


In this problem, we need to count the total number of strings that contain the same number of lowercase and uppercase characters in a given string. The naive way to solve this problem is to find all substrings and count the total number of substrings with the same number of lowercase and uppercase characters.
An effective method is to use subarray summation problem. We can treat lower case characters as -1 and upper case characters as 1 and we will learn both ways to solve the problem.
Problem Statement- We are given a string str which contains lowercase and uppercase alphabetic characters. We need to count the total number of substrings that contain the same number of lowercase and uppercase characters.
Example
Input – str = ‘TutOR’
Output – 4
Explanation – We can get 4 substrings, which are 'Tu', 'TutO', 'tO' and 'utOR', which contain the same number of lowercase letters and uppercase letters
Input – str = ‘abcd’
Output – 0
Explanation – We cannot get any substring containing the same lowercase and uppercase characters because the string contains only lowercase characters
Input – str = ‘XYz’
Output– 1
Explanation - We can only get the 'Yz' substring.
method 1
This is a naive way to solve the problem. We will use 3 nested loops to find all substrings of a given string. We will check if each substring contains the same number of lowercase and uppercase characters. If so, we increment the count by 1.
algorithm
Define variable 'cnt' and initialize it to zero.
Use a for loop to traverse the string
In the loop, define the 'upperCase' and 'lowerCase' variables and initialize them with zeros
Add another nested loop to the code to iterate the string starting from the i-th index. In this way, we can get a substring
from the i-th index to the j-th index
In nested loops, if the character is uppercase, increase the value of the uppercase variable by 1. Otherwise, increase the value of the lowercase variable by 1.
If the values of the 'upperCase' and 'lowerCase' variables are equal, increase the value of 'cnt' by 1.
Return the value of 'cnt'.
Example
is:Example
#include <iostream> using namespace std; // function to find the total number of substrings that have an equal number of lowercase and uppercase characters int totalSubStrings(string &str, int N){ // variable to store the count. int cnt = 0; for (int i = 0; i < str.length(); i++){ // variable to store the count of upper and lower case characters int upperCase = 0; int lowerCase = 0; for (int j = i; j < str.length(); j++){ // If the character is in uppercase, then increment upperCase if (str[j] >= 'A' && str[j] <= 'Z') upperCase++; else lowerCase++; // If the count of uppercase and lowercase characters is equal, then increment cnt if (upperCase == lowerCase) cnt++; } } return cnt; } int main(){ string str = "TutOR"; cout << "The total number of substrings that have equal lowercase and uppercase characters is " << totalSubStrings(str, str.length()); return 0; }
Output
The total number of substrings that have equal lowercase and uppercase characters is 4
Time complexity - O(N^2), because we use nested loops to find all substrings.
Space complexity - O(1), because we use constant space.
Method Two
In this method, we will optimize the code of the above method to improve the time complexity of the solution. We treat uppercase characters as 1 and lowercase characters as -1. Additionally, we will use a map data structure to store the frequency of the prefix sum. If we find that the prefix sum is equal to zero, or the same as any prefix sum stored in the map, we can increment the count value.
algorithm
Define a "sum" map containing integers as key-value pairs.
The variable 'cnt' is defined and initialized to zero to store the total number of substrings with equal lowercase and uppercase characters. At the same time, define the variable 'current' to store the prefix and
Start traversing the string. If the current character is an uppercase letter, increase the 'current' variable by 1. Otherwise, decrement the 'current' character by 1.
If the value of 'current' is 0, we find the substring and increment the value of 'cnt' by 1
Check if the map contains "current" as a key. If it is, then get its value and add it to the "cnt" variable.
Increase the value of the "current" key in the map by 1.
Example
is:Example
To better understand the problem. Let's debug the example input, which is 'TutOR'.
So, when we start iterating the string, the value of 'current' will become 0 at the first index. So, increase the value of 'cnt' by 1. Again, at the third index it will go to zero. So, increase the value of 'cnt' by 1 to 2. We also got 0 before, so it's present in the map and we need to add that value to 'cnt'. So, it will become 3.
When we reach the 4th index, the value of the 'current' variable will be 1 and we get it again just like we got it at the 0th index. So add '1' to 'cnt'.
The basic logic is that if 'current' is 0, we get the substring. If we get the value of 'current' again, we can get the string between the two indices as 'current - current' is zero.
<span style="font-size: 13.125px;">#include <iostream> #include <unordered_map> using namespace std; // function to find the total number of substrings that have an equal number of lowercase and uppercase characters int totalSubStrings(string &str, int N){ // map to store the frequency of sums generated by the difference in the count of lowercase and uppercase characters unordered_map<int, int> sum; // to store the count of substrings that have an equal number of lowercase and uppercase characters int cnt = 0; // current sum int current = 0; for (int i = 0; i < N; i++){ // If the character is uppercase, then increment the current value if (str[i] >= 'A' and str[i] <= 'Z'){ current++; } // Otherwise, decrement the current value else current--; // If the current value is 0, then a substring is found. So, increment the count by 1 if (current == 0) cnt++; // If the current value exists in the map, then update the cnt by adding the frequency of the current value if (sum[current]){ cnt += (sum[current]); } // Increment the frequency of the current value in the map sum[current]++; } return cnt; } int main(){ string str = "TutOR"; cout << "The total number of substrings that have equal lowercase and uppercase characters is " << totalSubStrings(str, str.length()); return 0; }</span>
Output
The total number of substrings that have equal lowercase and uppercase characters is 4
Time complexity - O(N), because we only traverse the string once.
Space Complexity – O(N) because we use a map to store the prefix sum. In the worst case, when the string contains all lowercase or uppercase characters, we need O(N) space.
We learned to use two different methods to solve the above problem. The first method uses nested loops to check all strings, while the second method is more efficient than the first in terms of time complexity, but takes up more space.
The above is the detailed content of Number of substrings with the same number of lowercase and uppercase letters. For more information, please follow other related articles on the PHP Chinese website!
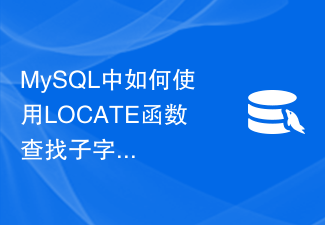
MySQL中如何使用LOCATE函数查找子字符串在字符串中的位置在MySQL中,有许多函数可以用来处理字符串。其中,LOCATE函数是一种非常有用的函数,可以用来查找子字符串在字符串中的位置。LOCATE函数的语法如下:LOCATE(substring,string,[position])其中,substring为要查找的子字符串,string为要在其中
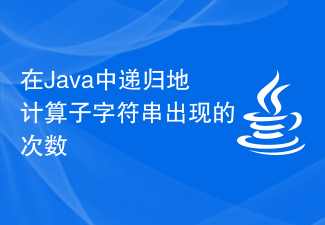
给定两个字符串str_1和str_2。目标是使用递归过程计算字符串str1中子字符串str2的出现次数。递归函数是在其定义中调用自身的函数。如果str1是"Iknowthatyouknowthatiknow",str2是"know"出现次数为-3让我们通过示例来理解。例如输入str1="TPisTPareTPamTP",str2="TP";输出Countofoccurrencesofasubstringrecursi
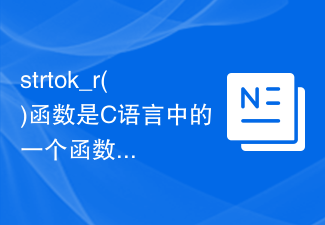
该函数与strtok()函数类似。唯一的关键区别是_r,它被称为可重入函数。可重入函数是在执行过程中可以被中断的函数。这种类型的函数可用于恢复执行。因此,可重入函数是线程安全的,这意味着它们可以安全地被线程中断,而不会造成任何损害。strtok_r()函数有一个称为上下文的额外参数。这样函数就可以在正确的位置恢复。strtok_r()函数的语法如下:#include<string.h>char*strtok_r(char*string,constchar*limiter,char**
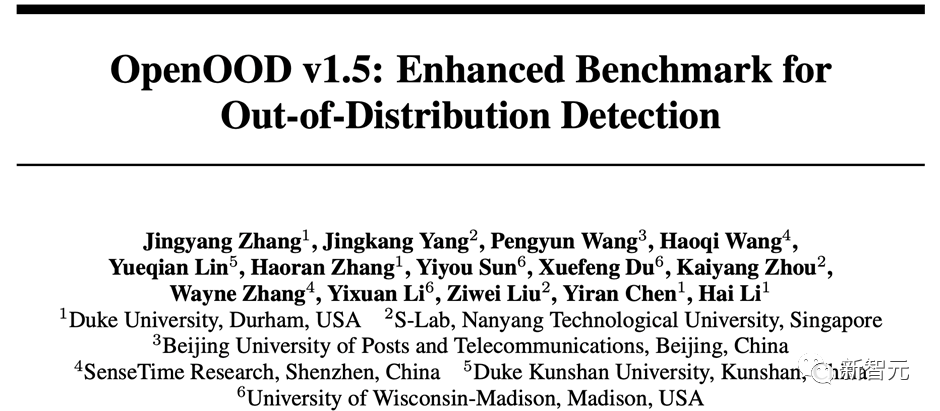
分布外(OOD)检测对于开放世界智能系统的可靠运行至关重要,但目前面向对象的检测方法存在「评估不一致」(evaluationinconsistencies)的问题。之前的工作OpenOODv1统一了OOD检测的评估,但在可扩展性和可用性方面仍然存在限制。最近开发团队再次提出OpenOODv1.5,相比上一版本,新的OOD检测方法评估在确保准确、标准化和用户友好等方面得到显著提升。图片Paper:https://arxiv.org/abs/2306.09301OpenOODCodebase:htt
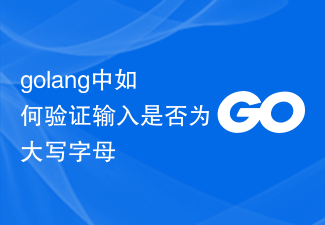
Golang是一门高性能、现代化的编程语言,在日常开发中经常涉及到字符串的处理。其中,验证输入是否为大写字母是一个常见的需求。本文将介绍在Golang中如何验证输入是否为大写字母。方法一:使用unicode包Golang中的unicode包提供了一系列函数来判断字符的编码类型。对于大写字母,其对应的编码范围为65-90(十进制),因此我们可以使用unicod
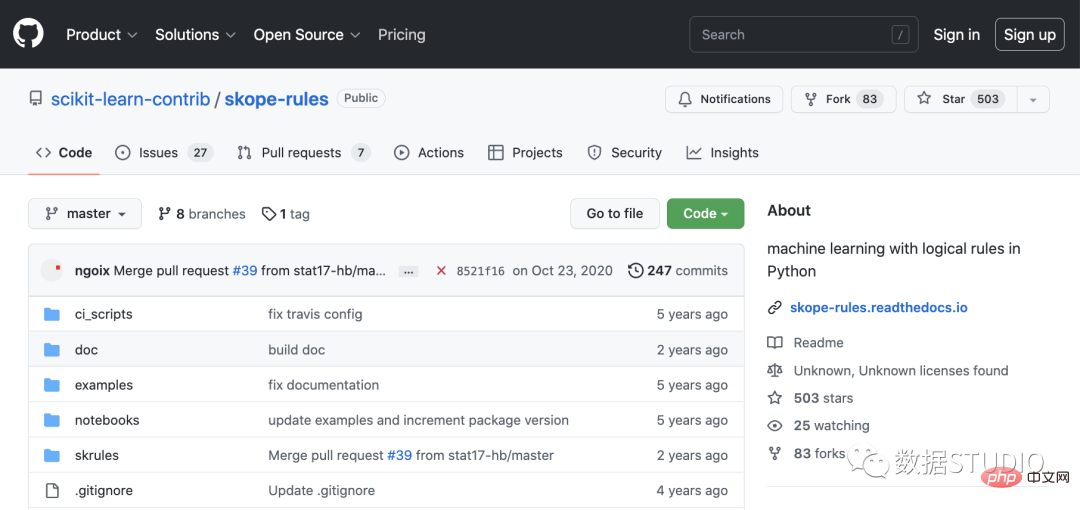
在准确率-召回率曲线上,同样的点是用不同的坐标轴绘制的。警告:左边的第一个红点(0%召回率,100%精度)对应于0条规则。左边的第二个点是第一个规则,等等。 Skope-rules使用树模型生成规则候选项。首先建立一些决策树,并将从根节点到内部节点或叶子节点的路径视为规则候选项。然后通过一些预定义的标准(如精确度和召回率)对这些候选规则进行过滤。只有那些精确度和召回率高于其阈值的才会被保留。最后,应用相似性过滤来选择具有足够多样性的规则。一般情况下,应用Skope-rules来学习每个根本原因的
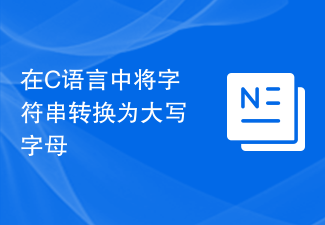
这是一个将字符串转换为大写字母的C语言程序示例:示例#include<stdio.h>#include<string.h>intmain(){ chars[100]; inti; printf("Enterastring:"); gets(s); for(i=0;s
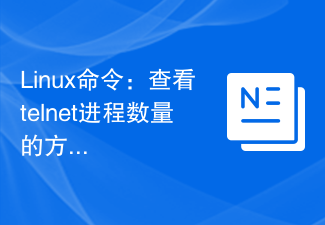
Linux命令是系统管理员日常工作中必不可少的工具之一,它们可以帮助我们完成各种系统管理任务。在运维工作中,有时候需要查看系统中某个进程的数量以便及时发现问题和进行调优。本文将介绍如何使用Linux命令查看telnet进程的数量,让我们一起来学习吧。在Linux系统中,我们可以使用ps命令结合grep命令来查看telnet进程的数量。首先,我们需要打开终端,


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor
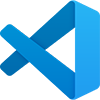
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
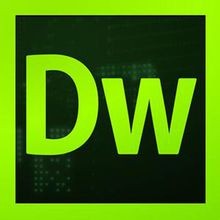
Dreamweaver CS6
Visual web development tools
