Java program that handles exception methods
Exception is an abnormal event that disrupts the normal execution flow of the program. When an exception occurs, an object called the exception object is generated, which contains the details of the exception such as name, description, and program status. In this section we will write a java program to handle different exception methods present in java.
Exception type
There are three types of exceptions −
Checked exception − Checked exceptions are compile-time exceptions, which are checked during program compilation. These exceptions cannot be ignored and must be handled by the programmer. For example: IOException, SQLException, ClassNotFounException.
Unchecked exceptions - Unchecked exceptions are runtime exceptions, i.e. they are ignored during compilation and checked during program execution. For example: NullPointerException (null pointer exception), ArithmeticException (arithmetic exception) and ArrayIndexOutOfBoundsException (array out-of-bounds exception).
Error − Errors are unrecoverable exceptions that usually occur due to problems with the Java virtual machine or system resources. Errors, unlike exceptions, should not be caught or handled by programmers because they tell us that there is a serious problem that cannot be fixed by the program. For example: OutOfMemoryError, StackOverflowError.
Exception handling
Exception handling is the process of handling exceptions raised during program execution so that the execution flow is not interrupted. This is done using try-catch blocks in Java. The try block contains code that may throw exceptions, and the catch block contains code that handles exceptions.
We can use built-in exceptions or create custom or user-defined exceptions. Custom exceptions extend the Exception class or the RuntimeException class.
Java provides many methods to handle these exceptions. Some of these methods are -
getMessage() − This method is used to return the error message as a string. This is a method defined in the Throwable class in Java.
try { // some code that may throw an exception } catch (Exception e) { String message = e.getMessage(); System.out.println("Exception occurred: " + message);
getStackTrace() - This method returns an array of the sequence of method calls that caused the exception. This is the method defined in Throwable class in Java.
try { // some code that may throw an exception } catch (Exception e) { StackTraceElement[] st = e.getStackTrace(); for (StackTraceElement ele : st) { System.out.println(ele.toString()); } }
printStackTrace() - This method prints an array of the sequence of method calls that caused the exception. This is the method defined in Throwable class in Java.
try { // some code that may throw an exception } catch (Exception e) { e.printStackTrace(); }
Throw − 'throw' is the keyword in Java used to explicitly throw exceptions. When this keyword is executed, the normal program flow will be stopped and it will throw an exception, which will be caught by the nearest catch statement.
public void divide(int a, int b) { if (b == 0) { throw new ArithmeticException("Cannot divide by zero!"); // throws Arthemetic Exception } int result = a / b; System.out.println("Result: " + result); }
getCause() − This method returns the cause of other exceptions that raised this exception. If the cause is unknown, 'null' is returned. This is a method defined in the Throwable class in Java.
try { // some code that may throw an exception } catch (Exception e) { Throwable cause = e.getCause(); if (cause != null) { System.out.println("Cause: " + cause.toString()); } else { System.out.println("No cause found."); } }
grammar
try-catch block - try-catch block in java is used to handle exceptions. The try block contains code that may throw an exception. The catch block catches exceptions and handles them. An attempt can be followed by a set of catch blocks.
try { // Protected code } catch (ExceptionName e1) { // Catch block }
Now, we will discuss in detail the different ways of handling method exceptions in Java.
Method 1: Use a single try-catch block
In this approach, we will use a single try and a single catch block to handle the exception that occurs.
algorithm
Initialize an array with random values.
Try to access an element in the array such that the index should be greater than the length of the array. Put this code in a try block as it will throw an exception.
Once an exception occurs, use the catch() method to capture the ArrayIndexOutOfBounds exception, use the getMessage() method to print the message, and use the printStackTrace() method to print the sequence of method calls.
Example
In this example, we write the code in try and catch blocks. In the try block, we initialize an array with 5 values and access the 8th element of the array, which usually throws an exception "ArrayIndexOutOfBoundsException" . The catch block captures this exception and uses the getMessage() method to print the error message. The printStackTrace() method is used to print the method call sequence when the exception occurs.
import java.util.*; public class Main { public static void main(String[] args) { try { int[] arr = {1, 2, 3,4,5}; System.out.println(arr[8]); } catch (ArrayIndexOutOfBoundsException e) { System.out.println("An exception occurred: " + e.getMessage()); e. printStackTrace() ; } } }
Output
An exception occurred: Index 8 out of bounds for length 5 java.lang.ArrayIndexOutOfBoundsException: Index 8 out of bounds for length 5 at Main.main(Main.java:6)
Method 2: Use a single try block and multiple catch blocks
In this approach, we will use a single try and multiple catch blocks to handle the exceptions that occur.
algorithm
Declare a try block and initialize two integer variables, namely numerator and denominator. The denominator variable is initialized to 0.
Now, if the denominator value is equal to 0, an ArithmeticException is thrown.
Write multiple catch blocks to handle different exceptions.
Use different built-in methods in java and print the exception message and the exception that occurred.
示例
在此示例中,我们使用了一个 try 块,后跟多个 catch 块。如果从 try 块中抛出 ArithmeticException,则执行处理 ArithmeticException 代码的 catch 块。如果 try 块抛出 NullPointerException,则执行该特定的 catch 块。如果 catch 块不处理 try 块引发的特定异常,则执行最后一个 catch 块代码,因为它正在处理通用异常情况。从示例中,当分母值初始化为零时,我们使用“throw”关键字抛出一个 ArthemeticException,并执行处理 ArthemeticException 的 catch 块。
import java.util.*; public class Main { public static void main(String[] args) { try { int numerator = 10, denominator = 0 ; if(denominator == 0) { throw new ArithmeticException(); } } catch (ArithmeticException e) { System.out.println("ArithmeticException caught."); System.out.println("Message: " + e.getMessage()); System.out.println("Stack Trace: "); e.printStackTrace(); System.out.println("Cause: " + e.getCause()); } catch (NullPointerException e) { System.out.println("NullPointerException caught."); System.out.println("Message: " + e.getMessage()); System.out.println("Stack Trace: "); e.printStackTrace(); System.out.println("Cause: " + e.getCause()); } catch (ArrayIndexOutOfBoundsException e) { System.out.println("ArrayIndexOutOfBoundsException caught."); System.out.println("Message: " + e.getMessage()); System.out.println("Stack Trace: "); e.printStackTrace(); System.out.println("Cause: " + e.getCause()); }catch (Exception e) { System.out.println("NullPointerException caught."); System.out.println("Message: " + e.getMessage()); System.out.println("Stack Trace: "); e.printStackTrace(); System.out.println("Cause: " + e.getCause()); } } }
输出
ArithmeticException caught. Message: null Stack Trace: java.lang.ArithmeticException at Main.main(Main.java:7) Cause: null
因此,在本文中,我们讨论了处理Java编程语言中异常方法的不同方法。
The above is the detailed content of Java program that handles exception methods. For more information, please follow other related articles on the PHP Chinese website!
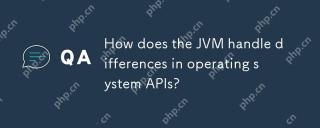
JVM handles operating system API differences through JavaNativeInterface (JNI) and Java standard library: 1. JNI allows Java code to call local code and directly interact with the operating system API. 2. The Java standard library provides a unified API, which is internally mapped to different operating system APIs to ensure that the code runs across platforms.
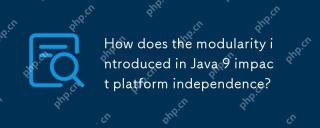
modularitydoesnotdirectlyaffectJava'splatformindependence.Java'splatformindependenceismaintainedbytheJVM,butmodularityinfluencesapplicationstructureandmanagement,indirectlyimpactingplatformindependence.1)Deploymentanddistributionbecomemoreefficientwi
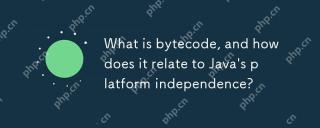
BytecodeinJavaistheintermediaterepresentationthatenablesplatformindependence.1)Javacodeiscompiledintobytecodestoredin.classfiles.2)TheJVMinterpretsorcompilesthisbytecodeintomachinecodeatruntime,allowingthesamebytecodetorunonanydevicewithaJVM,thusfulf
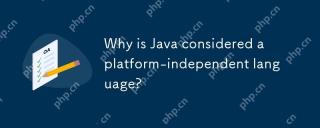
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),whichexecutesbytecodeonanydevicewithaJVM.1)Javacodeiscompiledintobytecode.2)TheJVMinterpretsandexecutesthisbytecodeintomachine-specificinstructions,allowingthesamecodetorunondifferentp
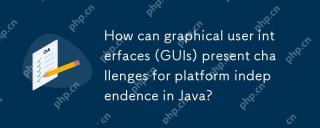
Platform independence in JavaGUI development faces challenges, but can be dealt with by using Swing, JavaFX, unifying appearance, performance optimization, third-party libraries and cross-platform testing. JavaGUI development relies on AWT and Swing, which aims to provide cross-platform consistency, but the actual effect varies from operating system to operating system. Solutions include: 1) using Swing and JavaFX as GUI toolkits; 2) Unify the appearance through UIManager.setLookAndFeel(); 3) Optimize performance to suit different platforms; 4) using third-party libraries such as ApachePivot or SWT; 5) conduct cross-platform testing to ensure consistency.
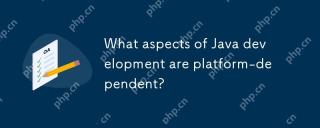
Javadevelopmentisnotentirelyplatform-independentduetoseveralfactors.1)JVMvariationsaffectperformanceandbehavioracrossdifferentOS.2)NativelibrariesviaJNIintroduceplatform-specificissues.3)Filepathsandsystempropertiesdifferbetweenplatforms.4)GUIapplica
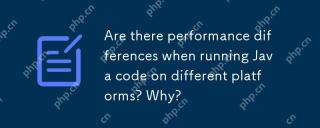
Java code will have performance differences when running on different platforms. 1) The implementation and optimization strategies of JVM are different, such as OracleJDK and OpenJDK. 2) The characteristics of the operating system, such as memory management and thread scheduling, will also affect performance. 3) Performance can be improved by selecting the appropriate JVM, adjusting JVM parameters and code optimization.
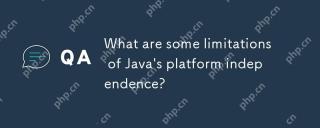
Java'splatformindependencehaslimitationsincludingperformanceoverhead,versioncompatibilityissues,challengeswithnativelibraryintegration,platform-specificfeatures,andJVMinstallation/maintenance.Thesefactorscomplicatethe"writeonce,runanywhere"


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
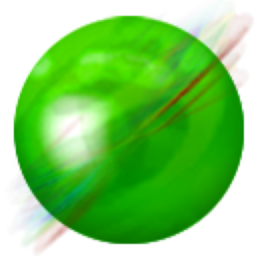
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
