Regular expressions are patterns that contain various characters. We can use regular expressions to search if a string contains a specific pattern.
Here, we will learn to create regular expressions to verify various mathematical formulas. We will use the test() or match() method to check if a specific mathematical formula matches a regular expression
grammar
Users can create regular expressions that accept special mathematical formulas according to the following syntax.
let regex = /^\d+([-+]\d+)*$/g;
The regular expression above only accepts 10 – 13 12 23, just like a mathematical formula.
Regular expression explanation
/ / – It represents the beginning and end of the regular expression.
^ – It represents the beginning of the formula string.
\d – It represents at least one or more digits at the beginning of the formula.
[- ] – Represents the " " and "-" operators in regular expressions.
([- ]\d )* – means that the formula can contain numbers, followed by multiple " " or "-" operators.
$ – It represents the end of the string.
g – It matches all occurrences of the identifier.
Example
In the example below, we create a regular expression that accepts formulas that contain numbers " " or the "-" operator.
Users can observe that the first formula matches the regular expression pattern in the output. The second formula does not match the regular expression pattern because it contains the "*" operator. Also, the third formula is the same as the first, but it contains spaces between the operator and the number, so it doesn't match the regular expression.
<html> <body> <h3 id="Creating-the-regular-expression-to-validate-special-mathematical-formula-in-JavaScript">Creating the regular expression to validate special mathematical formula in JavaScript</h3> <div id = "output"></div> <script> let output = document.getElementById('output'); function matchFormula(formula) { let regex = /^\d+([-+]\d+)*$/g; let isMatch = regex.test(formula); if (isMatch) { output.innerHTML += "The " + formula + " is matching with " + regex + "<br>"; } else { output.innerHTML += "The " + formula + " is not matching with " + regex + "<br>"; } } let formula = "10+20-30-50"; matchFormula(formula); matchFormula("60*70*80"); matchFormula("10 + 20 - 30 - 50") </script> </body> </html>
Regular expressions used in the examples below
We used the /^\d (\s*[- */]\s*\d )*$/g regular expression in the example below. Users can find below an explanation of the regular expressions used.
^\d – It represents at least one digit at the beginning of the formula.
\s* – It represents zero or more spaces.
(\s*[- */]\s*\d )* – means that the formula can contain spaces and multiple times in the same order. operators, spaces and numbers.
Example
In the following example, we call the TestMultiplyFormula() function three times by passing various formulas as parameters. We use the test() method to check if the formula matches the regular expression pattern.
In the output, we can see that the regular expression accepts formulas with "*" and "/" operators and spaces.
<html> <body> <h2 id="Creating-the-regular-expression-i-to-validate-special-mathematical-formula-i-in-JavaScript">Creating the regular expression <i> to validate special mathematical formula </i> in JavaScript.</h2> <div id = "output"> </div> <script> let output = document.getElementById('output'); function TestMultiplyFormula(formula) { let regex = /^\d+(\s*[-+*/]\s*\d+)*$/g; let isMatch = regex.test(formula); if (isMatch) { output.innerHTML += "The " + formula + " is matching with " + regex + "<br>"; } else { output.innerHTML += "The " + formula + " is not matching with " + regex + "<br>"; } } let formula = "12312323+454+ 565 - 09 * 23"; TestMultiplyFormula(formula); TestMultiplyFormula("41*14* 90 *80* 70 + 90"); TestMultiplyFormula("41*14& 90 ^80* 70 + 90"); </script> </body> </html>
This tutorial teaches us to create a regular expression that accepts a special mathematical formula. In both examples, we used the test() method to match a formula against a regular expression. Additionally, we used different regular expression patterns in the two examples.
The above is the detailed content of How to create a regular expression that only accepts special formulas?. For more information, please follow other related articles on the PHP Chinese website!
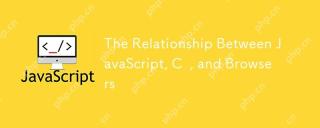
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
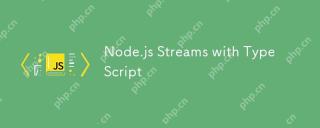
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe
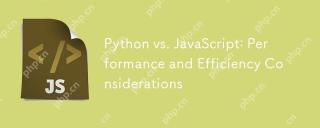
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.
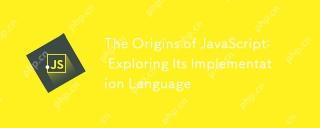
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.
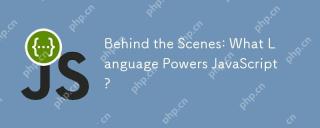
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.
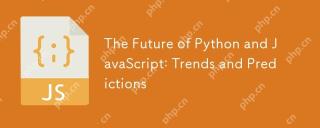
The future trends of Python and JavaScript include: 1. Python will consolidate its position in the fields of scientific computing and AI, 2. JavaScript will promote the development of web technology, 3. Cross-platform development will become a hot topic, and 4. Performance optimization will be the focus. Both will continue to expand application scenarios in their respective fields and make more breakthroughs in performance.
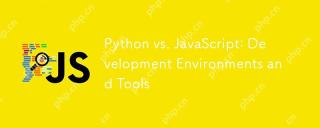
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.
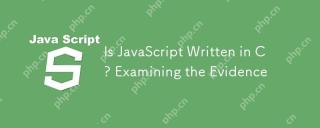
Yes, the engine core of JavaScript is written in C. 1) The C language provides efficient performance and underlying control, which is suitable for the development of JavaScript engine. 2) Taking the V8 engine as an example, its core is written in C, combining the efficiency and object-oriented characteristics of C. 3) The working principle of the JavaScript engine includes parsing, compiling and execution, and the C language plays a key role in these processes.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
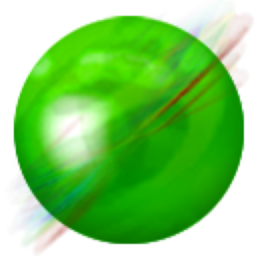
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
