


Newsletter application development based on Vue: Real-time data synchronization using Firebase Cloud Firestore
Vue-based newsletter application development: using Firebase Cloud Firestore to achieve real-time data synchronization, specific code examples are required
Introduction:
With the rapid development of the Internet , people's attention and demand for current affairs are also getting higher and higher. Today, many people want to be able to stay up to date with the latest news and hot topics anytime and anywhere. To meet this need, we can develop a Vue-based newsletter application and use Firebase Cloud Firestore to achieve real-time data synchronization. This article will introduce you to the development process based on Vue and Firebase, and provide detailed code examples.
1. Preparation:
- Install Node.js and Vue CLI.
- Create a new Vue project, name it "news-app", and enter the project directory.
2. Create a Firebase project:
- Enter the Firebase official website (https://firebase.google.com/) and log in with your Google account.
- Click "Get Started" to create a new project.
- In the project console, click "Add Application" and select "Web".
- Enter the name of the application, such as "NewsApp", and then click "Register Application".
- In the configuration code below, copy the contents of the "firebaseConfig" object.
3. Install Firebase dependencies:
- Open the terminal and enter the "news-app" project directory.
-
Run the following command to install Firebase related dependencies:
npm install firebase
- Create a new folder in the src directory and name it "firebase".
- Create a new file in the "firebase" folder and name it "config.js".
-
In the "config.js" file, paste the "firebaseConfig" object copied before and export it:
export default { // 粘贴firebaseConfig对象 }
4. Initialize Firebase:
-
Import Firebase and "firebase/config" files in the "main.js" file:
import firebase from 'firebase/app' import 'firebase/firestore' import firebaseConfig from './firebase/config'
-
Initialize Firebase:
firebase.initializeApp(firebaseConfig)
-
Create a Firebase Firestore instance:
const db = firebase.firestore()
-
Add the Firestore instance to the Vue prototype for access throughout the app:
Vue.prototype.$db = db
5. Create a news list page:
- Create a new file in the "src/views" directory and name it "NewsList.vue".
-
In the "NewsList.vue" file, write the following template code:
<template> <div> <h1 id="时事新闻">时事新闻</h1> <ul> <li v-for="news in newsList" :key="news.id"> {{ news.title }} </li> </ul> </div> </template>
-
In the "NewsList.vue" file, write the following script code:
<script> export default { data() { return { newsList: [] } }, mounted() { this.getNewsList() }, methods: { getNewsList() { this.$db.collection('news') .orderBy('timestamp', 'desc') .onSnapshot(snapshot => { this.newsList = snapshot.docs.map(doc => { const data = doc.data() return { id: doc.id, title: data.title } }) }) } } } </script>
6. Create a news addition page:
- Create a new file in the "src/views" directory and name it "AddNews.vue" .
-
In the "AddNews.vue" file, write the following template code:
<template> <div> <h1 id="添加新闻">添加新闻</h1> <form @submit.prevent="addNews"> <label for="title">标题:</label> <input type="text" id="title" v-model="title" required> <button type="submit">提交</button> </form> </div> </template>
-
In the "AddNews.vue" file, write the following script code:
<script> export default { data() { return { title: '' } }, methods: { addNews() { this.$db.collection('news').add({ title: this.title, timestamp: new Date() }) this.title = '' } } } </script>
7. Configure routing:
-
In the "src/router/index.js" file, import "NewsList.vue" and "AddNews.vue":
import NewsList from '@/views/NewsList.vue' import AddNews from '@/views/AddNews.vue'
-
In the "routes" array, create two routing objects:
{ path: '/', name: 'NewsList', component: NewsList }, { path: '/add', name: 'AddNews', component: AddNews }
8. Create the home page component :
- Create a new file in the "src/views" directory and name it "Home.vue".
-
In the "Home.vue" file, write the following template code:
<template> <div> <h1 id="时事通讯应用">时事通讯应用</h1> <router-link to="/">查看新闻</router-link> <router-link to="/add">添加新闻</router-link> <router-view></router-view> </div> </template>
9. Update App components:
-
In the "src/App.vue" file, replace the initial template code with the following code:
<template> <div id="app"> <router-view></router-view> </div> </template>
10. Run the application:
-
Run the following command in the terminal to start the application:
npm run serve
- Open the browser and visit "http://localhost:8080" to see the home page of the application.
Conclusion:
Through the sample code in this article, we successfully created a Vue-based newsletter application and used Firebase Cloud Firestore to achieve real-time data synchronization. Developers can continue to improve the application according to their own needs and ideas, such as adding user authentication, comment functions, etc. I hope this article can be helpful to the practical application of Vue and Firebase, so that your application can better meet the needs of users.
The above is the detailed content of Newsletter application development based on Vue: Real-time data synchronization using Firebase Cloud Firestore. For more information, please follow other related articles on the PHP Chinese website!

Netflixusesacustomframeworkcalled"Gibbon"builtonReact,notReactorVuedirectly.1)TeamExperience:Choosebasedonfamiliarity.2)ProjectComplexity:Vueforsimplerprojects,Reactforcomplexones.3)CustomizationNeeds:Reactoffersmoreflexibility.4)Ecosystema

Netflix mainly considers performance, scalability, development efficiency, ecosystem, technical debt and maintenance costs in framework selection. 1. Performance and scalability: Java and SpringBoot are selected to efficiently process massive data and high concurrent requests. 2. Development efficiency and ecosystem: Use React to improve front-end development efficiency and utilize its rich ecosystem. 3. Technical debt and maintenance costs: Choose Node.js to build microservices to reduce maintenance costs and technical debt.

Netflix mainly uses React as the front-end framework, supplemented by Vue for specific functions. 1) React's componentization and virtual DOM improve the performance and development efficiency of Netflix applications. 2) Vue is used in Netflix's internal tools and small projects, and its flexibility and ease of use are key.

Vue.js is a progressive JavaScript framework suitable for building complex user interfaces. 1) Its core concepts include responsive data, componentization and virtual DOM. 2) In practical applications, it can be demonstrated by building Todo applications and integrating VueRouter. 3) When debugging, it is recommended to use VueDevtools and console.log. 4) Performance optimization can be achieved through v-if/v-show, list rendering optimization, asynchronous loading of components, etc.

Vue.js is suitable for small to medium-sized projects, while React is more suitable for large and complex applications. 1. Vue.js' responsive system automatically updates the DOM through dependency tracking, making it easy to manage data changes. 2.React adopts a one-way data flow, and data flows from the parent component to the child component, providing a clear data flow and an easy-to-debug structure.

Vue.js is suitable for small and medium-sized projects and fast iterations, while React is suitable for large and complex applications. 1) Vue.js is easy to use and is suitable for situations where the team is insufficient or the project scale is small. 2) React has a richer ecosystem and is suitable for projects with high performance and complex functional needs.
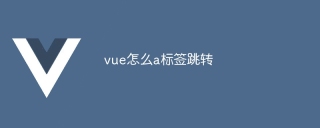
The methods to implement the jump of a tag in Vue include: using the a tag in the HTML template to specify the href attribute. Use the router-link component of Vue routing. Use this.$router.push() method in JavaScript. Parameters can be passed through the query parameter and routes are configured in the router options for dynamic jumps.
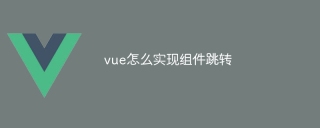
There are the following methods to implement component jump in Vue: use router-link and <router-view> components to perform hyperlink jump, and specify the :to attribute as the target path. Use the <router-view> component directly to display the currently routed rendered components. Use the router.push() and router.replace() methods for programmatic navigation. The former saves history and the latter replaces the current route without leaving records.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
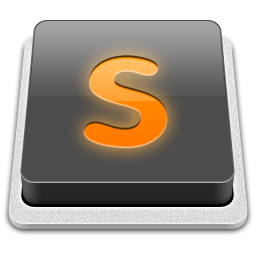
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
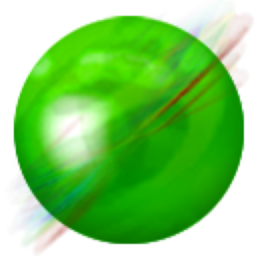
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment