Nowadays, many applications allow users to upload and download their files. For example, plagiarism detection tools allow users to upload a document file that contains some text. It then checks for plagiarism and generates a report that users can download.
Everyone knows to use input type file to create a file upload button, but few developers know how to use JavaScript/JQuery to create a file download button.
This tutorial will teach various ways to trigger file downloads when an HTML button or JavaScript is clicked.
Use HTML's tag and download attribute to trigger file download when the button is clicked
Whenever we add the download attribute to the tag, we can use the tag as a file download button. We need to pass the URL of the file as the value of the href attribute to allow the user to download a specific file when they click on the link.
grammar
Users can create a file download button using the tag according to the following syntax.
<a href = "file_path" download = "file_name">
In the above syntax, we added the download attribute and the file name as the value of the download attribute.
parameter
file_path – This is the path to the file we want the user to download.
Example 1
is translated as:Example 1
In the example below, we are passing the image URL as the value of the href attribute of the HTML tag. We use the download button as the anchor text for the tagWhenever the user clicks the button, they can see it triggers the file download.
<html> <body> <h3 id="Using-the-i-download-attribute-of-a-tag-i-to-create-file-download-button-using-JavaScript"> Using the <i> download attribute of <a> tag </i> to create file download button using JavaScript. </h3> <p> Click the below button to download the image file </p> <a href = "https://images.pexels.com/photos/268533/pexels-photo-268533.jpeg?cs=srgb&dl=pexels-pixabay-268533.jpg&fm=jpg" Download = "test_image"> <button type = "button"> Download </button> </a> </body> </html>
Use window.open() method
The window.open() method opens a URL in a new tab. We can pass the URL as a parameter of the open() method.
If the open() method cannot open the URL, file download will be triggered.
grammar
Users can use the window.open() method according to the following syntax to create a file download button.
window.open("file_url")
In the above syntax, we pass the file URL as a parameter of the window.open() method.
Example 2
In the example below, whenever the user clicks the button, it triggers the downloadFile() function. In the downloadFile() function, the window.open() method triggers file downloading.
<html> <body> <h3 id="Using-the-i-window-open-method-i-to-create-a-file-download-button-using-JavaScript"> Using the <i> window.open() method </i> to create a file download button using JavaScript. </h3> <p> Click the below button to download the image file </p> <button type = "button" onclick = "downloadFile()"> Download </button> </body> <script> function downloadFile() { window.open("https://images.pexels.com/photos/268533/pexels-photo-268533.jpeg?cs=srgb&dl=pexels-pixabay-268533.jpg&fm=jpg") } </script> </html>
Get user input, create a file using that input, and allow the user to download the file
This method will allow the user to write text in the input box. After that, using the entered text, we will create a new file and allow the user to download the file.
grammar
Users can create a file with custom text following the following syntax and allow users to download it.
var hidden_a = document.createElement('a'); hidden_a.setAttribute('href', 'data:text/plain;charset=utf-8,' + encodeURIComponent(texts)); hidden_a.setAttribute('download', "text_file"); document.body.appendChild(hidden_a); hidden_a.click();
In the above syntax, we encoded the text to append it to the file and created it using the tag.
algorithm
Step One - Get the text by accessing the HTML input.
Step 2 − Create a custom HTML tag using JavaScript createElement() method.
Step 3 − Use the setAttribute() method to set the href attribute for the hidden_a HTML element. Use the encoded text as the value of the href attribute.
Step 4 − Use the setAttribute() method again and set the download attribute to the download file name value of the hidden element hidden_a.
Step 5 - Append the hidden_a element to the body.
Step 6 - Use the click() method to trigger a click on the hidden_a element. When it calls the click() method, it starts downloading.
Step 7 - Use the removeChild() method to remove the hidden_a element from the document body.
Example 3
is:Example 3
In the example below, users can enter any custom text in the input field and click the button to trigger file download using JavaScript. We have implemented the above algorithm to trigger a file download.
<html> <body> <h3 id="Create-the-file-from-the-custom-text-and-allow-users-to-download-that-file"> Create the file from the custom text and allow users to download that file </h3> <p> Click the below button to download the file with custom text. </p> <input type = "text" id = "file_text" value = "Entetr some text here."> <button type = "button" onclick = "startDownload()"> Download </button> </body> <script> function startDownload() { // access the text from the input field let user_input = document.getElementById('file_text'); let texts = user_input.value; // Create dummy <a> element using JavaScript. var hidden_a = document.createElement('a'); // add texts as a href of <a> element after encoding. hidden_a.setAttribute('href', 'data:text/plain;charset=utf-8, '+ encodeURIComponent(texts)); // also set the value of the download attribute hidden_a.setAttribute('download', "text_file"); document.body.appendChild(hidden_a); // click the link element hidden_a.click(); document.body.removeChild(hidden_a); } </script> </html>
Use axios library to create a download file button
axios library allows us to get data from any URL. So we will get the data from any URL or file path and then set that data as the value of the href attribute of the tag. Additionally, we will add the download attribute to the tag using the setAttribute() method and trigger the file download using the click() method.
grammar
Users can use axios and JavaScript to trigger file downloads according to the following syntax.
let results = await axios({ url: 'file_path', method: 'GET', responseType: 'blob' }) // use results as a value of href attribute of <a> tag to download file hidden_a.href = window.URL.createObjectURL(new Blob([results.data]));
In the above syntax, the axios.get() method allows us to get data from the file_path stored in the results variable. After that, we use the new Blob() constructor to convert the data into a Blob object.
The Chinese translation ofExample 4
is:Example 4
In the example below, we use axios to get the data from the URL, convert it to a Blob object, and set it as the value of the href attribute.
Afterwards, we clicked on the element via JavaScript to trigger the file download.
<html> <head> <script src = "https://cdnjs.cloudflare.com/ajax/libs/axios/1.3.1/axios.min.js"> </script> </head> <body> <h3 id="Using-the-i-axios-library-i-to-trigger-a-download-file"> Using the <i> axios library </i> to trigger a download file. </h3> <p> Click the below button to download the file with custom text. </p> <button type = "button" onclick = "startDownload()"> Download </button> </body> <script> async function startDownload() { // get data using axios let results = await axios({ url: 'https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcTZ4gbghQxKQ00p3xIvyMBXBgGmChzLSh1VQId1oyhYrgir1bkn812dc1LwOgnajgWd-Yo&usqp=CAU', method: 'GET', responseType: 'blob' }) let hidden_a = document.createElement('a'); hidden_a.href = window.URL.createObjectURL(new Blob([results.data])); hidden_a.setAttribute('download', 'download_image.jpg'); document.body.appendChild(hidden_a); hidden_a.click(); } </script> </html>
The above is the detailed content of How to trigger file download when clicking HTML button or JavaScript?. For more information, please follow other related articles on the PHP Chinese website!
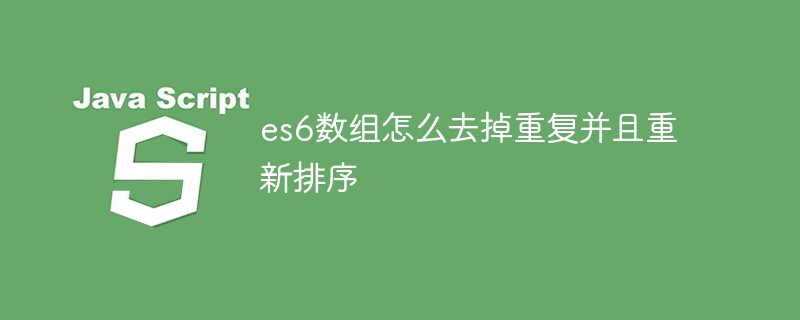
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
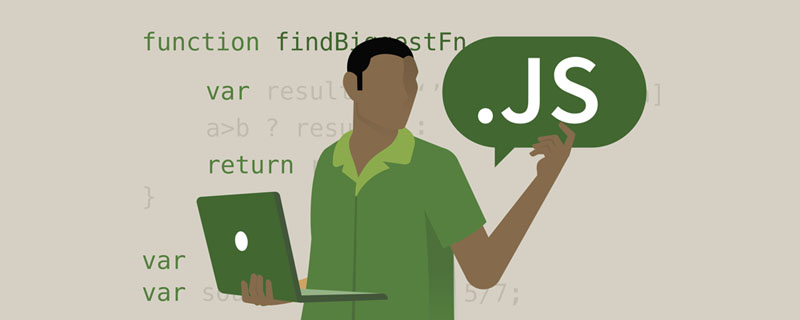
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
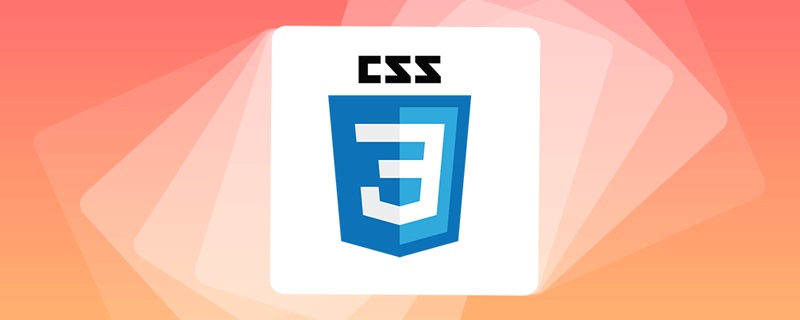
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
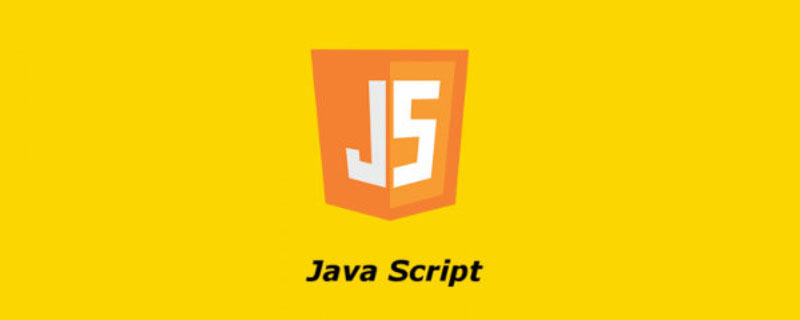
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
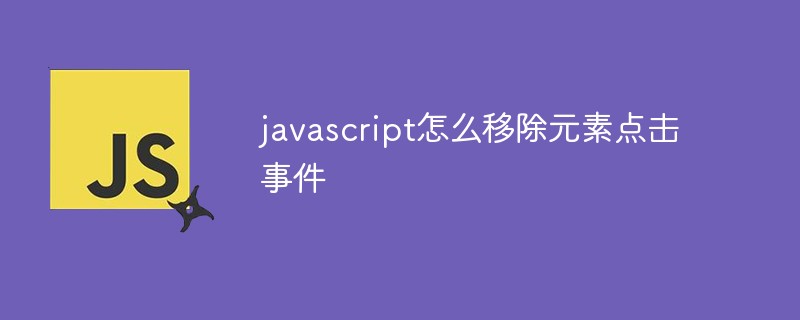
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
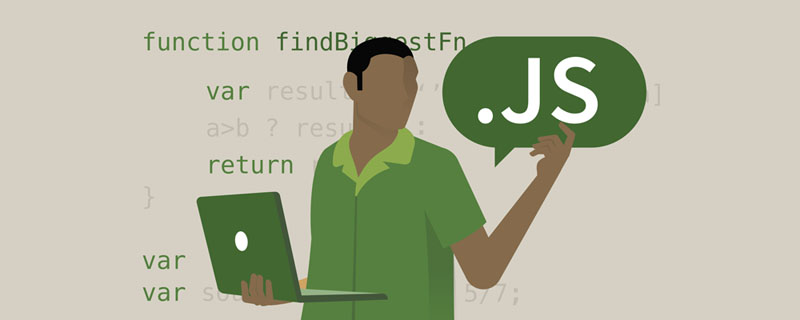
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
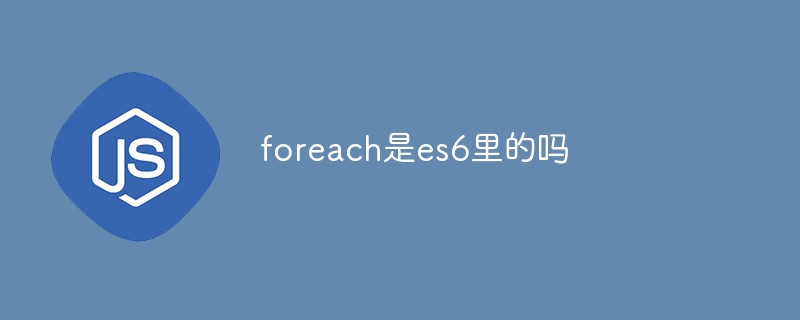
foreach不是es6的方法。foreach是es3中一个遍历数组的方法,可以调用数组的每个元素,并将元素传给回调函数进行处理,语法“array.forEach(function(当前元素,索引,数组){...})”;该方法不处理空数组。
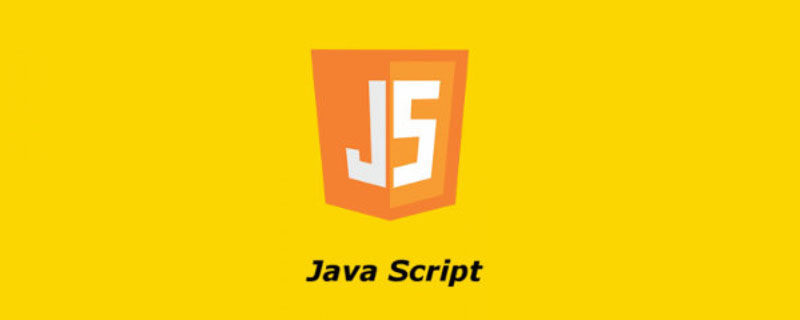
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
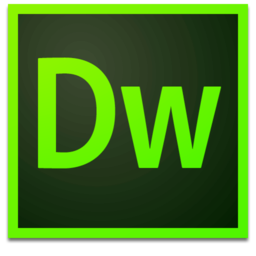
Dreamweaver Mac version
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
