How to use PHP message queue to develop real-time chat function
With the rapid development of the Internet, real-time communication has become an increasingly important application requirement. In web applications, it is a very common requirement to implement real-time chat function, and using PHP message queue to develop real-time chat function can easily implement asynchronous processing and improve the performance and scalability of the system. This article will introduce in detail how to use PHP message queue to develop real-time chat function.
1. Understand the basic concepts of message queue
Message queue is a first-in-first-out (FIFO) data structure used to solve the problem of asynchronization between systems. In the real-time chat function, the message queue can act as the middleware for message delivery, sending messages to subscribers to achieve real-time message communication.
2. Choose the appropriate message queue system
Currently, there are many message queue systems on the market to choose from, such as RabbitMQ, Apache Kafka, ActiveMQ, etc. When selecting a message queue system, factors such as system performance, reliability, applicable scenarios, and scalability should be considered. In this article, we take RabbitMQ as an example to introduce how to use PHP message queue to develop real-time chat function.
3. Install and configure RabbitMQ
Before you start using RabbitMQ, you need to install and configure it first. For specific installation and configuration steps, please refer to the official documentation of RabbitMQ.
4. Create producers and consumers of PHP message queue
In PHP, you can use the PHP-amqplib library to operate RabbitMQ. First, you need to create a producer and consumer of the message queue. The producer is responsible for sending messages to the message queue, and the consumer is responsible for obtaining messages from the message queue and processing them. The following is a simple sample code:
Producer code:
<?php require_once __DIR__.'/vendor/autoload.php'; use PhpAmqpLibConnectionAMQPStreamConnection; use PhpAmqpLibMessageAMQPMessage; $connection = new AMQPStreamConnection('localhost', 5672, 'guest', 'guest'); $channel = $connection->channel(); $channel->queue_declare('chat_queue', false, false, false, false); $message = new AMQPMessage('Hello World!'); $channel->basic_publish($message, '', 'chat_queue'); echo "Message sent to chat_queue "; $channel->close(); $connection->close();
Consumer code:
<?php require_once __DIR__.'/vendor/autoload.php'; use PhpAmqpLibConnectionAMQPStreamConnection; $connection = new AMQPStreamConnection('localhost', 5672, 'guest', 'guest'); $channel = $connection->channel(); $channel->queue_declare('chat_queue', false, false, false, false); echo 'Waiting for messages. To exit press CTRL+C '; $callback = function ($msg) { echo "Received message: " . $msg->body . " "; }; $channel->basic_consume('chat_queue', '', false, true, false, false, $callback); while ($channel->is_consuming()) { $channel->wait(); } $channel->close(); $connection->close();
5. Implement real-time chat function
Based on the above producer and consumer code that enables real-time chat functionality. On the chat interface, when the user sends a message, the message is sent to the producer through an AJAX request, and the producer sends the message to the message queue; at the same time, the consumer monitors the message queue in real time, and once a new message arrives, the callback function is triggered. for processing. The following is a simple sample code:
// 聊天界面的HTML代码 <div id="chat_box"></div> <input type="text" id="chat_input" placeholder="请输入消息"> <button id="send_button">发送</button> // JavaScript代码 <script> var chatInput = document.getElementById('chat_input'); var sendButton = document.getElementById('send_button'); var chatBox = document.getElementById('chat_box'); sendButton.addEventListener('click', function () { var message = chatInput.value; chatInput.value = ''; // 发送消息到生产者 var xhr = new XMLHttpRequest(); xhr.open('POST', 'send_message.php'); xhr.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded'); xhr.send('message=' + encodeURIComponent(message)); }); // 定时从服务器获取消息 setInterval(function () { var xhr = new XMLHttpRequest(); xhr.open('GET', 'get_messages.php'); xhr.onreadystatechange = function () { if (xhr.readyState === XMLHttpRequest.DONE && xhr.status === 200) { var messages = JSON.parse(xhr.responseText); chatBox.innerHTML = ''; for (var i = 0; i < messages.length; i++) { var div = document.createElement('div'); div.innerText = messages[i]; chatBox.appendChild(div); } } }; xhr.send(); }, 1000); </script> // send_message.php代码 <?php require_once __DIR__.'/vendor/autoload.php'; use PhpAmqpLibConnectionAMQPStreamConnection; use PhpAmqpLibMessageAMQPMessage; $connection = new AMQPStreamConnection('localhost', 5672, 'guest', 'guest'); $channel = $connection->channel(); $channel->queue_declare('chat_queue', false, false, false, false); $message = $_POST['message']; $message = new AMQPMessage($message); $channel->basic_publish($message, '', 'chat_queue'); $channel->close(); $connection->close(); // get_messages.php代码 <?php require_once __DIR__.'/vendor/autoload.php'; use PhpAmqpLibConnectionAMQPStreamConnection; use PhpAmqpLibMessageAMQPMessage; $connection = new AMQPStreamConnection('localhost', 5672, 'guest', 'guest'); $channel = $connection->channel(); $channel->queue_declare('chat_queue', false, false, false, false); $messages = []; $callback = function ($msg) use (&$messages) { $messages[] = $msg->body; }; $channel->basic_consume('chat_queue', '', false, true, false, false, $callback); while ($channel->is_consuming()) { $channel->wait(); } $channel->close(); $connection->close(); echo json_encode($messages);
6. Summary
Using PHP message queue to develop real-time chat function can greatly improve the performance and scalability of the system. Through RabbitMQ, we can easily implement asynchronous processing, send messages to subscribers, and achieve real-time message communication. Through the above sample code, you can simply implement a real-time chat function based on PHP message queue. Of course, in practical applications, it is also necessary to consider the implementation of functions such as message persistence, message subscription and push, which requires in-depth development based on specific needs. I hope this article can be helpful to develop real-time chat function using PHP message queue.
The above is the detailed content of How to use PHP message queue to develop real-time chat function. For more information, please follow other related articles on the PHP Chinese website!
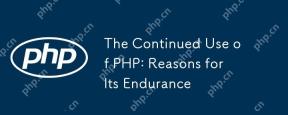
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
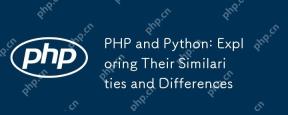
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
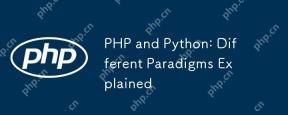
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
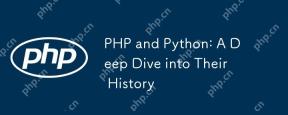
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
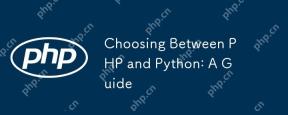
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
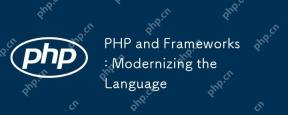
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
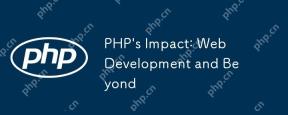
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
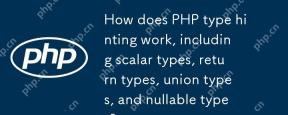
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
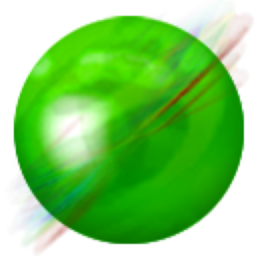
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment