How to use Java technology to achieve high-performance database search?
Abstract:
With the increase in data volume, how to achieve high-performance database search has become an important issue for developers. This article will introduce how to use Java technology to implement high-performance database search and provide specific code examples.
Keywords: Java, high performance, database search
1. Introduction
In today's big data era, the explosive growth of data volume has put forward higher requirements for database search performance. Require. Many application scenarios require fast and accurate retrieval of qualified data from the database. In order to achieve high-performance database search, we need to give full play to the advantages and features of Java technology. In this article, we will focus on the implementation of the following aspects.
2. Optimize database query statements
Database optimization is the basis for improving database search performance. We can optimize database query statements through the following methods:
- Use indexes: Creating appropriate indexes in database tables can greatly improve query performance. Indexes allow the database engine to locate the data that needs to be searched more quickly.
- Avoid full table scan: Full table scan is a very inefficient method. Try to avoid full table scan in large data tables. Full table scans can be avoided by creating appropriate indexes and using optimized query conditions.
- Use appropriate connection methods: When performing multi-table queries, choosing an appropriate connection method can improve query performance. You can choose to use inner join, left join or right join according to the actual situation.
3. Use Java technology to achieve high-performance search
After optimizing the database query statement, we can further use Java technology to improve search performance. The following introduces several commonly used Java technologies to achieve high-performance database search.
- Use connection pool
The connection pool can improve the database connection reuse rate and reduce the cost of each database connection. There are many connection pool implementations in Java, such as Apache Commons DBCP, C3P0, etc. The connection pool can reduce the cost of connection creation and destruction by pre-creating a certain number of database connection objects and reusing them when needed. - Batch query data
For batch query scenarios, we can use the batch query method in Java to improve performance. The database implements a batch query interface, and we can reduce database communication overhead by issuing multiple query requests at one time. For example, use JDBC's addBatch() and executeBatch() methods to implement batch queries. - Caching query results
For some popular queries and repeated query results, we can cache them in memory to improve query performance. You can use caching frameworks in Java such as Ehcache, Redis, etc., or implement simple caching functions manually.
4. Code Example
The following is a sample code that uses Java technology to implement high-performance database search:
// 使用连接池 DataSource dataSource = new BasicDataSource(); ((BasicDataSource) dataSource).setUrl("jdbc:mysql://localhost:3306/mydb"); ((BasicDataSource) dataSource).setUsername("root"); ((BasicDataSource) dataSource).setPassword("password"); Connection conn = dataSource.getConnection(); Statement stmt = conn.createStatement(); // 批量查询数据 String[] queries = {"SELECT * FROM table1", "SELECT * FROM table2"}; List<ResultSet> results = new ArrayList<>(); for (String query : queries) { results.add(stmt.executeQuery(query)); } // 缓存查询结果 Cache<String, ResultSet> cache = Ehcache.createCache("queryCache"); for (ResultSet result : results) { cache.put(key, result); } conn.close();
Through the above code example, we can see how to use connections Pools, batch queries, and caching to enable high-performance database searches. These technologies can effectively reduce database connection overhead, database communication overhead, and reduce repeated queries.
Summary:
This article introduces how to use Java technology to implement high-performance database search, and provides specific code examples. By optimizing query statements, using connection pools, querying data in batches, and caching query results, the performance of database searches can be greatly improved. When faced with large amounts of data and high concurrency, rational application of these technologies can effectively improve system performance.
The above is the detailed content of How to implement high-performance database search using Java technology?. For more information, please follow other related articles on the PHP Chinese website!
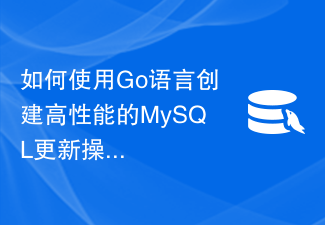
在现代的Web应用程序中,数据库是不可避免的一部分。MySQL是一种常用的关系型数据库管理系统,与许多编程语言兼容。Go语言是一种自带并发性能且易于编写的编程语言。在本文中,我们将介绍如何结合Go语言和MySQL创建高性能的数据库更新操作。连接MySQL数据库在开始之前,您需要确保已经安装并配置了MySQL数据库。我们使用Go语言内置的MySQL驱动程序来连
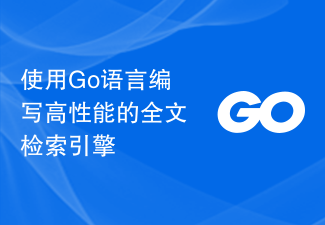
随着互联网时代的到来,全文检索引擎越来越受到人们的重视。在无数的网页、文档和数据中,我们需要快速地找到所需的内容,这就需要使用高效的全文检索引擎。Go语言是一种以效率而闻名的编程语言,它的设计目标是提高代码的执行效率和性能。因此,使用Go语言编写全文检索引擎可以大大提高其运行效率和性能。本文将介绍如何使用Go语言编写高性能的全文检索引擎。一、理解全文检索引擎
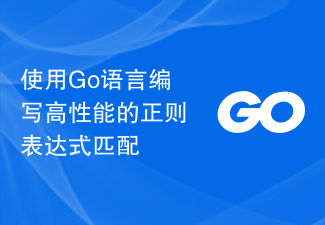
随着数据量的不断增大,正则表达式匹配成为了程序中常用的操作之一。而在Go语言中,由于其天然的并行ism,以及与底层系统的交互性和高效性,使得Go语言的正则表达式匹配极具优势。那么如何使用Go语言编写高性能的正则表达式匹配呢?一、了解正则表达式在使用正则表达式前,我们首先需要了解正则表达式,了解其基本语法规则以及常用的匹配字符,使我们能够在编写正则表达式时更加
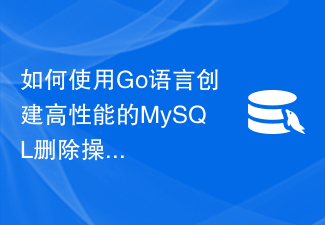
随着数据量的增加,数据库中的删除操作往往会成为程序的瓶颈。为了提高删除操作的性能,可以考虑使用Go语言。Go语言是一种高效的编程语言,能够很好地支持并发和网络编程,同时也有很强的类型检查功能,可靠性和安全性都很高。下面,我们将介绍如何使用Go语言创建高性能的MySQL删除操作。使用Go的并发机制首先,可以使用Go语言的goroutine并发机制来加速删除操作
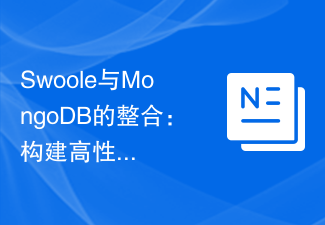
在现代企业应用程序开发中,需要处理海量数据和高并发的访问请求。为了满足这些需求,开发人员需要使用高性能的数据库系统,以确保系统的稳定性和可扩展性。本文将介绍如何使用Swoole和MongoDB构建高性能的文档数据库系统。Swoole是一个基于PHP语言开发的异步网络通信框架,它能够大大提高PHP应用程序的性能和并发能力。MongoDB是一种流行的文档数据库,
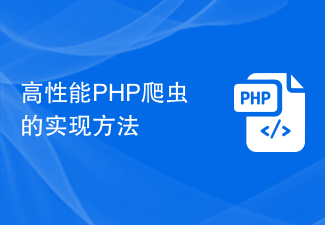
随着互联网的发展,网页中的信息量越来越大,越来越深入,很多人需要从海量的数据中快速地提取出自己需要的信息。此时,爬虫就成了重要的工具之一。本文将介绍如何使用PHP编写高性能的爬虫,以便快速准确地从网络中获取所需的信息。一、了解爬虫基本原理爬虫的基本功能就是模拟浏览器去访问网页,并获取其中的特定信息。它可以模拟用户在网页浏览器中的一系列操作,比如向服务器发送请
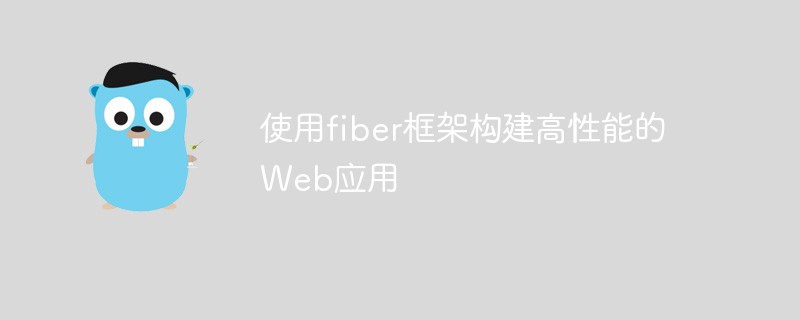
随着互联网的快速发展,越来越多的企业和个人开始涉足Web应用的开发领域,而如何构建高性能的Web应用已经成为人们关注的焦点之一。对于Web应用的性能来说,最主要的就是服务器端的处理能力和响应时间。近年来,随着技术的发展,有许多新的框架被提了出来,其中Fiber框架因其高性能和易用性备受青睐。Fiber是一个轻量级的Go语言Web框架,它的主要特点就是高性能和
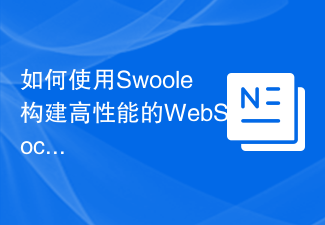
近年来,WebSocket技术在互联网开发中越来越流行,尤其是在实时通信、在线游戏、推送消息等领域。而Swoole作为一款高性能、异步的PHP扩展,可以帮助开发者轻松构建高性能的WebSocket服务器。本文将介绍如何使用Swoole搭建一个高性能的WebSocket服务器。一、安装SwooleSwoole支持PHP5.3~7.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
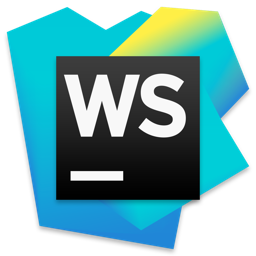
WebStorm Mac version
Useful JavaScript development tools
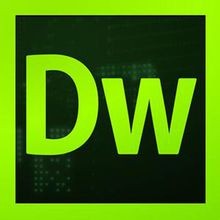
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use
