In this problem, we will find the K closest points to the origin in the 2D plane from the given N points.
We can use the standard Euclidean distance formula to calculate the distance between the origin and each given point. After that, we can store the points with distance into an array, sort the array based on distance, and take the top K points.
However, we can also use a priority queue to store 2D points based on their distance from the origin. Afterwards, we can dequeue K times from the queue.
Problem Statement− We are given N points on the two-dimensional plane. We need to find the K points closest to the origin of the plane.
Note - Treat Euclidean distance as the distance between the origin and a given point on the plane.
Example
enter
points = {{2, -2}, {-5, 1}, {2, 1}, {0, 3}, {6, 0}, {5, 5}, {4, 9}}, K = 4
Output
{2,1} {2,-2} {0,3} {-5,1}
Explanation − Here is the Euclidean distance from each point to the origin.
(2, −2) −> sqrt(8)
(−5, 1) −> sqrt(26)
(2, 1) -> sqrt(5)
(0, 3) −> sqrt(9)
(6, 0) -> sqrt(36)
(5, 5) -> sqrt(50)
(4, 9) -> sqrt(97)
Therefore, the 4 closest points are {2,1} {2,−2} {0,3} {−5,1}.
enter
points = {{1, 2}, {2, 1}, {-2, 1}, {1, -2}} K = 2
Output
{1, 2}, {2, 1}
Explanation − The distance from all points to the origin is the same. Therefore, we can print any 2 points as output.
enter
points = {{1, 3}, {6, 7}, {1, 1}, {1, 0}} K = 4
Output
{1, 0}, {1, 1}, {1, 3}, {6, 7}
Explanation− K is equal to the given point. Therefore, we need to print all points.
method one
In this method, we will sort the array using sort() method. Additionally, we will use a comparator function to sort the points based on their distance from the origin. After that, we print the first K elements of the sorted array.
algorithm
Step 1 − Use the sort() method to sort the list and pass the distComparator() function as the third parameter.
Step 2− Define the distComparator() function to compare the distance of a given point. This function takes as parameters a pair of p and q.
Step 2.1 − Get the distance from point p to the origin and store it in dist_p.
Step 2.2 − Store the distance from point q to the origin in the dist_q variable.
Step 2.3 − If dist_p is less than dist_q, return true. Otherwise, returns false.
Step 3- Iterate through the array and print the first K points of the array.
Example
#include <bits/stdc++.h> using namespace std; bool distComparator(const pair<int, int> &p, const pair<int, int> &q) { int dist_p = p.first * p.first + p.second * p.second; int dist_q = q.first * q.first + q.second * q.second; return dist_p < dist_q; } vector<pair<int, int>> findKPoints(vector<pair<int, int>> points, int n, int k) { vector<pair<int, int>> res_points; sort(points.begin(), points.end(), distComparator); // Get the first K points for (int p = 0; p < k; p++) { res_points.push_back({points[p].first, points[p].second}); } return res_points; } int main() { int k = 4, n = 7; vector<pair<int, int>> points{{2, -2}, {-5, 1}, {2, 1}, {0, 3}, {6, 0}, {5, 5}, {4, 9}}; vector<pair<int, int>> res = findKPoints(points, n, k); cout << "The " << k << " closest points from origin are - "; for (int p = 0; p < k; p++) { cout << "{" << res[p].first << "," << res[p].second << "} "; } return 0; }
Output
The 4 closest points from origin are - {2,1} {2,-2} {0,3} {-5,1}
Time complexity - The time complexity of sorting an array is O(NlogN).
Space Complexity - O(N) for sorting the array.
Method Two
In this method, we will use priority queue to insert points. Additionally, we will use a comparator function and a priority queue to store points based on their shortest distance from the origin.
algorithm
Step 1 − Define the ‘res_points’ list to store the K nearest points.
Step 2- Define priority queue. Here, ‘pair
Step 3− Define the cmp() function to compare the Euclidean distance between two points and the origin.
Step 3.1 - Return a Boolean value based on the distance from point a to the origin being greater than the distance from point b to the origin.
Step 4 - Insert each element of the array into the priority queue.
Step 5− Pop the first K elements from the queue and store them in the res_points list.
Step 6− Return the res_points list of points.
Example
#include <bits/stdc++.h> using namespace std; vector<pair<int, int>> findKPoints(vector<pair<int, int>> points, int n, int k) { vector<pair<int, int>> res_points; // Custom comparator to compare points based on their distance from the origin auto cmp = [](const pair<int, int>& a, const pair<int, int>& b) { return (a.first * a.first + a.second * a.second) > (b.first * b.first + b.second * b.second); }; // Use the custom comparator in the priority_queue priority_queue<pair<int, int>, vector<pair<int, int>>, decltype(cmp)> p_que(cmp); for (int p = 0; p < n; p++) { // Inserting points in a queue p_que.push(points[p]); } // Get first K points while (k--) { auto temp = p_que.top(); res_points.push_back(temp); p_que.pop(); } return res_points; } int main() { int k = 4, n = 7; vector<pair<int, int>> points{{2, -2}, {-5, 1}, {2, 1}, {0, 3}, {6, 0}, {5, 5}, {4, 9}}; vector<pair<int, int>> res = findKPoints(points, n, k); cout << "The " << k << " closest points from origin are - "; for (int p = 0; p < k; p++) { cout << "{" << res[p].first << "," << res[p].second << "} "; } return 0; }
Output
The 4 closest points from origin are - {2,1} {2,-2} {0,3} {-5,1}
Time complexity - O(N*logN) Insert N elements into the priority queue. Here, N is the total number of points.
Space Complexity - O(N) to store points in priority queue.
Priority queue uses heap data structure. Therefore, inserting and deleting elements only takes O(logN) time. Both methods require the same memory and time. However, the second method is more efficient because it uses the heap data structure.
The above is the detailed content of Use priority queue to find the K closest points to the origin. For more information, please follow other related articles on the PHP Chinese website!
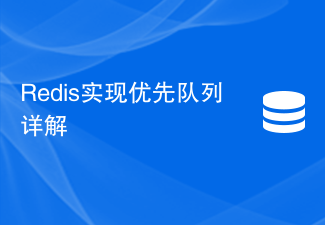
Redis实现优先队列详解优先队列是一种常见的数据结构,它可以按照某种规则对元素进行排序,并在队列操作时保持这个排序,从而使得队列中取出的元素总是按照预设的优先级进行。Redis作为一种内存数据库,因其快速、高效的数据访问能力,在实现优先队列时也有着优势。本文将详细介绍Redis实现优先队列的方法和应用。一、Redis实现基本原理Redis实现优先队列的基本
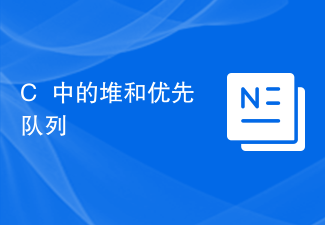
堆和优先队列是C++中常用的数据结构,它们都具有重要的应用价值。本文将分别对堆和优先队列进行介绍和解析,帮助读者更好地理解和使用它们。一、堆堆是一种特殊的树形数据结构,它可以用来实现优先队列。在堆中,每个节点都满足如下性质:它的值不小于(或不大于)其父节点的值。它的左右子树也是一个堆。我们将不小于其父节点的堆称为“最小堆”,将不大于其父节点的堆称为“最大堆”
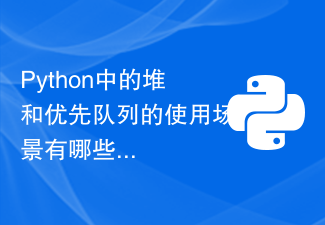
Python中的堆和优先队列的使用场景有哪些?堆是一种特殊的二叉树结构,常用于高效地维护一个动态的集合。Python中的heapq模块提供了堆的实现,可以方便地进行堆的操作。优先队列也是一种特殊的数据结构,不同于普通的队列,它的每个元素都有一个与之相关的优先级。最高优先级的元素先被取出。Python中的heapq模块也可以实现优先队列的功能。下面我们介绍一些
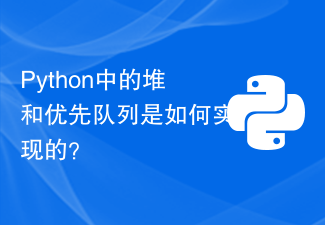
Python中的堆和优先队列是如何实现的?堆和优先队列是在计算机科学中常用的数据结构。在Python中,我们可以使用heapq模块来实现堆和优先队列。堆是一种特殊的完全二叉树,在堆中,每个父节点的值都比它的子节点的值要小(或大),这样的堆被称为小根堆(或大根堆)。在Python中,堆可以通过列表来表示。Python的heapq模块提供了一些方法来操作堆。首先
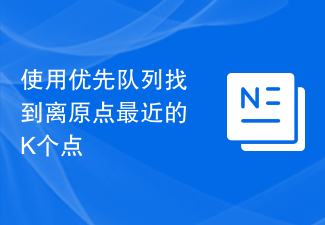
在这个问题中,我们将从给定的N个点中找到2D平面中距离原点最近的K个点。我们可以使用标准的欧氏距离公式来计算原点到每个给定点之间的距离。之后,我们可以将有距离的点存储到数组中,根据距离对数组进行排序,并取前K个点。然而,我们还可以使用优先队列根据点与原点的距离来存储二维点。之后,我们可以从队列中出队K次。问题陈述−我们在二维平面上给定了N个点。我们需要找到离平面原点最近的K个点。注意-将欧几里得距离视为原点和平面给定点之间的距离。示例输入points={{2,-2},{-5,1},{2,1},{
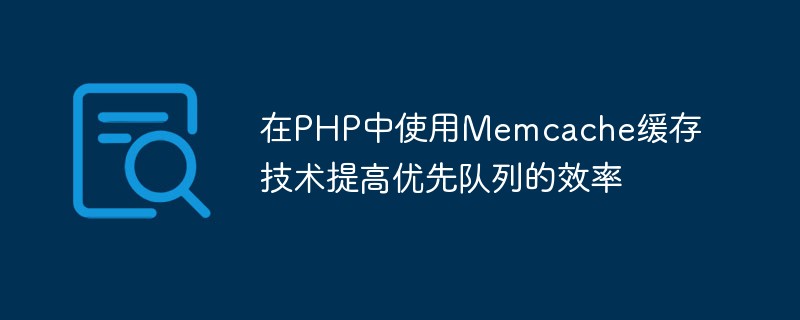
随着社会的不断发展,人们对于计算机技术的要求也变得越来越高。在计算机中,队列是一种非常重要的数据结构,能够帮助我们高效地解决很多问题。然而,在实际的应用过程中,队列的效率却往往会受到一些因素的限制,比如网络的延迟、查询数据库的速度等等。所以,今天我们来介绍一种解决这个问题的方法:在PHP中使用Memcache缓存技术,以提高优先队列的效率。一、什么是优先队列
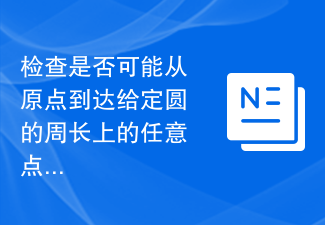
圆的周长可以定义为圆的外边界。它是圆的周长。圆周围的每个点都遵循某些属性,如下所示-点(x,y)位于圆内,使得$\mathrm{x^2+y^2R^2}$其中R=圆的半径。问题陈述给定一个表示一系列移动(L、R、U、D)的字符串S和一个表示圆半径的整数R。检查是否可以通过选择从S开始的任何移动子序列来到达以原点为半径为R的圆的圆周上的任何点。每个移动的操作如下所示,L=减少x坐标R=增量x坐标U=y坐标增量D=递减y坐标示例1输入S=“RURDLR”R=2输出Yes说明选择子序列“RR”-最初,(
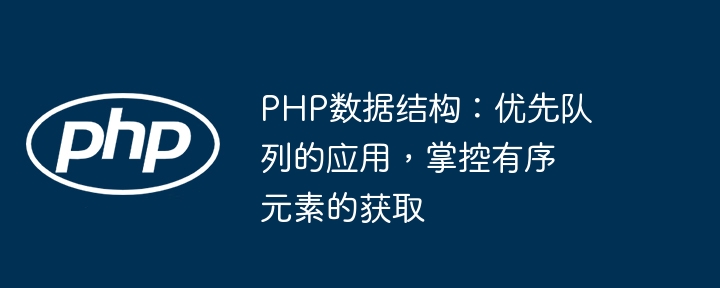
优先队列允许按优先级存储和访问元素,基于可比较标准(如值、时间戳或自定义逻辑)设定优先级。PHP中的实现方法包括SplPriorityQueue类和Min/Max堆。实战案例演示了如何使用SplPriorityQueue类创建优先队列并按优先级获取元素。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
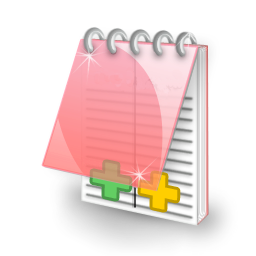
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
