Print out all non-repeating words in two given sentences
In this tutorial, we will identify and print out all non-repeating words in two given sentences. Unrepeated words refer to words that appear only once in two sentences, that is, they do not appear repeatedly in another sentence. This task involves analyzing an input sentence, identifying individual words, and comparing between two sentences to find words that appear only once. The output should be a list of all these words. This task can be accomplished through various programming methods, such as using loops, arrays, or dictionaries.
method
Here are two ways to print out all non-repeating words in two given sentences−
Method 1: Use dictionary
Method 2: Using collections
Method 1: Use dictionary
Using a dictionary, count the number of times each word appears in two phrases. We can then look up the dictionary and print all the words that appear only once. The Dictionary function in C is usually used to output all unique words in two specified sentences. The method involves using a dictionary or hash table data structure to store the frequency of each word in two phrases. We can then iterate through the dictionary and print out terms that appear only once.
grammar
Here is the syntax without the actual code, using dictionary methods in C to print all non-repeating words in two given sentences -
Declare a dictionary to store word frequencies
map<string, int> freqDict;
Enter two sentences as strings
string sentence1 = "first sentence"; string sentence2 = "second sentence";
Split sentences into words and insert into dictionary
istringstream iss (sentence1 + " " + sentence2); string word; while (iss >> word) { freqDict[word]++; }
Traverse the dictionary and print unique words
for (const auto& [word, frequency]: freqDict) { if (frequency == 1) { cout << word << " "; } }
algorithm
In C, this is a trick to use dictionary methods to print step by step all non-duplicate items in two specified sentences -
Step 1 - Create two strings s1 and s2 containing sentences.
Step 2 - Declare an empty unordered map string, int> dict, used to record the frequency of each word in the sentence.
Step 3 − Using C’s string stream class, parse the two phrases to extract words.
Step 4 - For each extracted word, check if it appears in the dictionary. If it is, increase its frequency by one. Otherwise, add it to the dictionary with frequency 1.
Step 5 - After processing both sentences, iterate the dictionary and display all terms with frequency 1. These are words that are not repeated in the two sentences.
Step 6 − The time complexity of this method is O(n),
The Chinese translation ofExample 1
is:Example 1
This code uses an unordered map to store the frequency of each word in the combined phrase. It then loops through the map, adding each word that appears only once to a vector of non-repeating words. Finally, it publishes non-duplicate words. This example implies that the two sentences are hard-coded into the program rather than entered by the user.
#include <iostream> #include <string> #include <unordered_map> #include <sstream> #include <vector> using namespace std; vector<string> getNonRepeatingWords(string sentence1, string sentence2) { // Combine the two sentences into a single string string combined = sentence1 + " " + sentence2; // Create a map to store the frequency of each word unordered_map<string, int> wordFreq; // Use a string stream to extract each word from the combined string stringstream ss(combined); string word; while (ss >> word) { // Increment the frequency of the word in the map wordFreq[word]++; } // Create a vector to store the non-repeating words vector<string> nonRepeatingWords; for (auto& pair : wordFreq) { if (pair.second == 1) { nonRepeatingWords.push_back(pair.first); } } return nonRepeatingWords; } int main() { string sentence1 = "The quick brown fox jumps over the lazy dog"; string sentence2 = "A quick brown dog jumps over a lazy fox"; vector<string> nonRepeatingWords = getNonRepeatingWords(sentence1, sentence2); // Print the non-repeating words for (auto& word : nonRepeatingWords) { cout << word << " "; } cout << endl; return 0; }
Output
a A the The
Method 2: Using collections
This strategy involves using sets to find terms that appear only once in two phrases. We can build term sets for each phrase and then identify the intersection of these sets. Finally, we can iterate over the intersection and output all items that appear only once.
A collection is an associative container that holds different elements in sorted order. We can insert terms from both phrases into the collection and any duplicates will be automatically removed.
grammar
certainly! Following is the syntax you can use in Python to print out all non-repeating words in two given sentences −
Define two sentences as strings
sentence1 = "The fox jumps over dog" sentence2 = "A dog jumps over fox"
Split each sentence into a list of words
words1 = sentence1.split() words2 = sentence2.split()
Create a set from these two word lists
set1 = set(words1) set2 = set(words2)
Find unique words through the intersection of sets
Nonrepeating = set1.symmetric_difference(set2)
Print unique words
for word in non-repeating: print(word)
algorithm
Follow the instructions below to output all non-repeating words in two given sentences using aggregate functions in C -
Step 1 - Create two string variables to store the two sentences.
Step 2 - Using the string flow library, split each sentence into independent words and store them in two separate arrays.
Step 3 - Make two sets, one for each sentence, to store unique words.
Step 4 - Loop through each word array and insert each word into the correct set.
Step 5 - Loop through each set and print out the unique words.
The Chinese translation ofExample 2
is:Example 2
In this code, we use the string stream library to split each sentence into separate words. We then use two collections, uniqueWords1 and uniqueWords2, to store the unique words in each sentence. Finally, we loop through each set and print out the non-duplicate words.
#include <iostream> #include <string> #include <sstream> #include <set> using namespace std; int main() { string sentence1 = "This is the first sentence."; string sentence2 = "This is the second sentence."; string word; stringstream ss1(sentence1); stringstream ss2(sentence2); set<string> uniqueWords1; set<string> uniqueWords2; while (ss1 >> word) { uniqueWords1.insert(word); } while (ss2 >> word) { uniqueWords2.insert(word); } cout << "Non-repeating words in sentence 1:" << endl; for (const auto& w : uniqueWords1) { if (uniqueWords2.find(w) == uniqueWords2.end()) { cout << w << " "; } } cout << endl; cout << "Non-repeating words in sentence 2:" << endl; for (const auto& w : uniqueWords2) { if (uniqueWords1.find(w) == uniqueWords1.end()) { cout << w << " "; } } cout << endl; return 0; }
输出
Non-repeating words in sentence 1: first Non-repeating words in sentence 2: second
结论
总之,从两个提供的句子中打印所有非重复单词的任务是通过使用各种编程方法来实现的,例如将句子分解为单个单词,利用字典来量化每个单词的频率,以及过滤掉非重复单词。生成的非重复单词集合可以报告给控制台或保存在列表或数组中以供进一步使用。这项工作对于基本的编程文本操作和数据结构操作很有帮助。The above is the detailed content of Print out all non-repeating words in two given sentences. For more information, please follow other related articles on the PHP Chinese website!
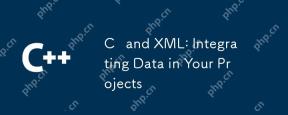
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
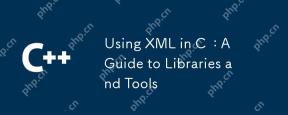
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
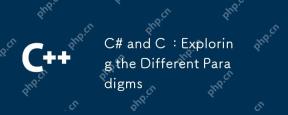
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
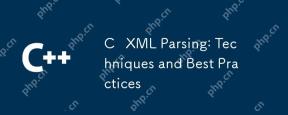
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
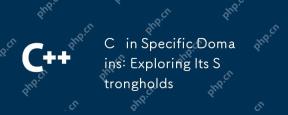
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
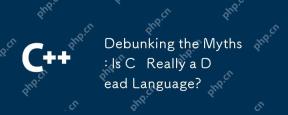
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
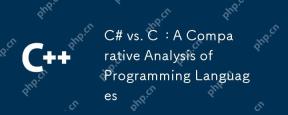
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
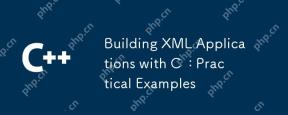
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
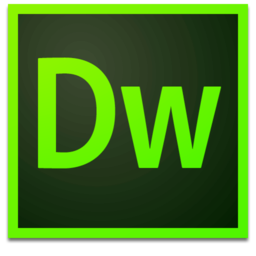
Dreamweaver Mac version
Visual web development tools
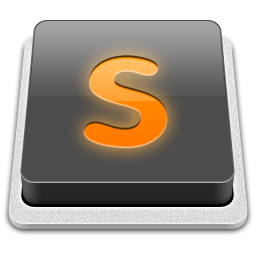
SublimeText3 Mac version
God-level code editing software (SublimeText3)
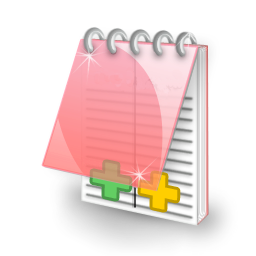
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
