开发人员需要使用高性能的数据库系统,以确保系统的稳定性和可扩展性。本文将介绍如何使用Swoole和MongoDB构建高性能的文档数据库系统。Swoole是一个基于PHP语言开发的异步网络通信框架,它能够大大提高PHP应用程序的性能和并发能力。MongoDB是一种流行的文档数据库,
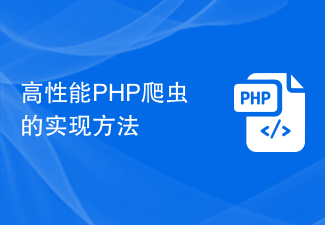
随着互联网的发展,网页中的信息量越来越大,越来越深入,很多人需要从海量的数据中快速地提取出自己需要的信息。此时,爬虫就成了重要的工具之一。本文将介绍如何使用PHP编写高性能的爬虫,以便快速准确地从网络中获取所需的信息。一、了解爬虫基本原理爬虫的基本功能就是模拟浏览器去访问网页,并获取其中的特定信息。它可以模拟用户在网页浏览器中的一系列操作,比如向服务器发送请
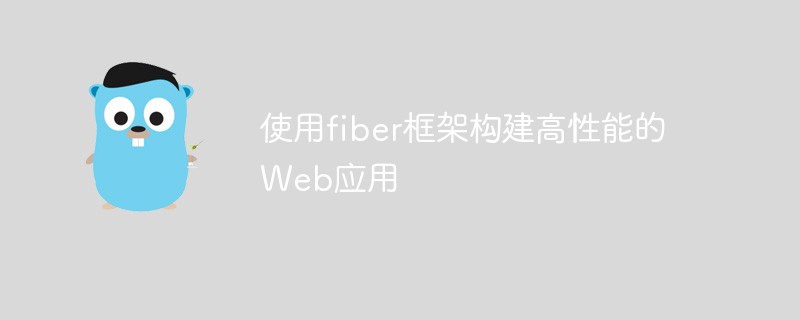
随着互联网的快速发展,越来越多的企业和个人开始涉足Web应用的开发领域,而如何构建高性能的Web应用已经成为人们关注的焦点之一。对于Web应用的性能来说,最主要的就是服务器端的处理能力和响应时间。近年来,随着技术的发展,有许多新的框架被提了出来,其中Fiber框架因其高性能和易用性备受青睐。Fiber是一个轻量级的Go语言Web框架,它的主要特点就是高性能和
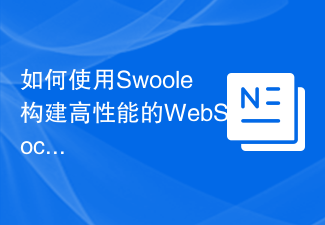
近年来,WebSocket技术在互联网开发中越来越流行,尤其是在实时通信、在线游戏、推送消息等领域。而Swoole作为一款高性能、异步的PHP扩展,可以帮助开发者轻松构建高性能的WebSocket服务器。本文将介绍如何使用Swoole搭建一个高性能的WebSocket服务器。一、安装SwooleSwoole支持PHP5.3~7.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
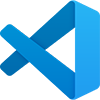
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
