


Discussion on Java implementation techniques of high-performance database search algorithms
Discussion on Java implementation techniques of high-performance database search algorithms
Abstract:
With the advent of the big data era, the performance requirements for database search algorithms are increasing The higher. This article will focus on Java implementation techniques for high-performance database search algorithms and provide specific code examples.
- Introduction
Database search is the process of extracting and obtaining information stored in a database. When processing large amounts of data, the performance of search algorithms is critical because they directly affect the response time and throughput of the database. - Index data structure
Index is the key to improving database search efficiency. Common index data structures include hash tables, B-trees, and inverted indexes. These data structures have different advantages and applicable scenarios, and we need to choose the appropriate index structure according to specific needs. - Search algorithm
When implementing database search algorithms, we can use a variety of algorithms, such as linear search, binary search, hash search, and inverted index. The implementation techniques of several commonly used high-performance search algorithms will be discussed below.
3.1. Linear search
Linear search is the simplest search algorithm, which compares elements in the database one by one until a matching element is found. The time complexity of this algorithm is O(n), which is suitable for small-scale databases.
Sample code:
public class LinearSearch { public static int linearSearch(int[] arr, int target) { for (int i = 0; i < arr.length; i++) { if (arr[i] == target) { return i; } } return -1; } }
3.2. Binary search
Binary search is an efficient search algorithm, which requires that the database to be searched must be ordered. The algorithm splits the database in half and gradually narrows the search range until the target element is found or the search range is empty. The time complexity of this algorithm is O(logn).
Sample code:
import java.util.Arrays; public class BinarySearch { public static int binarySearch(int[] arr, int target) { Arrays.sort(arr); // 先对数组进行排序 int left = 0; int right = arr.length - 1; while (left <= right) { int mid = (left + right) / 2; if (arr[mid] == target) { return mid; } else if (arr[mid] < target) { left = mid + 1; } else { right = mid - 1; } } return -1; } }
3.3. Hash search
Hash search uses a hash function to map elements in the database to a fixed-size hash table, and through the hash Hash conflict resolution algorithm to handle hash conflicts. This allows you to quickly locate the element you are searching for. The average time complexity of a hash search is O(1).
Sample code:
import java.util.HashMap; import java.util.Map; public class HashSearch { public static int hashSearch(int[] arr, int target) { Map<Integer, Integer> map = new HashMap<>(); for (int i = 0; i < arr.length; i++) { map.put(arr[i], i); } return map.getOrDefault(target, -1); } }
3.4. Inverted index
The inverted index is a keyword-based index structure that maps keywords to database records containing the keyword . Inverted indexes are suitable for efficient full-text search operations.
Sample code:
import java.util.ArrayList; import java.util.HashMap; import java.util.List; import java.util.Map; public class InvertedIndex { public static Map<String, List<Integer>> createIndex(String[] documents) { Map<String, List<Integer>> index = new HashMap<>(); for (int i = 0; i < documents.length; i++) { String[] words = documents[i].split(" "); for (String word : words) { if (!index.containsKey(word)) { index.put(word, new ArrayList<>()); } index.get(word).add(i); } } return index; } public static List<Integer> search(Map<String, List<Integer>> index, String keyword) { return index.getOrDefault(keyword, new ArrayList<>()); } }
- Experimentation and analysis
By testing the implementation of different search algorithms, we can choose the most appropriate algorithm based on the specific data size and characteristics. In addition, performance can also be improved by optimizing the search algorithm, such as using parallel computing, incremental index update, compressed storage and other technologies.
Conclusion:
This article focuses on the Java implementation techniques of high-performance database search algorithms and provides specific code examples. In practical applications, factors such as data size, data type, and search requirements need to be comprehensively considered to select the most suitable search algorithm and index structure. At the same time, through the implementation of optimization algorithms and indexes, search performance can be further improved.
The above is the detailed content of Discussion on Java implementation techniques of high-performance database search algorithms. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
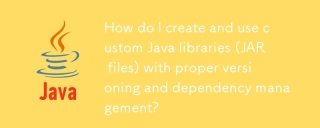
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
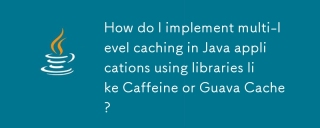
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
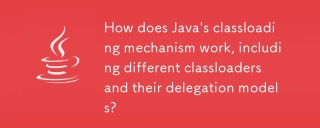
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
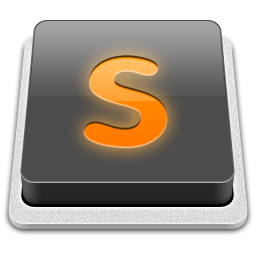
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
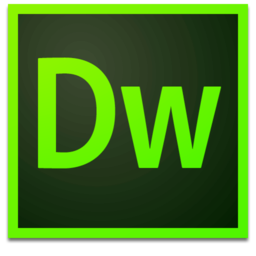
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool