In ES5, we use the concat method to copy an array. Additionally, some developers use the slice() method, which slices all elements of the referenced array and creates a new array by passing 0 as argument.
Example
Users can follow the example below to clone an array using the slice() method. We have created array1 which contains values of different data types. After that, we copy array1 using slice() method and store it in "clone" variable.
<html> <body> <h2 id="Using-the-i-slice-i-method-to-clone-the-array-in-JavaScript">Using the <i>slice()</i> method to clone the array in JavaScript</h2> <div id = "output"> </div> <script> let output = document.getElementById('output'); let array1 = [10, "hello", 30, true]; output.innerHTML += "The original array: " + array1 + " <br>"; let clone = array1.slice(0); output.innerHTML += "The cloned array: " + clone + " <br>"; </script> </body> </html>
Users have already seen how we can clone arrays in ES5.
Additionally, users can consider copying arrays just like ordinary variables such as strings, numbers, and Boolean values using the assignment operator.
Users may encounter problems when copying arrays using assignment operators. Let us understand it through the following example.
Copy array using assignment operator
In the example below, the string array contains various strings. We have assigned the strings array to the strings2 array. After that, we push the new string value into the strings2 array.
Example
<html> <body> <h2 id="Using-the-i-assignment-i-operator-to-clone-the-array-in-JavaScript">Using the <i>assignment</i> operator to clone the array in JavaScript</h2> <div id = "output"> </div> <script> let output = document.getElementById('output'); let array1 = ["Hi", " users", "Welcome"]; let array2 = array1; array2.push("New value"); output.innerHTML += "The value of array2: " + array2 + " <br>"; output.innerHTML += "The value of array1: " + array1 + " <br>"; </script> </body> </html>
In the above output, the user can observe that when we push the string value to the strings2 array, it is also pushed to the strings array. Why does this happen?
Here, the concepts of mutable and immutable objects come into play.
Mutable objects and immutable objects
In JavaScript, arrays and objects are mutable, which means we can change their values after initializing them after creating them. Therefore, the strings2 array does not exist in the above example. When we assign the strings array to the strings2 array, it generates a reference to the strings array. So whenever we change the strings2 array, it also changes the strings array.
Therefore, we need to create an actual copy of the array without referencing the other array
Now, let’s learn how to clone an array in ES6.
Clone an array using the spread operator (...) in ES6
The syntax of the expansion operator is three dots (...). We can use this to spread iterable objects like arrays. The spread operator creates a new copy of an array or object.
grammar
Users can copy arrays using the spread operator according to the following syntax.
let booleansCopy = [...booleans];
Example
In the example below, we have created a boolean array containing different boolean values. After that, we create a copy of the boolean array using the spread operator and assign the copy to the booleanCopy variable.
In the output, the user can observe that the booleanCopy array contains the same values as the boolean array contains.
<html> <body> <h2 id="Using-the-i-spread-operator-i-to-clone-the-array-in-JavaScript">Using the <i> spread operator </i> to clone the array in JavaScript</h2> <div id = "output"> </div> <script> let output = document.getElementById('output'); let booleans = [true, false, false, true, true]; let booleansCopy = [...booleans]; output.innerHTML += "The values of the booleansCopy array: " + booleansCopy + "<br>"; </script> </body> </html>
Example
In the example below, the dimensions array contains different numerical values. We created a copy of the size array using the spread operator and stored the array in the sizedClone variable using the assignment operator.
Afterwards, we push 60 into the sizeClone array.
<html> <body> <h2 id="Using-the-i-spread-i-operator-to-clone-the-array-in-JavaScript">Using the <i>spread</i> operator to clone the array in JavaScript</h2> <div id = "output"> </div> <script> let output = document.getElementById('output'); let sizes = [34, 43, 45, 47, 49, 50]; output.innerHTML += "-------Before Push-------" + "<br>"; output.innerHTML += "The values of the sizes array: " + sizes + "<br>"; let sizesClone = [...sizes]; output.innerHTML += "The values of the sizesClone array: " + sizesClone + "<br>"; sizesClone.push(60); output.innerHTML += "------After Push------" + "<br>"; output.innerHTML += "The values of the sizes array: " + sizes + "<br>"; output.innerHTML += "The values of the sizesClone array:" + sizesClone + "<br>"; </script> </body> </html>
In the above output, the user can observe that 60 is reflected in the sizesClone array but not in the Sizes array because we create an actual copy of the array instead like in example 2 Reference array.
Now, users clearly understand why assignment operators are not used in ES6 and why spread operators are used to clone arrays.
The above is the detailed content of How to clone an array in ES6?. For more information, please follow other related articles on the PHP Chinese website!
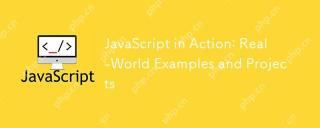
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
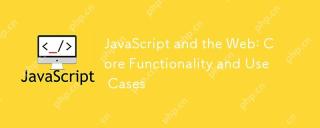
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
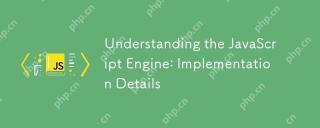
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
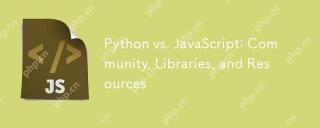
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
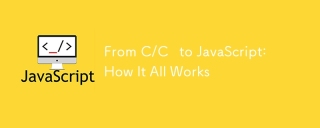
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
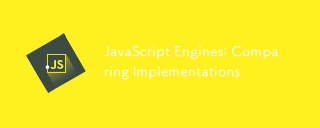
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
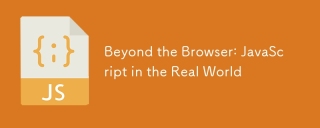
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Atom editor mac version download
The most popular open source editor
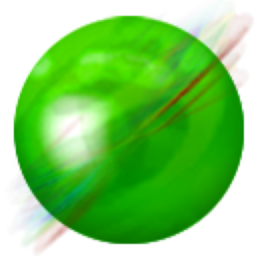
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment