In this problem, we need to find the last palindrome string in the array. If any string is the same when read, whether it is read from the beginning or from the end, it is said that the string is a palindrome. We can compare the start and end characters to check if a particular string is a palindrome. Another way to find a palindrome string is to reverse the string and compare it to the original string.
Problem Statement - We are given an array of length N containing different strings. We need to find the last palindrome string in the given array.
Example Example
Input– arr[] = {"werwr", "rwe", "nayan", "tut", "rte"};
Output – ‘tut’
Explanation– The last palindrome string in the given array is ‘tut’.
Input– arr[] = {"werwr", "rwe", "nayan", "acd", "sdr"};
Output-"nayan"
Explanation – ‘nayan’ is the last palindrome string in the given array.
Input– arr[] = {"werwr", "rwe", "jh", "er", "rte"};
Output-""
Explanation – Since the array does not contain any palindrome string, it prints the empty string.
method 1
In this method, we will iterate through the array from the beginning and store the last palindrome string in a variable. Additionally, we compare the starting and ending characters of the string to check if the string is a palindrome.
algorithm
Define variable 'lastPal' to store the last palindrome string.
Traverse the array.
Use the isPalindrome() function to check whether the string at the pth index in the array is a palindrome.
In the isPalindrome() function, use a loop to traverse the string.
Compares str[i] and str[len - p - 1] characters; if any characters do not match, return false.
Return true after all iterations of the loop are completed.
If the current string is a palindrome, update the value of the 'lastPal' variable with the current string.
Return "lastPal".
Example
#include <bits/stdc++.h> using namespace std; bool isPalindrome(string &str) { int size = str.length(); for (int p = 0; p < size / 2; p++) { // compare first ith and last ith character if (str[p] != str[size - p - 1]) { return false; } } return true; } string LastPalindrome(string arr[], int N) { string lastPal = ""; for (int p = 0; p < N; p++) { if (isPalindrome(arr[p])) { // if the current string is palindrome, then update the lastPal string lastPal = arr[p]; } } return lastPal; } int main() { string arr[] = {"werwr", "rwe", "nayan", "abba", "rte"}; int N = sizeof(arr)/sizeof(arr[0]); cout << "The last palindromic string in the given array is " << LastPalindrome(arr, N); return 0; }
Output
The last palindromic string in the given array is abba
Time complexity - O(N*K), because we loop through the array and check if each string is a palindrome.
Space complexity - O(1), because we are using constant space.
Method 2
In this method we will iterate through the array starting from the last one and when we find the last palindrome string we will return it. Additionally, we use the reverse() method to check if the string is a palindrome.
algorithm
Traverse the array starting from the last one.
Use the isPalindrome() function to check whether the string is a palindrome.
In the isPalindrome() function, store the 'str' string in the 'temp' variable.
Use the reverse() method to reverse a temporary string.
If str and temp are equal, return true. Otherwise, return false.
If the string at the i-th index is a palindrome, return the string.
Example
#include <bits/stdc++.h> using namespace std; bool isPalindrome(string &str) { string temp = str; reverse(temp.begin(), temp.end()); return str == temp; } string LastPalindrome(string array[], int N) { for (int p = N - 1; p >= 0; p--) { if (isPalindrome(array[p])) { return array[p]; } } // Return a default value if no palindrome is found return "No palindromic string found"; } int main() { string arr[] = {"werwr", "rwe", "nayan", "tut", "rte"}; int N = sizeof(arr) / sizeof(arr[0]); cout << "The last palindromic string in the given array is " << LastPalindrome(arr, N); return 0; }
Output
The last palindromic string in the given array is tut
Time complexity - O(N*K), since we iterate through the array and reverse the string.
Space complexity - O(1), because we don't use dynamic space.
Here, we learned two methods to find the last palindrome string in a given array. The time and space complexity of both methods are almost similar, but the second code is more readable and better than the first one.
Also, programmers can try to find the penultimate string in a given array and practice more.
The above is the detailed content of Find the last palindrome string in the given array. For more information, please follow other related articles on the PHP Chinese website!
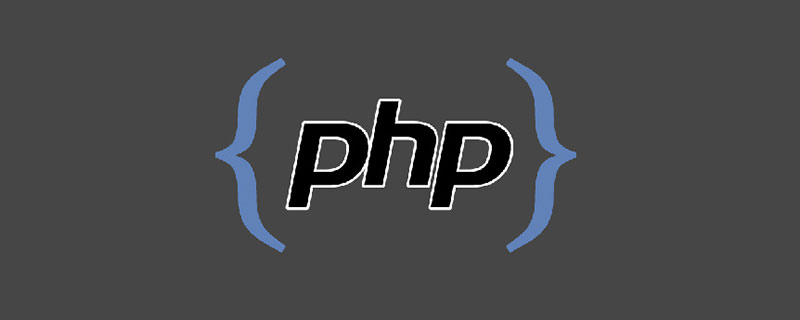
php求2个数组相同元素的方法:1、创建一个php示例文件;2、定义两个有相同元素的数组;3、使用“array_intersect($array1,$array2)”或“array_intersect_assoc()”方法获取两个数组相同元素即可。

C语言数组初始化的三种方式:1、在定义时直接赋值,语法“数据类型 arrayName[index] = {值};”;2、利用for循环初始化,语法“for (int i=0;i<3;i++) {arr[i] = i;}”;3、使用memset()函数初始化,语法“memset(arr, 0, sizeof(int) * 3)”。
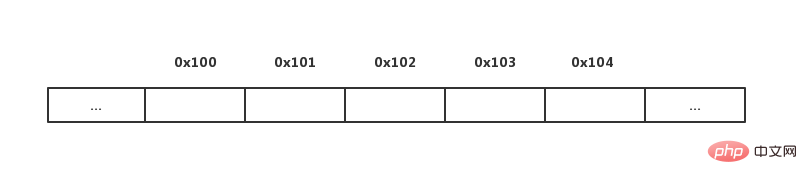
Part1聊聊Python序列类型的本质在本博客中,我们来聊聊探讨Python的各种“序列”类,内置的三大常用数据结构——列表类(list)、元组类(tuple)和字符串类(str)的本质。不知道你发现没有,这些类都有一个很明显的共性,都可以用来保存多个数据元素,最主要的功能是:每个类都支持下标(索引)访问该序列的元素,比如使用语法Seq[i]。其实上面每个类都是使用数组这种简单的数据结构表示。但是熟悉Python的读者可能知道这3种数据结构又有一些不同:比如元组和字符串是不能修改的,列表可以
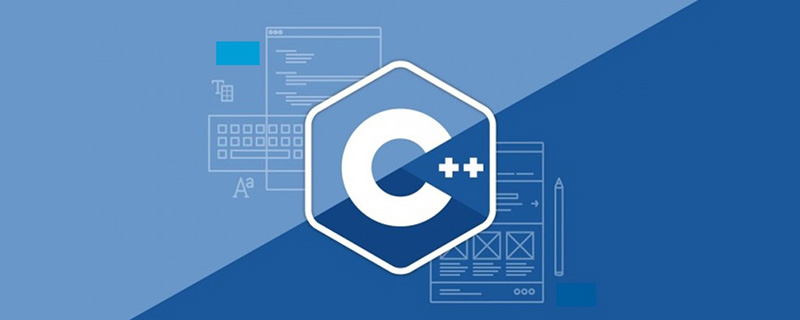
c++初始化数组的方法:1、先定义数组再给数组赋值,语法“数据类型 数组名[length];数组名[下标]=值;”;2、定义数组时初始化数组,语法“数据类型 数组名[length]=[值列表]”。
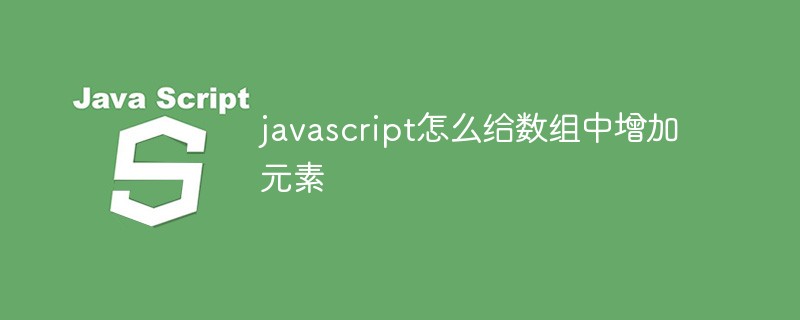
增加元素的方法:1、使用unshift()函数在数组开头插入元素;2、使用push()函数在数组末尾插入元素;3、使用concat()函数在数组末尾插入元素;4、使用splice()函数根据数组下标,在任意位置添加元素。
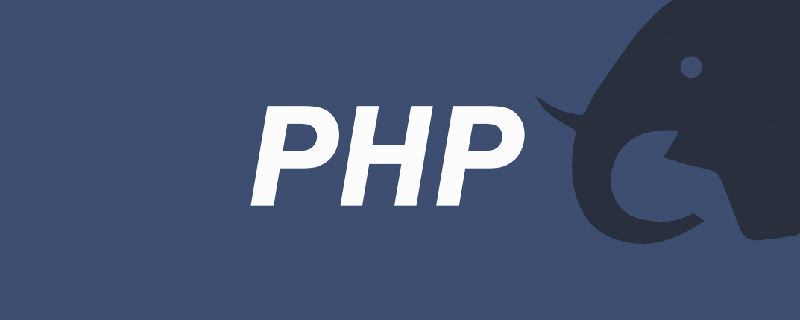
php判断数组里面是否存在某元素的方法:1、通过“in_array”函数在数组中搜索给定的值;2、使用“array_key_exists()”函数判断某个数组中是否存在指定的key;3、使用“array_search()”在数组中查找一个键值。
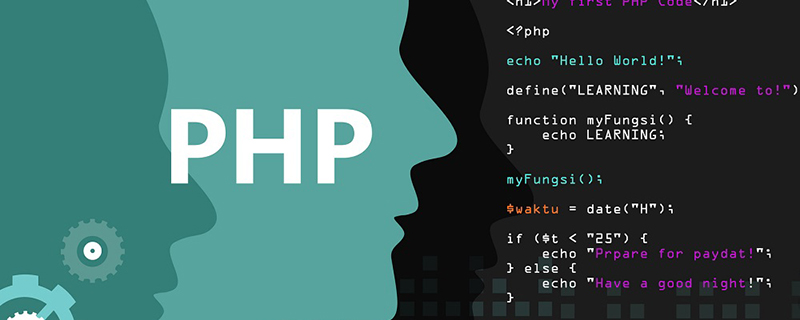
php去除第一个数组元素的方法:1、新建一个php文件,并创建一个数组;2、使用“array_shift”方法删除数组首个元素;3、通过“print_”r输出数组即可。
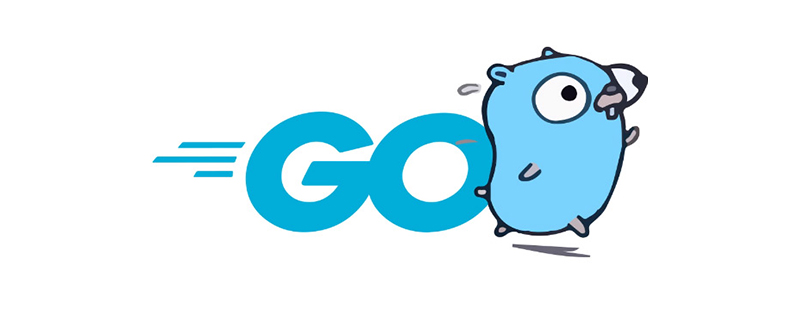
元组是固定长度不可变的顺序容器(元素序列),go语言中没有元组类型,数组就相当于元组。在go语言中,数组是一个由固定长度的特定类型元素组成的序列,一个数组可以由零个或多个元素组成;数组的声明语法为“var 数组变量名 [元素数量]Type”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
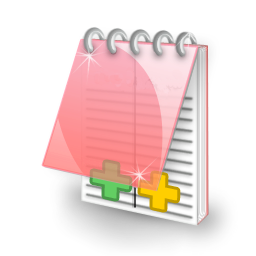
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use
